I understand this. However, this is how i have my system so far set up… and since your last message i have made progress. Understand, all of this works up to the point of snapping into the frame i want.
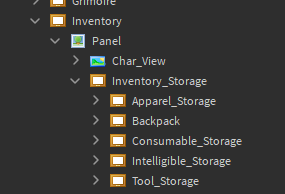
The backpack is the side tool bar setting, to bind certain items to the toolbar.
Within all of the item types is this:
so when the items refresher goes off, it detects all of the slots to find the first available one, and then adds the item to that slot… this has been working. I also have the filled slots as a physical value to help manage between the drag script, which i have been using as a basic local script within the image button itself because in the module(which is where i’d like to do it) it wasnt working right.
When you drag the item… which i have been doing with user input to activate, I am also using .MouseEntered and Leave to detect the hovered over frame, and if a frame is hovered over from any of the container frames, it should snap to the thing before you let go. This is working… except for the fact that its buggy in that MouseEntered inst registering these slots so close together, because to save time i looped through each folder to detect the frames and added the event through there, which works… but not consistently.
The image button is of a size of Udmi2.new(1,0,1,0), so its nice and easy to get into the frame and fit nicely to whatever the frames size it. And inorder to be nice and orderly i am using a UIGridlayout for the main item containers, and a UiListLayout for the Backpack. And its nice and pretty that way. All of this is working, but i’d like an alternative to mouseEntered.
local UserInputService = game:GetService("UserInputService")
local player = game.Players.LocalPlayer
local Mouse = player:GetMouse()
local gui = script.Parent
local PlayerGui = player:WaitForChild("PlayerGui")
local Inventory_Window = PlayerGui:WaitForChild("Bar"):WaitForChild("Inventory")
local Inventory_Panel = Inventory_Window:WaitForChild("Panel")
local Inventory_Storage = Inventory_Panel:WaitForChild("Inventory_Storage")
local Backpack = Inventory_Storage:WaitForChild("Backpack")
local Item_Select = Inventory_Panel:WaitForChild("Item_Select")
local Apparel_Button = Inventory_Panel:WaitForChild("Apparel_Button")
local Apparel_Storage = Inventory_Storage:WaitForChild("Apparel_Storage")
local Tools_Button = Inventory_Panel:WaitForChild("Tools_Button")
local Tools_Storage = Inventory_Storage:WaitForChild("Tool_Storage")
local Consumables_Button = Inventory_Panel:WaitForChild("Consumables_Button")
local Consumables_Storage = Inventory_Storage:WaitForChild("Consumable_Storage")
local Intelligibles_Button = Inventory_Panel:WaitForChild("Intelligibles_Button")
local Intelligibles_Storage = Inventory_Storage:WaitForChild("Intelligible_Storage")
local Inventory_Folder = player:WaitForChild("Player_Inventory")
local Apparel_Folder = Inventory_Folder:WaitForChild("Apparel")
local Tools_Folder = Inventory_Folder:WaitForChild("Tool")
local Consumables_Folder = Inventory_Folder:WaitForChild("Consumable")
local Intelligibles_Folder = Inventory_Folder:WaitForChild("Intelligible")
local Item_In_Question = Inventory_Folder:WaitForChild(gui.Item_Type.Value):WaitForChild(gui.Name)
local dragging
local Hover
local dragInput
local dragStart
local startPos
local startParent
spawn(function()
coroutine.resume(coroutine.create(function()
for i,v in pairs(Backpack:GetChildren()) do
if v:IsA'Frame' then
v.MouseEnter:Connect(function()
Hover = v
end)
v.MouseLeave:Connect(function()
Hover = nil
end)
end
end
end))
end)
spawn(function()
coroutine.resume(coroutine.create(function()
for i,v in pairs(Apparel_Storage:GetChildren()) do
if v:IsA'Frame' then
v.MouseEnter:Connect(function()
Hover = v
end)
v.MouseLeave:Connect(function()
Hover = nil
end)
end
end
end))
end)
spawn(function()
coroutine.resume(coroutine.create(function()
for i,v in pairs(Tools_Storage:GetChildren()) do
if v:IsA'Frame' then
v.MouseEnter:Connect(function()
Hover = v
end)
v.MouseLeave:Connect(function()
Hover = nil
end)
end
end
end))
end)
spawn(function()
coroutine.resume(coroutine.create(function()
for i,v in pairs(Consumables_Storage:GetChildren()) do
if v:IsA'Frame' then
v.MouseEnter:Connect(function()
Hover = v
end)
v.MouseLeave:Connect(function()
Hover = nil
end)
end
end
end))
end)
spawn(function()
coroutine.resume(coroutine.create(function()
for i,v in pairs(Intelligibles_Storage:GetChildren()) do
if v:IsA'Frame' then
v.MouseEnter:Connect(function()
Hover = v
end)
v.MouseLeave:Connect(function()
Hover = nil
end)
end
end
end))
end)
local function update(input)
print(Hover)
local delta = input.Position - dragStart
if Hover ~= nil and Hover.Parent == "Backpack"then
gui.Parent = Hover
gui.Position = UDim2.new(0,0,0,0)
elseif Hover ~= nil and Hover.Parent ~= "Backpack" then
gui.Parent = Hover
gui.Position = UDim2.new(0,0,0,0)
elseif Hover == nil then
gui.Parent = startParent
gui.Position = UDim2.new(startPos.X.Scale, startPos.X.Offset + delta.X, startPos.Y.Scale, startPos.Y.Offset + delta.Y)
end
end
gui.InputBegan:Connect(function(input)
if input.UserInputType == Enum.UserInputType.MouseButton1 or input.UserInputType == Enum.UserInputType.Touch then
dragging = true
dragStart = input.Position
startPos = gui.Position
startParent = gui.Parent
input.Changed:Connect(function()
if input.UserInputState == Enum.UserInputState.End then
dragging = false
if Hover ~= nil and Hover.Parent == "Backpack" and gui.Item_Type.Value == ("Consumable" or "Intelligible") then
gui.Position = UDim2.new(0,0,0,0)
Hover.Item.Visible = false
gui.Parent = Hover
elseif Hover ~= nil and Hover.Parent ~= "Backpack" then
gui.Position = UDim2.new(0,0,0,0)
gui.Parent = Hover
elseif Hover == nil then
gui.Position = UDim2.new(0,0,0,0)
gui.Parent = startParent
end
end
startParent = gui.Parent
end)
end
end)
gui.InputChanged:Connect(function(input)
if input.UserInputType == Enum.UserInputType.MouseMovement or input.UserInputType == Enum.UserInputType.Touch then
dragInput = input
end
end)
UserInputService.InputChanged:Connect(function(input)
if input == dragInput and dragging then
update(input)
end
end)