Here is what I am doing for my game that is similar to yours:
On the server, and the client I have modules for all the moves,
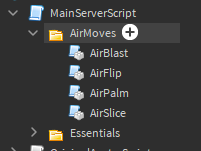
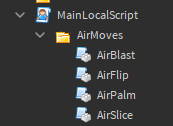
Example of how to set up the settings:
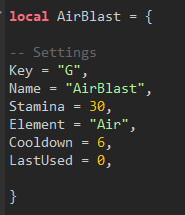
On the local script, I add all the modules to a table and require them if the key is the same as the module.
-- Load Modules
for i,v in pairs(script.Parent.PickElement:GetChildren()) do if v:IsA("TextButton") then
v.MouseButton1Down:Connect(function()
for i,v in pairs(script[Class.."Moves"]:GetChildren()) do
table.insert(MoveModules,v)
end
end)
end
end
-- Input (To Server)
UserInputService.InputBegan:Connect(function(key,chatting)
if chatting or Move_Break then return end
local BP = tostring(key.KeyCode)
local B = string.sub(BP, 14, string.len(BP))
Move_Break = true delay(1,function() Move_Break = false end)
for i,v in pairs(MoveModules) do
local Move = require(v)
if Move.Key == B then
local NewMove = require(v)
NewMove.new(Player.Name, Move.Element, Move.Cooldown, Move.Stamina, Move.LastUsed, Mouse.Hit)
NewMove:DoMove(Player.Name, Mouse.Hit, Mobile)
end
end
end)
On the server, I pass the arguments onto the serversided modules in order to do the stuff that is required to be on the server:
-- Load Modules
for i,v in pairs(script.AirMoves:GetChildren()) do
table.insert(AirMoveModules,v)
end
-- Handle Moves (On Server)
MainE.OnServerEvent:Connect(function(Player, Element, Move, Mobile, Mouse, Side)
if Element == "Air" then
for i,v in pairs(AirMoveModules) do
if Move == v.Name then
local NewMove = require(v)
NewMove.new(Player.Name, NewMove.Element, NewMove.Cooldown, NewMove.Stamina, NewMove.LastUsed, Mouse)
NewMove:DoMove(Player.Name, Mouse, Mobile, Side)
end
end
end
end)
Move_Break prevents someone from firing two moves at once, which is what you originally asked for.
You can either do this or add all your moves into a LocalScript. I would not recommend creating a value as this is easily changed on the client.
That being said, if you want the simplest way to do this, just add a Move_Break variable to your function for getting input, this is what that would look like:
UserInputService.InputBegan:Connect(function(key,chatting)
if chatting or Move_Break then return end
Move_Break = true delay(1,function() Move_Break = false end)
end)