Here’s a solution I found that seems relevant to what you need…
https://devforum.roblox.com/t/part-position-mouse-location/1126174
Remote Events allow Local Scripts and Server Scripts to communicate with each other. You can read more about them here.
In a Local Script, game.Players.LocalPlayer
already refers to the client’s player object, so you don’t need to change their name.
The player’s mouse can only be retrieved in a Local Script.
@JustAGameDeveloper1’s code is on the right lines, but I would rewrite the code using UserInputService
(although how the code is written depends on what you want to do specifically), like so:
-- client code
local UIS = game:GetService("UserInputService")
local player = game.Player.LocalPlayer
local mouse = player:GetMouse()
local brickEvent = game:GetService("ReplicatedStorage").BRICK
UIS.InputChanged:Connect(function(input, gameprocessed)
if input.UserInputType == Enum.UserInputType.MouseMovement then
brickEvent:FireServer(mouse.Hit.Position)
end
end)
-- server code
local brickEvent = game:GetService("ReplicatedStorage").BRICK
brickEvent.OnServerEvent:Connect(function(player, mousePosition)
game.Workspace.MyBrick.Position = mousePosition
end)
Also, the Server and Client code must be in a Server and Local script respectively. Please explain how any code you try doesn’t work, and share any errors that appear.
Here is an example of a Remote Event in use with explanations.
-- this code is in a Local Script
-- the remote event is in `ReplicatedStorage`
local RepStorage = game:GetService("ReplicatedStorage")
local remoteEvent = RepStorage.RemoteEvent
-- sending a message over to the server side of the event
local message = "This is a message from the client!"
remoteEvent:FireServer(message)
-- this code is in a Server Script
-- the remote event also needs to be acquired from
-- ReplicatedStorage in this script, just as it was in the Local Script
local RepStorage = game:GetService("ReplicatedStorage")
local remoteEvent = RepStorage.RemoteEvent -- the same event
-- two arguments get passed through the function which came
-- from the remote event...
-- * player: this is the player who sent the message, not their
name but the ACTUAL player object, and this is the argument
which always appears first before any other argument
-- * message: this is the message which was passed on from
the event when called by the client
local function onServerConnect(player, message)
print(player.Name.." sent a message: "..message)
end
-- fires the onServerConnect function whenever a signal
-- is received from the client (local) side of the event
remoteEvent.OnServerEvent:Connect(onServerConnect)
This is a setup you could have:
- the Remote Event is in
game > ReplicatedStorage
- the Server Script is in
game > ServerScriptService
- the Local Script is in
game > StarterPlayer > StarterPlayerScripts
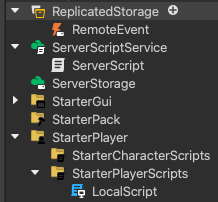