Sorry if this does not answer your original query, but I don’t quite get what you mean. Taking on @xuefei123’s suggestion:
ReserveServer is a method that allows you to create a special, reserved server of your game - sort of like a VIP server, but without a player having to buy it. These reserved servers don’t show up on your game page under “servers” and can only be joined by using TeleportToPrivateServer.
For example, imagine you have a party of four players, PlayersToTeleport
. All the elements of that table are Player objects. This means you could manually do:
local PlayersToTeleport = {
game.Players.Technoles,
game.Players.Builderman,
game.Players.RafDev,
game.Players.xuefei123
}
Ideally, you’d get this table/array/list of players through another system though, such as using the Occupant property of all Seats in a campfire - however, that is beside the scope of your question.
Now that you have your players, you need to reserve a server for them so they can play together. You can achieve this by using:
local code = game:GetService("TeleportService"):ReserveServer(game.PlaceId) -- you can use a different place ID if you want to take them to another place within the same universe
Note this method returns a special code that identifies the created server.
Finally, after you have reserved a server for your players, all you have to do is take them there. Do the following:
game:GetService("TeleportService"):TeleportToPrivateServer(game.PlaceId, code, PlayersToTeleport)
Please let me know if you don’t understand something I’ve said, I tried to explain the best I could.
EDIT: I’m providing a larger snippet on how you could make my above example work. Obviously, there are better ways to do this, but I think this is the easiest way to comprehend.
Assuming the following hierarchy:
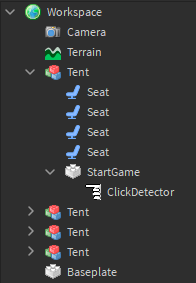
Putting the following script in ServerScriptService:
local PlaceId = 123456 -- the place ID for the actual game; must be in the same universe
if not game:IsLoaded() then
game.Loaded:Wait()
end
local WorkspaceChildren = workspace:GetChildren()
for key, value in pairs(WorkspaceChildren) -- goes through all children of workspace
if value:IsA("Model") and value.Name == "Tent" then
local AlreadyStarting = false
local ClickDetector = value.StartGame.ClickDetector
ClickDetector.MouseClick:Connect(function()
if AlreadyStarting then return end
AlreadyStarting = true
local PlayersToTeleport = {}
for _, Seat in pairs(value:GetChildren()) do
if Seat:IsA("Seat") and Seat.Occupant then
table.insert(PlayersToTeleport, Seat.Occupant)
end
end
local code = game:GetService("TeleportService"):ReserveServer(PlaceId)
game:GetService("TeleportService"):TeleportToPrivateServer(PlaceId, code, PlayersToTeleport)
end)
end
end