shlexr
(jenson)
#1
Hi there, I’m trying to keep the table when outputted into an UI list in order of how it was when I put it in the modulescript.
In the modulescript:
ItemPicker = {
Cups = {
Small = {
Name = "Cup",
ToolTip = "Small",
},
Medium = {
Name = "Cup",
ToolTip = "Medium",
},
Large = {
Name = "Cup",
ToolTip = "Large",
}
},
},
In game:
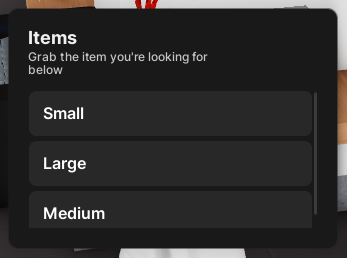
code that turns it into ui:
-- ItemList = Cups table from above
for _, Item in pairs(ItemList) do
local NewItem = ItemPicker.List.Template:Clone()
NewItem.Parent = ItemPicker.List
NewItem.Name = Item.Name
NewItem.Title.Text = Item.ToolTip or Item.Name
NewItem.Visible = true
NewItem.Interact.MouseButton1Click:Connect(function()
-- req item
end)
end
If anyone could help, it’d be much appreciated.
4Avern
(Avern Hendrix)
#2
In the modulescript, change the Cups
table to:
ItemPicker = {
Cups = {
{
Key = "Small",
Name = "Cup",
ToolTip = "Small",
},
{
Key = "Medium",
Name = "Cup",
ToolTip = "Medium",
},
{
Key = "Large",
Name = "Cup",
ToolTip = "Large",
},
},
}
In your game code, change the loop to use ipairs
instead of pairs
:
-- ItemList = Cups table from above
for _, Item in ipairs(ItemList) do
local NewItem = ItemPicker.List.Template:Clone()
NewItem.Parent = ItemPicker.List
NewItem.Name = Item.Name
NewItem.Title.Text = Item.ToolTip or Item.Name
NewItem.Visible = true
NewItem.Interact.MouseButton1Click:Connect(function()
-- req item
end)
end
Now your table is ordered, and using ipairs
instead of pairs
will preserve the order when iterating through the items in the table.
1 Like
shlexr
(jenson)
#3
Worked perfectly thank you. The key wasn’t necessary so I just removed that entirely.
1 Like
system
(system)
Closed
#4
This topic was automatically closed 14 days after the last reply. New replies are no longer allowed.