Of course, adding extra checks are usually necessary for cases like these,
@TooMuchRice my post was a rough basis for what you would have done.
You can use the API for that too.
A .Touched Event fires for a base part when it comes in contact with another physical instance. We pass a parameter to a function connected to the Event to detect what actually came in contact with that part.
For instance you have a part called “PartA” and you want to see whether it came in contact with “PartB” , for that you would do :
local partA = workspace:WaitForChild("PartA")
partA.Touched:Connect(function(hit)
-- we can name the parameter anything we want, I named it hit
if hit.Parent.Name == PartB then
print("Part B touched Part A")
end
end)
Now when we use this for players, we can use this to detect whether a player touched a part. (or rather a player’s characters descendant)
local part = workspace:WaitForChild("MyPart")
part.Touched:Connect(function(hit)
if not hit.Parent:FindFirstChild("Humanoid") or hit.Parent == nil then
return
end
-- below this, code will not run
-- if the above criteria returned true, like if hit.Parent was nil
-- then this part will not run, this prevents errors
local char = hit.Parent
local player = game:GetService("Players"):GetPlayerFromCharacter(hit.Parent)
-- do stuff
Basically there is a built in function to return a player instance from a character.
I used game:GetService(“Players”) rather than game.Players because GetService method calls return the service after they have instantiated, otherwise you would have the chances of indexing nil with players, because ‘Players’ wouldn’t have existed, that’s the reason GetService is always the best way to get a Service.
Also an anonymous function is a function that has no variable assigned to it, for example a function would be :
local function OnTouched(hit) -- the hit parameter passed
return print(hit.Name)
end
but if you would want to connect that to an Event, or simply put , run that code when a specific Event fires , you would do
Part.Touched:Connect(OnTouched)
Now if you didn’t have your function pre-defined, you could connect it just like
part.Touched:Connect(function(hit)
-- note how this function doesn't have a name assigned,
-- thre previous one was called OnTouched
My explanation can be wordy, use the API for better on-point information.
Touched Event :
https://developer.roblox.com/en-us/api-reference/event/BasePart/Touched
Anonymous Function :
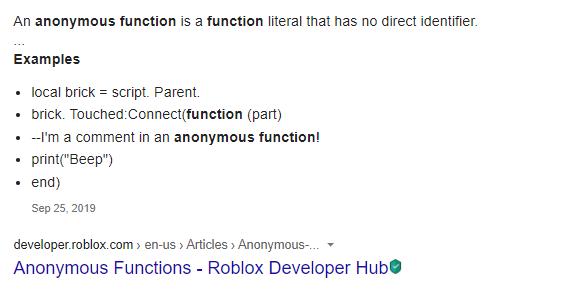
and How do I make an anonymous function?