Hello, I made a tags script where it is linked to a group, and you can run a “/tog” command to toggle the tag. The tag works and all, but when the command is ran, it will keep reinserting the table.
local GroupID = XXXX
local presets = {
[100] = {
Settings = {
{TagText = "🤝", TagColor = Color3.fromRGB(85, 170, 255)},
{TagText = "CONTRIBUTOR", TagColor = Color3.fromRGB(85, 170, 255)}
},
ChatColor = Color3.fromRGB(85, 170, 255),
Masked = true
};
[149] = {
Settings = {
{TagText = "MOD", TagColor = Color3.fromRGB(255, 170, 0)}
},
ChatColor = Color3.fromRGB(170, 0, 0),
Masked = true
};
[150] = {
Settings = {
{TagText = "ADMIN", TagColor = Color3.fromRGB(255, 170, 0)}
},
ChatColor = Color3.fromRGB(255, 170, 0),
Masked = true
};
[247] = {
Settings = {
{TagText = "⚒️", TagColor = Color3.fromRGB(170, 170, 255)},
{TagText = "DEVELOPER", TagColor = Color3.fromRGB(170, 170, 255)}
},
ChatColor = Color3.fromRGB(170, 170, 255),
Masked = true
};
[248] = {
Settings = {
{TagText = "⚒️", TagColor = Color3.fromRGB(207, 255, 130)},
{TagText = "Lead Developer", TagColor = Color3.fromRGB(207, 255, 130)}
},
ChatColor = Color3.fromRGB(207, 255, 130),
Masked = true
};
[250] = {
Settings = {
{TagText = "🛡️", TagColor = Color3.fromRGB(255, 255, 0)},
{TagText = "EXECUTIVE", TagColor = Color3.fromRGB(255, 255, 0)}
},
ChatColor = Color3.fromRGB(255, 255, 0),
Masked = true
};
[251] = {
Settings = {
{TagText = "🛡️", TagColor = Color3.fromRGB(255, 255, 0)},
{TagText = "MANAGER", TagColor = Color3.fromRGB(255, 255, 0)}
},
ChatColor = Color3.fromRGB(255, 255, 0),
Masked = true
};
[252] = {
Settings = {
{TagText = "bot", TagColor = Color3.fromRGB(170, 255, 255)}
},
ChatColor = Color3.fromRGB(170, 255, 255),
Masked = false
};
[253] = {
Settings = {
{TagText = "⚒️ 🛡️", TagColor = Color3.fromRGB(41, 122, 0)},
{TagText = "Co Founder", TagColor = Color3.fromRGB(41, 122, 0)}
},
ChatColor = Color3.fromRGB(41, 122, 0),
Masked = true
};
[254] = {
Settings = {
{TagText = "⚒️ 🛡️", TagColor = Color3.fromRGB(222, 148, 0)},
{TagText = "Founder", TagColor = Color3.fromRGB(222, 148, 0)}
},
ChatColor = Color3.fromRGB(222, 148, 0),
Masked = true
};
[255] = {
Settings = {
{TagText = "⚒️ 🛡️", TagColor = Color3.fromRGB(222, 148, 0)},
{TagText = "Founder", TagColor = Color3.fromRGB(222, 148, 0)}
},
ChatColor = Color3.fromRGB(222, 148, 0),
Masked = true
};
}
task.wait()
local ServerScriptService = game:GetService("ServerScriptService")
local ChatService = require(ServerScriptService:WaitForChild("ChatServiceRunner"):WaitForChild("ChatService"))
local Players = game:GetService("Players")
local function ScanIndex(t, v)
for i, r in next, t do
if i == v then
return true
else
return false
end
end
end
local custom_tags = {
}
local joined_WL = {[1] = false}
setmetatable(joined_WL, {
__newindex = function(t, i, v)
warn("NEW INDEX! (" .. tostring(i) .. ")")
end,
})
game.Players.PlayerAdded:Connect(function(plr)
for i,v in pairs(presets) do
if plr:GetRankInGroup(GroupID) == i and ScanIndex(joined_WL, plr.UserId) == false then
joined_WL[plr.UserId] = v.Masked
warn('yee')
plr.Chatted:Connect(function(msg)
local message = string.lower(msg)
if message == string.lower("/tog") then
if joined_WL[plr.UserId] == true and plr:GetRankInGroup(GroupID) == i then
joined_WL[plr.UserId] = false
ChatService:GetSpeaker(plr.Name):SetExtraData('Tags', nil)
ChatService:GetSpeaker(plr.Name):SetExtraData('ChatColor', nil)
game.ReplicatedStorage:WaitForChild("Broadcast"):FireClient(plr, "Tag was toggled OFF", Color3.fromRGB(255, 0, 0))
else
joined_WL[plr.UserId] = true
if plr:GetRankInGroup(GroupID) == i then
ChatService:GetSpeaker(plr.Name):SetExtraData('Tags', v.Settings)
ChatService:GetSpeaker(plr.Name):SetExtraData('ChatColor', v.ChatColor)
game.ReplicatedStorage:WaitForChild("Broadcast"):FireClient(plr, "Tag was toggled ON", Color3.fromRGB(85, 255, 0))
end
end
end
end)
end
end
end)
for i,v in pairs(joined_WL) do
print(tostring(i))
end
ChatService.SpeakerAdded:Connect(function(PlrName)
local Speaker = ChatService:GetSpeaker(PlrName)
for _, g in next, game.Players:GetPlayers() do
if PlrName == g.Name then
for i, v in pairs(presets) do
if g:GetRankInGroup(GroupID) == i then
if joined_WL[g.UserId] == false then
Speaker:SetExtraData('Tags', v.Settings)
Speaker:SetExtraData('ChatColor', v.ChatColor)
game.ReplicatedStorage:WaitForChild("Broadcast"):FireClient(Players[PlrName], "Tag was toggled ON", Color3.fromRGB(85, 255, 0))
elseif joined_WL[g.UserId] == true then
game.ReplicatedStorage:WaitForChild("Broadcast"):FireClient(Players[PlrName], "Tag was toggled OFF", Color3.fromRGB(255, 0, 0))
end
end
end
end
end
end)
p.s: this snippet of the code won’t run.
for i,v in pairs(joined_WL) do
print(tostring(i))
end
ChatService.SpeakerAdded:Connect(function(PlrName)
local Speaker = ChatService:GetSpeaker(PlrName)
for _, g in next, game.Players:GetPlayers() do
if PlrName == g.Name then
for i, v in pairs(presets) do
if g:GetRankInGroup(GroupID) == i then
if joined_WL[g.UserId] == false then
Speaker:SetExtraData('Tags', v.Settings)
Speaker:SetExtraData('ChatColor', v.ChatColor)
game.ReplicatedStorage:WaitForChild("Broadcast"):FireClient(Players[PlrName], "Tag was toggled ON", Color3.fromRGB(85, 255, 0))
elseif joined_WL[g.UserId] == true then
game.ReplicatedStorage:WaitForChild("Broadcast"):FireClient(Players[PlrName], "Tag was toggled OFF", Color3.fromRGB(255, 0, 0))
end
end
end
end
end
end)
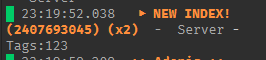