For finding the world-oriented CFrame from a surface and normal from a raycast, if the surface was facing up and already world-oriented, how do I make its result have a lookvector facing (0,0,-1) instead of (-1,0,0)
Result:
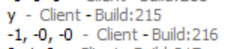
on a world-axis oriented baseplate (the slate floor)
Expected:

on a non world-axis oriented part (the plastic floor)
What I expect is that on all top-facing surfaces, the world-oriented CFrame from this function
local function GetWorldOrientedSurface(part, normalId)
local cf = part.CFrame
local rot = cf - cf.Position
local nObject = Vector3.fromNormalId(normalId)
local nWorld = rot * nObject
-- get orthogonal vector by utilizing the order of NormalId enums
-- i.e. Front.Value is 5 -> (5+1)%6 = 0 -> Right.Value
local xWorld = rot * Vector3.fromNormalId((normalId.Value + 1) % 6)
-- get other orthogonal vector
local zWorld = nWorld:Cross(xWorld)
-- make them both point "generally down"
if xWorld.Y > 0 then
print('gas')
xWorld = -xWorld
end
if zWorld.Y > 0 then
print('gass')
zWorld = -zWorld
end
-- choose the one pointing "more down" one as the z axis for the surface
if xWorld.Y < zWorld.Y then
print('gasss')
zWorld = xWorld
end
-- redefine x axis based on that
xWorld = nWorld:Cross(zWorld)
local surfaceRot = CFrame.fromMatrix(Vector3.new(), xWorld, nWorld, zWorld)
-- get width of part in direction of x and y
local sizeInWorldSpace = rot * part.Size
local sizeInSurfaceSpace = surfaceRot:Inverse() * sizeInWorldSpace
-- get position on surface
local surfaceCFrame = surfaceRot + cf.Position + nWorld * math.abs(sizeInSurfaceSpace.Y) / 2
return surfaceCFrame, Vector2.new(math.abs(sizeInSurfaceSpace.X), math.abs(sizeInSurfaceSpace.Z))
end
Should return a CFrame that has the lookvector of (0,0,-1), such that it always faces the negative Z direction.
IMPORTANT:
On the plastic platform, that’s what I want for the slate baseplate. The plastic platform is NOT rotated by any degree on its Y axis, and it is only rotated by its X axis by 90 degrees.
If it were rotated by any degree on its Y axis, the rotation of the part that follows my mouse would change as well.
The same goes for the slate baseplate. It’s NOT rotated AT ALL.