You are correct in thinking that you need to use the tween service for this, although you could interpolate as the answers above are suggesting, tweening will make it look smoother and you will have more control over your final product.
To use the tween service you need to retreve it using the :GetService
method from game, looking like this:
game:GetService("TweenService")
Next we need to tell the tween we are going to create what value to change and what to change it to, this is done via a table. For simplicity sake we will call it goal but you can call it any other valid variable name.
local Goal = {}
Goal.Transparency = 0 -- Goal.ValueToChange = What to change to
Now we need to tell the tween how we want the value to change - fast, slow, bouncy etc. This is done by creating a TweenInfo
value like this:
local tweenInfo = TweenInfo.new(time, easingStyle, easingDirection, repeatCount, reverse, delay)
If you reach an argument and don’t want to change anymore, you don’t have to put in all of the arguments. Don’t worry if you can’t remember all of the arguments, they can all be found here.
So filled out for your example you may want:
local tweenInfo = TweenInfo.new(1, Enum.EasingStyle.Linear, Enum.EasingDirection.Out)
Easing Style Cheat Sheet
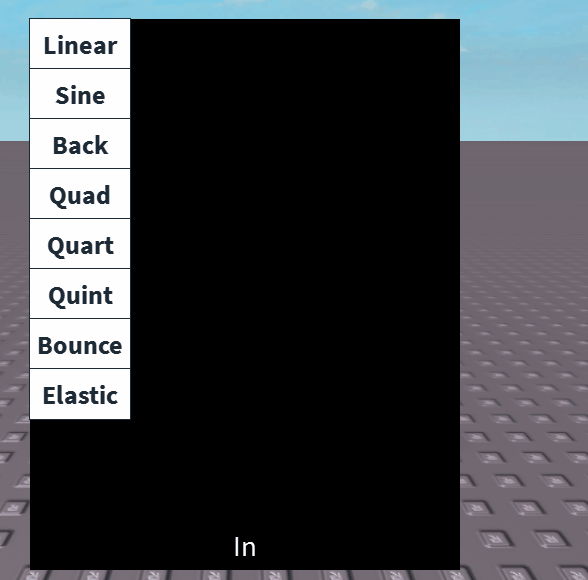
Now all we need to do is create the tween and play it. We can do this by using the :Create
method from tween service with the arguments we just made.
local GuiFrame = -- Wherever your frame is
local tween = TweenService:Create(GuiFrame, tweenInfo, goal)
tween:Play() -- Play the tween
So all together your code should look something like the following:
local GuiFrame = -- Wherever your frame is
local Goal = {}
Goal.Transparency = 0 -- Goal.ValueToChange = What to change to
local tweenInfo = TweenInfo.new(1, Enum.EasingStyle.Linear, Enum.EasingDirection.Out)
local tween = TweenService:Create(GuiFrame, tweenInfo, goal)
tween:Play() -- Play the tween