Well… in my framework I would do it like this:
A Core to store all the important stuff such as:
Compiler where all the framework functions are stored ( Doesn’t matter with autofill )
Config store all the Settings ( Doesn’t matter with autofill )
Types where all the types get stored and exported ( Required )
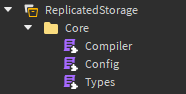
In my Client I would have:
A Loader which loads all the Scripts Children ( Doesn’t matter with autofill but needed to load the Scripts Children)
A Scripts Folder ( Currently a Module Script for autofill. Required ) which stored all the Scripts
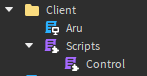
Here how the process work:
The Scripts Folder ( Module Script ) require the Compiler to get the framework functions or no and require the Types module
Then the Script Children will require it parent which will direct to the Scripts Folder where it return a table to autofill.
The code for the Scripts Folder
--Types
local Types = require(game:GetService("ReplicatedStorage").Shared.Core.Types)
--Game's Services
local ReplicatedStorage = game:GetService("ReplicatedStorage")
--Shared
local Shared = ReplicatedStorage:WaitForChild("Shared")
local Core = Shared:WaitForChild("Core")
--Compiler
local Compiler = require(Core["Compiler"])
local SharedFunctions = Compiler["Shared"]
local function New()
return setmetatable({}, {
__index = SharedFunctions -- You can either set __index or don't but must return a table with assigned type
}) :: Types.Client --Require for the Script to autofill framework functions
end
return New()
The code for the Types module
type Dictionary = { [string]: any }
type DefineDictionary<Type> = { [string]: Type }
export type Table = { [any]: any }
export type DefineTable<Value> = { [string]: Value }
export type Array = { [number]: any }
export type DefineArray<Type> = { [number]: Type }
export type Function = (...any) -> ...any
export type DefineFunction<Return> = (...any) -> Return
export type Shared = {
NewSharedClass: (self: any, ClassName: string, Recursive: boolean | any, ...any) -> Table,
GetSharedClass: (self: any, Name: string, Recursive: boolean) -> Table,
GetSharedModule: (self: any, Name: string, Recursive: boolean) -> Table,
GetEngine: (self: any, Name: string, Recursive: boolean) -> Table,
GetLibrary: (self: any, Name: string, Recursive: boolean) -> Table,
GetService: (self: any, Name: string, Recursive: boolean) -> Table,
GetPackage: (self: any, Name: string, Recursive: boolean) -> Instance | Table,
GetSharedAsset: (self: any, Name: string, Recursive: boolean) -> Instance | Table,
GetAllSharedAssets: (self: any) -> DefineArray<Instance>,
} & ExternalShared
export type Client = {
Setup: (self: any) -> never,
Heartbeat: (self: any, Time: number) -> never,
Stepped: (self: any, Time: number) -> never,
RenderStepped: (self: any, Time: number) -> never,
Start: (self: any) -> never,
NewClass: (self: any, ClassName: string, Recursive: boolean | any, ...any) -> Table,
GetClass: (self: any, Name: string, Recursive: boolean) -> Table,
GetModule: (self: any, Name: string, Recursive: boolean) -> Table,
GetGui: (self: any, Name: string, Recursive: boolean) -> GuiBase2d,
GetBackpack: (self: any, Name: string, Recursive: boolean) -> BackpackItem,
Warn: (self: any, any) -> never,
Classes: Folder,
Modules: Folder,
Scripts: Folder,
Player: Player,
Gui: PlayerGui,
Backpack: Backpack,
Character: Model,
Assets: Folder,
} & Shared & ExternalClient
type ExternalShared = {}
type ExternalClient = {}
return nil
Oh also I just know you can do this
export type Client = {
Scripts: typeof(Scripts Folder)
} & Shared & ExternalClient
You get every scripts children in the scripts folder