ate9d
(ate9d)
#1
Hello! I was wondering if anyone knew how to limit a character’s arms from moving up too far or too far down shown below.
My code snippet:
RunService.RenderStepped:connect(function(DeltaTime)
if ToolEquipped then
if Mouse.Hit.Position ~= nil then
local RightX, RightY, RightZ = RightShoulder.C0:ToEulerAnglesYXZ()
local LeftX, LeftY, LeftZ = LeftShoulder.C0:ToEulerAnglesYXZ()
TweenService:Create(RightShoulder, TweenInfo.new(0.125), {C0 = (RightShoulder.C0 * CFrame.Angles(0,0, -RightZ)) * CFrame.Angles(0,0, math.asin((Mouse.Hit.Position - Mouse.Origin.Position).Unit.Y))}):Play()
TweenService:Create(LeftShoulder, TweenInfo.new(0.125), {C0 = (LeftShoulder.C0 * CFrame.Angles(0,0, -LeftZ)) * CFrame.Angles(0,0, math.asin((-Mouse.Hit.Position - -Mouse.Origin.Position).Unit.Y))}):Play()
end
end
end)
If anyone has done this before please let me know how you were able to achieve it!
1 Like
Ax1sAng1e
(Pastor James)
#2
You would use math.clamp for this, you might have to experiment with the min and max values as i don’t know what those would be.
local minimumAngle = -10
local maximumAngle = 10
RunService.RenderStepped:connect(function(DeltaTime)
if ToolEquipped then
if Mouse.Hit.Position ~= nil then
local RightX, RightY, RightZ = RightShoulder.C0:ToEulerAnglesYXZ()
local LeftX, LeftY, LeftZ = LeftShoulder.C0:ToEulerAnglesYXZ()
local mouseAngle = math.clamp(Mouse.Hit.Position - Mouse.Origin.Position, minimumAngle, maximumAngle)
TweenService:Create(RightShoulder, TweenInfo.new(0.125), {C0 = (RightShoulder.C0 * CFrame.Angles(0,0, -RightZ)) * CFrame.Angles(0,0, math.asin((mouseAngle).Unit.Y))}):Play()
TweenService:Create(LeftShoulder, TweenInfo.new(0.125), {C0 = (LeftShoulder.C0 * CFrame.Angles(0,0, -LeftZ)) * CFrame.Angles(0,0, math.asin((-mouseAngle).Unit.Y))}):Play()
end
end
end)
1 Like
ate9d
(ate9d)
#3
math.clamp needs a number not a vector3 (Mouse.Hit.Position - Mouse.Origin.Position).
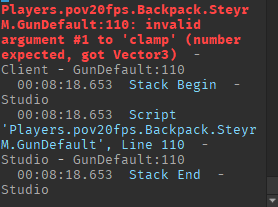
1 Like
Ax1sAng1e
(Pastor James)
#4
Try this?
local minimumAngle = -10
local maximumAngle = 10
RunService.RenderStepped:connect(function(DeltaTime)
if ToolEquipped then
if Mouse.Hit.Position ~= nil then
local RightX, RightY, RightZ = RightShoulder.C0:ToEulerAnglesYXZ()
local LeftX, LeftY, LeftZ = LeftShoulder.C0:ToEulerAnglesYXZ()
local mouseAngle = math.clamp(math.asin(((Mouse.Hit.Position - Mouse.Origin.Position).Unit.Y, minimumAngle, maximumAngle))
TweenService:Create(RightShoulder, TweenInfo.new(0.125), {C0 = (RightShoulder.C0 * CFrame.Angles(0,0, -RightZ)) * CFrame.Angles(0,0,mouseAngle)}):Play()
TweenService:Create(LeftShoulder, TweenInfo.new(0.125), {C0 = (LeftShoulder.C0 * CFrame.Angles(0,0, -LeftZ)) * CFrame.Angles(0,0,-mouseAngle)}):Play()
end
end
end)```