I am trying to display your roblox character with a tool in a viewport frame but when i clone the character and the tool, then parent said tool to the character it doesn’t automatically create the “Right Grip” weld that it normally does when equipping a tool. I also tried using the method Humanoid:EquipTool()
but that too wouldn’t create the weld. So I decided I would just copy the way roblox actually creates this “Right Grip” weld. I’ve never been the best at welding so I’m a bit stuck as the orientation of the tool is not how it should be.
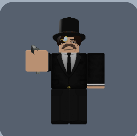
-viewport view
-in game view (right arm made transparent to show whole tool)
(there is also another problem of the tool somehow rendering inverted. which is a whole other can of worms)
Here is the code I wrote:
local RightGrip = Instance.new("Weld")
RightGrip.Name = "Right Grip"
RightGrip.Parent = CharacterClone:FindFirstChild("Right Arm")
RightGrip.Part0 = CharacterClone:FindFirstChild("Right Arm")
RightGrip.Part1 = ToolClone.Handle
RightGrip.C0 = CharacterClone:FindFirstChild("Right Arm").RightGripAttachment.CFrame
RightGrip.C1 = CFrame.fromMatrix(Vector3.zero, ToolClone.GripRight, ToolClone.GripUp, ToolClone.GripForward)
One problem is that the GripForward should be negated in the fromMatrix call—a positive Z direction is actually towards the “back” of a CFrame.
interesting, that somehow made the mesh render correctly and also makes the tool point up, however that means the orientation is still off.
There’s a Grip property of tools which encapsulates all of those other ones, might be useful/solve the problem:
RightGrip.C0 = ToolClone.Grip
-- leave the C1 unset
1 Like
I had no idea tools had a property called grip but I tried what you put and that left me with this.
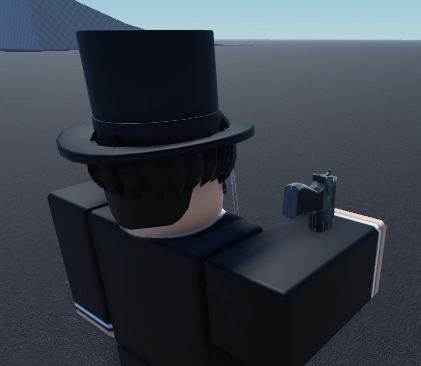
Did you leave the C1 unset?
To C1 = CFrame.new()
Did you also try to put the tool grip CFrame in C1 as well swapping it in case it doesn’t work?
If not then there is the new RigidConstraint instead of welds which is easier to visualize though functionally the same as a weld.
I decided to use the Rigid Constraint you were talking about and that worked very well
for anyone curious this is what I did:
local RigidConstraint = Instance.new("RigidConstraint")
RigidConstraint.Parent = CharacterClone:FindFirstChild("Right Arm")
RigidConstraint.Attachment0 = CharacterClone:FindFirstChild("Right Arm").RightGripAttachment
local Attachment = Instance.new("Attachment")
Attachment.CFrame = ToolClone.Grip * CFrame.Angles(math.rad(90),0,0) --This offset is applied by default for some reason
Attachment.Parent = ToolClone.Handle
RigidConstraint.Attachment1 = Attachment
I see the issue,
With welds replace attach0 with weld C0 vice versa for attach1 and C1
So
C1 = Attachment.CFrame
C0 = RightGripAttachment.CFrame
This should be the weld equivalent.
I also tried explaining this in my CFrame tutorial though I think it’s a bit too verbose.
I swear I tried that but I actually got that to work this time around.
so this is actually the true solution:
local RightGrip = Instance.new("Weld")
RightGrip.Name = "Right Grip"
RightGrip.Parent = CharacterClone:FindFirstChild("Right Arm")
RightGrip.Part0 = CharacterClone:FindFirstChild("Right Arm")
RightGrip.Part1 = ToolClone.Handle
RightGrip.C1 = ToolClone.Grip * CFrame.Angles(math.rad(90),0,0)
RightGrip.C0 = CharacterClone:FindFirstChild("Right Arm").RightGripAttachment.CFrame