- I want to make bullet look at mouse cursor and move to mouse cursor direciton
Explorer Is this one.
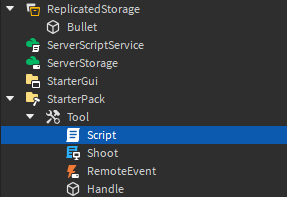
Also, script is
Local Script,
local Players = game:GetService("Players")
local player = Players.LocalPlayer
local mouse = player:GetMouse()
script.Parent.Activated:Connect(function()
script.Parent.RemoteEvent:FireServer(mouse)
end)
Script
script.Parent.RemoteEvent.OnServerEvent:Connect(function(Player,Mouse)
local bullet = game.ReplicatedStorage.Bullet:Clone()
--Move bullet To handle position and move to mouse cursor direction
end)```
1 Like
You need to do this:
local Players = game:GetService("Players")
local player = Players.LocalPlayer
local mouse = player:GetMouse()
script.Parent.Activated:Connect(function()
mouse = player:GetMouse()
script.Parent.RemoteEvent:FireServer(mouse)
end)
No, you can’t send the player’s mouse over to the server. It would end up as nil,
Actually yeah I was being a bit dumb. I don’t remember how to get the cursor position in 3D space though. But pretty much send a Vector3 and use CFrame.new’s 2nd argument to point to that Vector3.
You can’t send over the mouse to the server. Instead send over mouse.Hit
which is a CFrame, in which over on the server you can choose to tween, it, use bodyVelo, lookVector, there are too many ways to move the bullet, it depends on which way you want to do it. You would use CFrame.lookAt
or multiply the bullet’s CFrame like so bullet.CFrame = bullet.CFrame * CFrame.lookAt(bullet.Position, mousePosition)
.
1 Like
Contrary to other responses here: mouse
is a deprecated class. If possible, you should be using UserInputService
.
Depending on your threat model, decide whether to raycast on the client or server. I prefer the client because it’s less resource intensive. Ergo:
LocalScript
:
-- FETCH THE 2D POSITION OF THE PLAYER'S MOUSE ON THEIR SCREEN
local mouse: Vector2 = UIS:GetMouseLocation()
-- FETCH THE CAMERA FOR THE PLAYER
local camera: Camera = game.Workspace.CurrentCamera
-- ACCOUNTING FOR GUI INSET, RAYCAST FROM THE PLAYER'S CAMERA
-- TO A 3D POSITION IN THE WORKSPACE, AT A DEPTH OF 100
local ray: Ray = camera:ScreenPointToRay(mouse.X, mouse.Y, 100)
-- PASS THE Origin TO THE SERVER, WHICH IS THE 3D POSITION IN THE WORKSPACE
event:FireServer(ray.Origin)
Then, in a Script
on the server:
function main(plyr: Player, origin: Vector3)
local DS: Debris = game:GetService("Debris")
-- POSITION A
local character_primary: Part = character.PrimaryPart
local distance: number = (character_primary.Position - origin).Magnitude
local speed: number = distance / 20
local bullet: Part = Instance.new("Part")
bullet.Position = character_primary.Position + character_primary.CFrame.LookVector * 5
bullet.Anchored = true
bullet.Parent = game.Workspace
bullet.Touched:Connect(function()
-- CREATE TOUCHINTEREST EVENT FROM CONNECTED FUNCTION
end)
-- CREATE A TWEEN MOVING THE BULLET FROM THE PLAYER TO TO DESTINATION
local movement: Tween = TS:Create(bullet, TweenInfo.new(speed), {Position = origin})
movement:Play()
-- REMOVE THE BULLET AFTER 5 SECONDS
DS:AddItem(bullet, 5)
-- ASYNCHRONOUSLY UPDATE BULLET
coroutine.wrap(function()
local debounce: boolean = false
-- WHILE THE BULLET EXISTS
while bullet do
-- GET THE PARTS IN CONTACT, ITERATE THROUGH THEM
local parts = bullet:GetTouchingParts()
for k, v: Part in pairs(parts) do
-- DO SOMETHING, THEN DESTROY ON HIT
task.wait(1)
bullet:Destroy()
end
task.wait()
end
end)()
end
event.OnServerEvent:Connect(main)
Though, I appreciate this isn’t the most readable. mouse
should work fine for now, unless ROBLOX removes support for it, so I suggest keeping up with non-deprecated classes.
1 Like