Hello, i have this issue with probably my code where my animation of my model is not playing when its moving. Do you guys know how to fix this?
here is my code: (please note that i dont have experience with activating animations with scripts)
local animation = script:WaitForChild("Animation")
local npc = script.Parent:WaitForChild("Furnite")
local model = npc:LoadAnimation(animation)
model:Play()
Here is also a picture of where my stuff is parented and childed to.
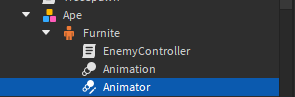
Also no errors. My NPC is just standing in its normal position when it was builded.
Do you guys know how to fix this?
1 Like
It looks like you’re calling :WaitForChild("Animation")
on your script
but the Animation is actually in the NPC (Humanoid). Additionally, “Furnite” is your script’s parent, so you would never resolve either of these two statements.
Try this:
local npc = script.Parent
local animation = npc:WaitForChild("Animation")
local model = npc:LoadAnimation(animation)
model:Play()
i get a infinite yield possible error
I guess I should have asked, is this script the EnemyController
script shown in the screenshot?
No, I’ll show you the script of what i wrote there.
local RunService = game:GetService("RunService")
local Players = game:GetService("Players")
local furnite = script.Parent
local root = furnite.Parent.PrimaryPart
local targetDistance = script:GetAttribute("TargetDistance")
local stopDistance = script:GetAttribute("StopDistance")
local damage = script:GetAttribute("Damage")
local attackDistance = script:GetAttribute("AttackDistance")
local attackWait = script:GetAttribute("AttackWait")
local lastAttack = tick()
function findNearestPlayer()
local playerList = Players:GetPlayers()
local nearestPlayer = nil
local distance = nil
local direction = nil
for _, player in pairs(playerList) do
local character = player.Character
if character then
local distanceVector = (player.Character.HumanoidRootPart.Position - root.Position)
if not nearestPlayer then
nearestPlayer = player
distance = distanceVector.Magnitude
direction = distanceVector.Unit
elseif distanceVector.Magnitude < distance then
nearestPlayer = player
distance = distanceVector.Magnitude
direction = distanceVector.Unit
end
end
end
return nearestPlayer, distance, direction
end
RunService.Heartbeat:Connect(function()
local nearestPlayer, distance, direction = findNearestPlayer()
if nearestPlayer then
if distance <= targetDistance and distance >= stopDistance then
furnite:Move(direction)
else
furnite:Move(Vector3.new())
end
if distance <= attackDistance and tick() - lastAttack >= attackWait then
lastAttack = tick()
nearestPlayer.Character.Humanoid.Health -= damage
end
end
end)
No need, just need to know where this script is relative to the other stuff in your screenshot.
I think it might be because your loading an animation into a character model, but the animation is not playing when the model is moving.
Try these…
Check if the animation is enabled
Check if the animation is set to loop
Check if the animation is being overwritten
Check if the animation is correctly bound to the model
If those dont help here’s an updated version of your code that includes some error handling to help you troubleshoot the issue:
local animation = script:WaitForChild("Animation")
local npc = script.Parent:WaitForChild("Furnite")
local model = npc:LoadAnimation(animation)
if model == nil then
warn("Failed to load animation")
return
end
if model.IsPlaying == true then
model:Stop()
end
model.Looped = true
model.Priority = Enum.AnimationPriority.Action
model:Play()
Infinite yield possible on 'Workspace.Ape.AnimatePLayer:WaitForChild(“Animation”)
its relitive to also the humanoid/ “furnite.”
Can you show a screenshot with all of that stuff and the script that you’re working on?
dumb me, i forgot to put the animation player in the humanoid.
I think its cause there’s a typo in the first line I didn’t realise replace the first line with
local animation = script.Parent:WaitForChild("Animation")
Infinite yield possible on 'Workspace.Ape:WaitForChild(“Animation”) i get and here:
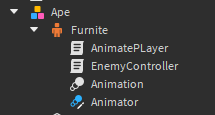
So AnimatePlayer
is the script we’re working on correct?
Also can you post your code again? Something isn’t right.
1 Like
still got a infinite yield possible message
indeed AnimatePlayer Is the script were working on.
local animation = script:WaitForChild(“Animation”)
local npc = script.Parent:WaitForChild(“Furnite”)
local model = npc:LoadAnimation(animation)
model:Play()
Can you try the code I posted earlier?
It’s Inifinitly looping the animation while not moving, I think i forgot to add that i want it to play the animation whenever its chasing somebody or just moving in general.