The first thing you want to do is to work out which direction the player’s character is facing:
local Facing = Character.HumanoidRootPart.CFrame.LookVector
Then you want to get the direction from your character to the object:
local Vector = (Object.Position - Character.HumanoidRootPart.Position).unit
Then you can get the angle between those two vectors using the equation:
Light theme users
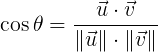
Dark theme users
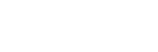
What this means is that the cosine
of the angle between vectors u
and v
is equal to the dot product of u
and v
, divided by the lengths of u
and v
multiplied together. The dot on the top of the fraction represents the dot product, and the dot on the bottom represents just a normal multiplication of two numbers.
This can be rewritten as follows:
Light theme users
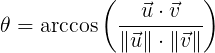
Dark theme users
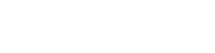
Applying it to Roblox:
-
arccos
is represented by the math.acos
function.
- the dot product can be computed using
u:Dot(v)
.
-
u
and v
are our two vectors we defined at the top which we have called Facing
and Vector
.
- the lengths of those two vectors are both
1
(this is how LookVector is defined and because using .unit returns the vector in the same direction but with length 1
).
Therefore, we can get the angle, combining it with the code we wrote above, with the following:
local Angle = math.acos(Facing:Dot(Vector) / (1 * 1))
Which simplifies to
local Angle = math.acos(Facing:Dot(Vector))
From this point you can just compare the angle and see if it is less than your maximum angle. Note that this angle is in radians, so if you want to compare it to an angle in degrees you’ll need to convert the angle you calculated to degrees using math.deg
.
Hope this is helpful!