In case anyone visited this thread expecting it to be about an animation gui I’d recommend the GetAnimations
API method.
https://developer.roblox.com/en-us/api-reference/function/KeyframeSequenceProvider/GetAnimations
do
local Enumeration = Enum
local Game = game
local KeyframeSequenceProvider = Game:GetService("KeyframeSequenceProvider")
local MarketplaceService = Game:GetService("MarketplaceService")
local Players = Game:GetService("Players")
local LocalPlayer = Players.LocalPlayer
local AnimationGui = Instance.new("ScreenGui")
AnimationGui.Name = "AnimationGui"
AnimationGui.ResetOnSpawn = false
local AnimationFrame = Instance.new("Frame")
AnimationFrame.Name = "AnimationFrame"
AnimationFrame.Position = UDim2.new(0, 50, 0.5, 0)
AnimationFrame.Size = UDim2.new(0, 250, 0, 150)
AnimationFrame.Style = Enumeration.FrameStyle.RobloxSquare
AnimationFrame.Visible = false
AnimationFrame.Parent = AnimationGui
local function OnGuiTextButtonMouseClick(GuiTextButton)
local State = (GuiTextButton.Style == Enumeration.ButtonStyle.RobloxButton)
GuiTextButton.Style = if State then Enumeration.ButtonStyle.RobloxButtonDefault else Enumeration.ButtonStyle.RobloxButton
GuiTextButton.Text = if State then "👈" else "👉"
AnimationFrame.Visible = State
end
local GuiTextButton = Instance.new("TextButton")
GuiTextButton.Name = "GuiTextButton"
GuiTextButton.Font = Enumeration.Font.GothamMedium
GuiTextButton.Position = UDim2.new(0, 0, 0.5, 0)
GuiTextButton.Size = UDim2.new(0, 50, 0, 50)
GuiTextButton.Style = Enumeration.ButtonStyle.RobloxButton
GuiTextButton.Text = "👉"
GuiTextButton.TextColor3 = Color3.new(1, 1, 1)
GuiTextButton.TextSize = 48
GuiTextButton.MouseButton1Click:Connect(function() OnGuiTextButtonMouseClick(GuiTextButton) end)
GuiTextButton.Parent = AnimationGui
local AnimationsFrame = Instance.new("ScrollingFrame")
AnimationsFrame.Name = "AnimationsFrame"
AnimationsFrame.AutomaticCanvasSize = Enumeration.AutomaticSize.Y
AnimationsFrame.BackgroundTransparency = 1
AnimationsFrame.BorderSizePixel = 0
AnimationsFrame.CanvasSize = UDim2.new(0, 0, 0, 0)
AnimationsFrame.ScrollBarImageColor3 = Color3.new(1, 1, 1)
AnimationsFrame.ScrollBarThickness = 10
AnimationsFrame.Size = UDim2.new(1, 0, 1, 0)
AnimationsFrame.VerticalScrollBarInset = Enumeration.ScrollBarInset.ScrollBar
AnimationsFrame.Parent = AnimationFrame
local UIListLayout = Instance.new("UIListLayout")
UIListLayout.Parent = AnimationsFrame
local Success, Result = pcall(KeyframeSequenceProvider.GetAnimations, KeyframeSequenceProvider, LocalPlayer.UserId)
if not Success then warn(Result) return end
local AnimationIds = {}
while true do
local Page = Result:GetCurrentPage()
for _, Item in ipairs(Page) do
table.insert(AnimationIds, Item)
end
if Result.IsFinished then break end
Result:AdvanceToNextPageAsync()
end
local function OnAnimationStopped(AnimationTextButton)
AnimationTextButton.Style = Enumeration.ButtonStyle.RobloxButton
end
local Tracks = table.create(#AnimationIds)
local function OnAnimationTextButtonMouseClick(AnimationTextButton, AnimationId)
local Character = LocalPlayer.Character
if not Character then return end
local Humanoid = Character:FindFirstChildOfClass("Humanoid")
if not Humanoid then return end
local Animator = Humanoid:FindFirstChildOfClass("Animator")
if not Animator then return end
local State = (AnimationTextButton.Style == Enumeration.ButtonStyle.RobloxButton)
local Track
for _, _Track in ipairs(Tracks) do
local Id = string.match(_Track.Animation.AnimationId, "^rbxassetid://(%d+)")
Id = tonumber(Id)
if Id == AnimationId then Track = _Track break end
end
if State then
AnimationTextButton.Style = Enumeration.ButtonStyle.RobloxButtonDefault
for _, Track in ipairs(Tracks) do
if Track.IsPlaying then Track:Stop() break end
end
if not Track then
local Animation = Instance.new("Animation")
Animation.AnimationId = "rbxassetid://"..AnimationId
Track = Animator:LoadAnimation(Animation)
table.insert(Tracks, Track)
Track.Stopped:Connect(function() OnAnimationStopped(AnimationTextButton) end)
end
Track:Play()
else
if Track then Track:Stop() end
end
end
for _, AnimationId in ipairs(AnimationIds) do
local Success, Result = pcall(MarketplaceService.GetProductInfo, MarketplaceService, AnimationId)
if not Success then warn(Result) continue end
local AnimationTextButton = Instance.new("TextButton")
AnimationTextButton.Name = "AnimationTextButton"
AnimationTextButton.Font = Enumeration.Font.GothamMedium
AnimationTextButton.Size = UDim2.new(1, 0, 0, 50)
AnimationTextButton.Style = Enumeration.ButtonStyle.RobloxButton
AnimationTextButton.Text = Result.Name
AnimationTextButton.TextColor3 = Color3.new(1, 1, 1)
AnimationTextButton.TextSize = 18
AnimationTextButton.MouseButton1Click:Connect(function() OnAnimationTextButtonMouseClick(AnimationTextButton, AnimationId) end)
AnimationTextButton.Parent = AnimationsFrame
end
local function OnCharacterAdded(Character)
table.clear(Tracks)
end
LocalPlayer.CharacterAdded:Connect(OnCharacterAdded)
local Character = LocalPlayer.Character
if Character then task.spawn(OnCharacterAdded, Character) end
local PlayerGui = LocalPlayer:FindFirstChildOfClass("PlayerGui") or LocalPlayer:WaitForChild("PlayerGui", 10)
if not PlayerGui then return end
AnimationGui.Parent = PlayerGui
end
Here’s an example using ‘261’ (Shedletsky) as the user ID.
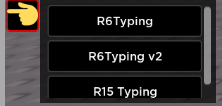