I am currently trying to calculate velocity accurately with real-world formulas. However, I found big chunks of difference between what I am trying to achieve.
Apologies for the lengthy explanation in advance, I am calculating velocity using:
d = .5 * g * t^2 -- distance
t = √(2h / g) -- time taken
v = g * t -- velocity
So in context it’ll look like:
var.elaspedTime += deltaTime -- variable table where i store default values
local gravity = workspace.Gravity -- 21/m^2 | 75
local distFallen = .5 * gravity * var.elaspedTime^2 -- d = .5 * g * t^2
local timeTaken = math.sqrt((2 * distFallen)/gravity) -- t = √(2h / g)
local velocity = gravity * timeTaken -- v = g * t
I expected this to work, so I tried it out and fall 10 studs down, but it returned 5.4930068155436
instead of anywhere remotely near to 10.
So I tried an alternative method I used previously which was subtracting the final Y position from the initial Y position to get the EXACT distance, it looks something like:
if hum:GetState() == Enum.HumanoidStateType.Freefall and rootpart.Velocity.Y < -.5 and not isGrounded() and not var.isFalling then
var.isFalling = true
local maxHeight = rootpart.Position.Y
local maxVelo
repeat
maxVelo = math.abs(math.max(rootpart.Velocity.Y))
runService.Heartbeat:Wait()
until (hum:GetState() ~= Enum.HumanoidStateType.Freefall and hum:GetState() ~= Enum.HumanoidStateType.Jumping) or rootpart.Velocity.Y >= -.5
local gravity = workspace.Gravity
local distFallen = math.max(0, maxHeight - rootpart.CFrame.Y)
local timeTaken = math.sqrt((2 * distFallen)/gravity) -- t = √(2h / g)
local velocity = gravity * timeTaken -- v = g * t
print("---- ACCURATE ----")
print("Distance: " .. distFallen)
print("Time: " .. timeTaken)
print("Velocity: " .. velocity)
print("Actual Velocity: " .. maxVelo)
var.isFalling = false
end
end
This returned an accurate result of 9.202651977539062
, close to being a 10.
In summary, I am just confused as to why my formulas didn’t work as intended or what I have done wrong (unit conversion? wrong formula used?)
Here is a video example:
This is the output:
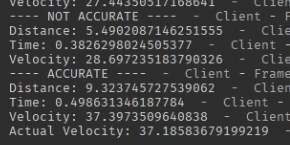
If anyone is good in math or physics please help me out :,) Thanks.