I already did that, did you watch the video closely? Oh right, you probably couldn’t. It was too low quality. Here are some screenshots of my code.
Hierarchy:
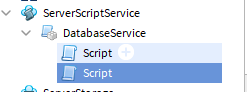
I already did that, did you watch the video closely? Oh right, you probably couldn’t. It was too low quality. Here are some screenshots of my code.
I see a mistake - it’s Value.Value.value
.
You’re going to have to POST to that table and key so you can get the value next time, or else it’s going to return nil.
like this?
sql = require(script.Parent)
game.Workspace.Model.Event.Event:Connect(function()
wait(10)
sql:PostAsync("nuke", "nuke", function(Success, Value, ServerResponse)
sql:GetAsync("nuke", "nuke", function(Success, Value, ServerResponse)
if (Success) then
if Value.Value.value==true then
print('hi')
end
end
end)
local a = true
p(a)
wait(.1)
a = false
p(a)
end)
function p(a)
sql:PostAsync("nuke", "nuke", function(Success, Value, ServerResponse)
if (Success) then
Value.Value.value = true
end
end)
end
and
sql = require(script.Parent)
while wait(.2) do
sql:PostAsync()("nuke", "nuke", function(Success, Value, ServerResponse)
sql:GetAsync()("nuke", "nuke", function(Success, Value, ServerResponse)
if (Success) then
print(Value.Value.value)
end
end)
end
No, you don’t want to post every time, you just need to post once so it isn’t nil.
mySQL:PostASync("nuke","nuke",true, function(Success, Value, ServerResponse)
print(Success)
end)
This will set nuke to true.
Now I am met with these errors.
What I am trying to do here is make it so that when the event is called, it waits 10 seconds then sets the nuke to true then waits .1 seconds then sets it back to false and then the other script checks if the nuke is set to false or true every .2 seconds
Give this a shot.
local mySQL = require(script.Parent)
--// Let's set the value before we try to experiment with it.
mySQL:PostAsync("nuke", "nuke", true, function(Success, Value, ServerResponse)
print(Success) --// Should print 'true' to the console.
end)
wait(5) --// Let's give it some time to ensure the value of nuke is changed.
--// Now let's experiment with the value of 'nuke'
mySQL:GetAsync("nuke", "nuke", function(Success, Value, ServerResponse)
if (Success) then --// If the database found the data.
if Value.Value.value == true then
print("Nuke is true!")
--// What to do if nuke is true.
end
end
end)
Is that script a child of the database module?
We can move to Private Messages if you’d like because this thread has gotten lengthy.
I can use the Spreadsheet API, both GetAsync and PostAsync functional, no errors, nothing. There must be something you’re doing wrong.
Yes. As I have two scripts, and the module cannot be duplicated.
You’re calling PostASync when in the module you posted it was PostAsync. The function doesn’t exist.
Thanks for catching that - I corrected my post.
Hey, ServerResponse (Value)'s “Value” object is a string, not an array.
You can refer to my documentation, although it’s not formatted well.
You should ideally check the “ValueExists” property as well if you need a specific false or true value.
ServerResponse table
{
Success: boolean,
(if not success) Message: string Error Message,
(postAsync) Changes: int
(deleteAsync) KeyDeleted: boolean
(getAsync) ValueExists: boolean
(getAsync) Value: string or null
}
My examples.md page is also incorrect on the PostAsync syntax, although you’ve seem to already figured that one out.
sql:PostAsync(string Table, string Key, string Value [, function Callback]);
This sets (or creates) a value in the database.
Example: sql:PostAsync("table1", "ROBLOX", 'Some Information About Roblox');
If you have any issues, feel free to ask. These are the only two issues I can see off the top of my head.
What do I do though?
What do I do though?
In your GetAsync code that you posted, you’ll want to change “Value.Value.value” to “Value.Value”, and another thing: Values cannot be boolean. This is a limitation with my k/v sqlite3 database. You’ll have to convert the booleans to string (“true”, “false”)
SQLite should automatically convert the PostAsync value to a string, so you should only have to check if it’s equal to true or True.
I still don’t have any idea how to fix this. Here are my scripts.
sql = require(script.Parent)
game.Workspace.Model.Event.Event:Connect(function()
wait(10)
sql:GetAsync("nuke", "nuke", function(Success, Value, ServerResponse)
if (Success) then
if Value.Value==true then
print('hi')
end
end
end)
local a = true
p(a)
wait(.1)
a = false
p(a)
end)
function p(a)
sql:PostAsync("nuke", "nuke", function(Success, Value, ServerResponse)
if (Success) then
Value.Value = true
end
end)
end
And:
sql = require(script.Parent)
while wait(.2) do
sql:GetAsync()("nuke", "nuke", function(Success, Value, ServerResponse)
if (Success) then
print(Value.Value)
end
end)
end
It keeps giving this error. What I am trying to do here is make it so that when the event is called, it waits 10 seconds then sets the nuke to true then waits .1 seconds then sets it back to false and then the other script checks if the nuke is set to false or true every .2 seconds
As I said in my above post, my sqlite3 database doesn’t support booleans. You’ll need to replace the PostAsync code with a string value (“True”, “False”) and then check if it’s equal to one of those inside of your getasync code. You also have extra paranthesis in your GetAsync code causing the Table is unspecified error.
Your PostAsync code doesn’t specify a value, as I told you above my documentation’s syntax is wrong.
sql:GetAsync()("nuke", "nuke", function(Success, Value, ServerResponse)
should be
sql:GetAsync("nuke", "nuke", function(Success, Value, ServerResponse)
The post async code should be
sql:PostAsync("nuke", "nuke", "True", function(Success, Value, ServerResponse)
end)
if Value.Value==true then
should be if Value.Value=="True" then
Thank you, it looks like it’s working but there’s one last problem. Instead of printing if it is true or not, it keeps printing this.
The output is coming from the loop script.
sql = require(script.Parent)
while wait(.2) do
sql:GetAsync("nuke", "nuke", function(Success, Value, ServerResponse)
if (Success) then
print(Value.Value)
end
end)
end
In that case Value.Value
is a table. Have you tried printing its contents via this method:
for a,b in pairs(Value.Value) do
print(a,b)
end