These arenât terribly hard, itâs similar to just normal slide bars but you multiply the 0-1 value by 255.
Unless youâre uncertain on how to arrange sliders in the first place, Iâm unsure how to describe how to start. So Iâll just explain everything as what I do, just minus the code as per requested. This is kind of a word bomb tbh and added lots of unnecessary content into this post.
To create a slider is fairly easy, personally I just make a small frame where the Y size will be like 1-2 offset value and X is scaled. I then add 3 child frames for visuals. Child one would be the min (Far left), child two (Far right) would be the max and lastly the slide bar itself.
kind of like this.
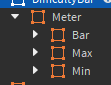
While you donât need min-max; I personally like them for the convenience of finding the min position and max position without the need of subtracting by half the absolute size of the main frame.
And finding the values 0 - 1 with this is pretty easy, check the mouseâs X coordinates subtract the min position value then divide that by the total distance (max - min). Then to set the sliderâs position, thereâs 2 ways of doing this but Iâll just go with the simple method, since you have the percent at which you dragged at, set the x scale factor to the percent.
For the code, I personally turn a slider into an object and have an OnUpdate
bind to let listening scripts know that the slider is being moved, but itâs not completely necessary. And to save some trouble, using Frame.MouseMoved:Connect(function(x, y)
is convenient for the fact it will only fire when the mouse is inside of the frame; this is why I have a parent background frame for the slider. Just need to be completely sure that when using InputBegan
and InputEnded
youâre listening for the correct input type, like mouse or touched (Was a dumb mistake on my part).
Now the numbers, if you want a method for players to type in the values you want, you can just as easily do that with a little bit of string formatting, but I wonât get too much into that. After taking the RGB value from text, just map it to 0-1 and update the slider with the new position and number.
And for text legibility, doing .Text = ("%.0f"):format(percent * 255)
is convenient and clean. The number 0 in %.0f
is the decimal place if you want to get specific.