As for keeping the player moving on one axis, one way you can do this is rebind the keys inside of PlayerModule
. You can find the script under your player’s PlayerScripts
folder.
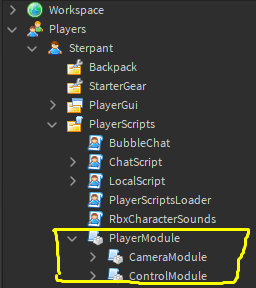
If you copy that into StarterPlayerScripts
it will override the default one.
You can change the keybindings inside of Keyboard
, which is under ControlModule
.
--[[
Here, I'm just commenting out the bindings that we don't need. I've then changed
the 'forward' action to move the character left, and
the 'backward' action to move
the character right.
]]
-- TODO: Revert to KeyCode bindings so that in the future the abstraction layer from actual keys to
-- movement direction is done in Lua
--ContextActionService:BindActionAtPriority("moveForwardAction", handleMoveForward, false,
-- self.CONTROL_ACTION_PRIORITY, Enum.PlayerActions.CharcterLeft)
--ContextActionService:BindActionAtPriority("moveBackwardAction", handleMoveBackward, false,
-- self.CONTROL_ACTION_PRIORITY, Enum.PlayerActions.CharcterRight)
ContextActionService:BindActionAtPriority("moveLeftAction", handleMoveLeft, false,
self.CONTROL_ACTION_PRIORITY, Enum.PlayerActions.CharacterBackward)
ContextActionService:BindActionAtPriority("moveRightAction", handleMoveRight, false,
self.CONTROL_ACTION_PRIORITY, Enum.PlayerActions.CharacterForward)
ContextActionService:BindActionAtPriority("jumpAction", handleJumpAction, false,
self.CONTROL_ACTION_PRIORITY, Enum.PlayerActions.CharacterJump)
Then, you can manipulate the camera to stay on one axis. Something quick to demonstrate how you might go about that:
local CAMERA_OFFSET = Vector3.new(20, 5, 0)
local RunService = game:GetService("RunService")
local player = game.Players.LocalPlayer
local character = player.Character or player.CharacterAdded:Wait()
local camera = workspace.CurrentCamera
while camera.CameraType ~= Enum.CameraType.Scriptable do
camera.CameraType = Enum.CameraType.Scriptable
end
local connection = RunService.RenderStepped:Connect(function()
camera.CFrame = CFrame.new(
character.HumanoidRootPart.Position + CAMERA_OFFSET, character.HumanoidRootPart.Position
)
return
end)
As for making the camera ‘smooth’, I would personally do that with springs. I made a post before about how you could do this, and there are also resources to help you: