Im making a shop UI that displays items in a ScrollingFrame. The thing i want to achieve is making a grid with all the items being kept in a square frame. The problem is i dont know what constraint to use. I know theres UIGridLayout but i can seem to keep the frames in a square size on every resolution. I didnt use UIListLayout because it can only do one line. Basically, I want it so if i insert a square frame inside the scrolling frame, it will automatically position itself to the next open space.
This is what i want to achieve:
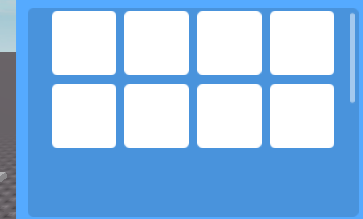
Help / Advice would be highly appreciated. Thank you 
You have three options:
- You can use a UIAspectRatioConstraint inside the UIGridLayout object
- You can manually keep the aspect ratio of CellSize with a script using AbsoluteSize and setting the CellSize
- You can make a custom grid system (e.g. using horizontal container frames in a vertical UIListLayout)
The first is the best option, it works charmingly.
If you wanted to the second, here’s a guide. Every time the Size of the ScrollingFrame changes, do this:
local PER_ROW = 4 -- you can of course use the UIGridLayout property for this
-- so scale of each item = 1/4 = 0.25
-- but we need to take padding into consideration so...
local totalPadding = gridLayout.CellPadding.X.Scale * (PER_ROW - 1)
local actualScale = (1 - totalPadding) / PER_ROW
-- convert from scale into pixels so we can guarantee will always be square
local sizeX = scrollingFrame.AbsoluteSize.X * actualScale
gridLayout.CellSize = UDim2.new(0, sizeX, 0, sizeX)
Also tip for grids in a ScrollingFrame: Every time the number of items in the grid change, update the CanvasSize of the ScrollingFrame to the size of UIGridLayout:
ScrollingFrame.CanvasSize = UDim2.new(0, 0, 0, UIGridLayout.AbsoluteContentSize.Y)
5 Likes
It works as intended! Thank you!
1 Like