I have a killbrick but it doesn’t kills me cause my obbys are on client
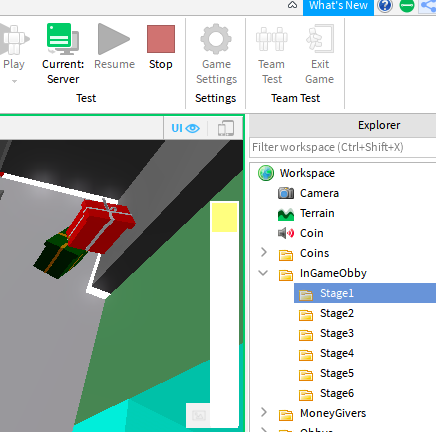
how can i make it client sided so that it kills the person who touches it
I have a killbrick but it doesn’t kills me cause my obbys are on client
You can make LocalScript and change LocalPlayer’s Humanoid health to Zero.
local Humanoid = game:GetService("Players").LocalPlayer.Character.Humanoid
local Brick = --Your Part that kills player on touch
Brick.Touched:Connect(function(touchedPart)
for _, parts in pairs(touchedPart) do
if parts:IsA("BasePart") and parts.Parent:FindFirstChildWhichIsA("Humanoid") then
parts.Parent:FindFirstChildWhichIsA("Humanoid").Health = 0
end
end
end)
can you translate this into local
this is my script in serversided killbrick i used the killbrick activated value on the replicated storage for the invincibility mutator.
local killBrickActivated = game.ReplicatedStorage.KillBrickActivated.Value
script.Parent.Touched:Connect(function(plr)
killBrickActivated = game.ReplicatedStorage.KillBrickActivated.Value
local humanoid = plr.Parent:FindFirstChild("Humanoid")
if killBrickActivated == true then
if humanoid then
print(plr.Name)
print("KillBrick is Activated")
humanoid.Health = 0
end
elseif killBrickActivated == false then
print("KillBrick is not activated")
end
end)
There seems to be no translation needed, you can simply put the code inside LocalScript
Does it print out
print(plr.Name)
print("KillBrick is Activated")
these results?
yeah it doesn’t print out the result and it doesn’t kill me
i think the event brick.Touched only works on server.
Touched
event does fire on client. The problem I could see is that your LocalScript
is descendant of the workspace
.
As you can see in this documentation, LocalScript
only runs on these conditions.
Backpack
, such as a child of a Tool
character
modelPlayerGui
PlayerScripts
.ReplicatedFirst
serviceYou would do it by doing this:
script.Parent.Touched:Connect(function(hit)
if hit.Parent:FindFirstChild("Humanoid")then
hit.Parent.Humanoid.Health = 0
end
Hope this helps.
I don’t think it’s working because the humanoid was located in workspace and not on Players.LocalPlayer.
Try use this inside PlayerGui
Tested and it worked
local player = game.Players.LocalPlayer.Name --get player name so the script can find player's humanoid
local playerpart = workspace:FindFirstChild(player) --the part where Humanoid was located
local Brick = --Part that kills player on touch
Brick.Touched:connect(function()
if playerpart:FindFirstChild("Humanoid") then
playerpart.Humanoid.Health = 0
end
end)
you want the script client sided?