This is invalid table constructor syntax. If you try this simplified example you’ll get the same error:
local a = {something.thing = "test"} -- '}' expected near '='
The whole idea of only tweening something only on the X axis, which I’m guessing you’re trying to do with the first SimpleTween
call, is sound but not something that Roblox/TweenService supports out of the box. You can only tween the entire Position property as a whole, not the individual X, Y or Z components of the Position.
If you want the X tween to run first, then the Y tween, then the solution is to first tween to a position that is whatever you want on the X axis, and exactly what it already is on the Y axis. Vice versa with the second tween.
If you want them to run at the same time but with different tween parameters, then you’re out of luck. That is not something TweenService can do. You’ll have to write some custom code that essentially wraps around TweenService to let you have more control over what exactly happens when a Tween runs.
edit: found some old code I had lying around, feel free to use it however you want:
function tweenFunction(tweenInfo, callback)
local instance = Instance.new("Part") --Class name is arbitrary, just need an instance for TweenService to work with
local property = "Transparency" --Property is also arbitrary, TweenService needs a property though
local tween = TweenS:Create(instance, tweenInfo, {[property] = 1})
local steppedC; steppedC = RunS.Stepped:Connect(function()
callback(instance[property])
end)
tween:Play()
local completedC; completedC = tween.Completed:Connect(function()
if tween.PlaybackState == Enum.PlaybackState.Completed then
callback(1)
end
steppedC:Disconnect()
steppedC = nil
completedC:Disconnect()
completedC = nil
end)
return tween
end
You can use it like so:
local TweenS = game:GetService("TweenService")
local RunS = game:GetService("RunService")
function tweenFunction(tweenInfo, callback)
local instance = Instance.new("Part") --Class name is arbitrary, just need an instance for TweenService to work with
local property = "Transparency" --Property is also arbitrary, TweenService needs a property though
local tween = TweenS:Create(instance, tweenInfo, {[property] = 1})
local steppedC; steppedC = RunS.Stepped:Connect(function()
callback(instance[property])
end)
tween:Play()
local completedC; completedC = tween.Completed:Connect(function()
if tween.PlaybackState == Enum.PlaybackState.Completed then
callback(1)
end
steppedC:Disconnect()
steppedC = nil
completedC:Disconnect()
completedC = nil
end)
return tween
end
local xTweenInfo = TweenInfo.new(3)
local yTweenInfo = TweenInfo.new(3)
local zTweenInfo = TweenInfo.new(3, Enum.EasingStyle.Bounce, Enum.EasingDirection.Out)
local part = game.Workspace.Part
wait(2)
local xTween = tweenFunction(xTweenInfo, function(t)
part.Position = Vector3.new(math.cos(t * math.pi * 2) * 5, part.Position.Y, part.Position.Z) --Only affects X axis
end)
local yTween = tweenFunction(yTweenInfo, function(t)
part.Position = Vector3.new(part.Position.X, math.sin(t * math.pi * 2) * 5, part.Position.Z) --Only affects Y axis
end)
local zTween = tweenFunction(zTweenInfo, function(t)
part.Position = Vector3.new(part.Position.X, part.Position.Y, t * -10) --Only affects Z axis
end)
xTween:Play()
yTween:Play()
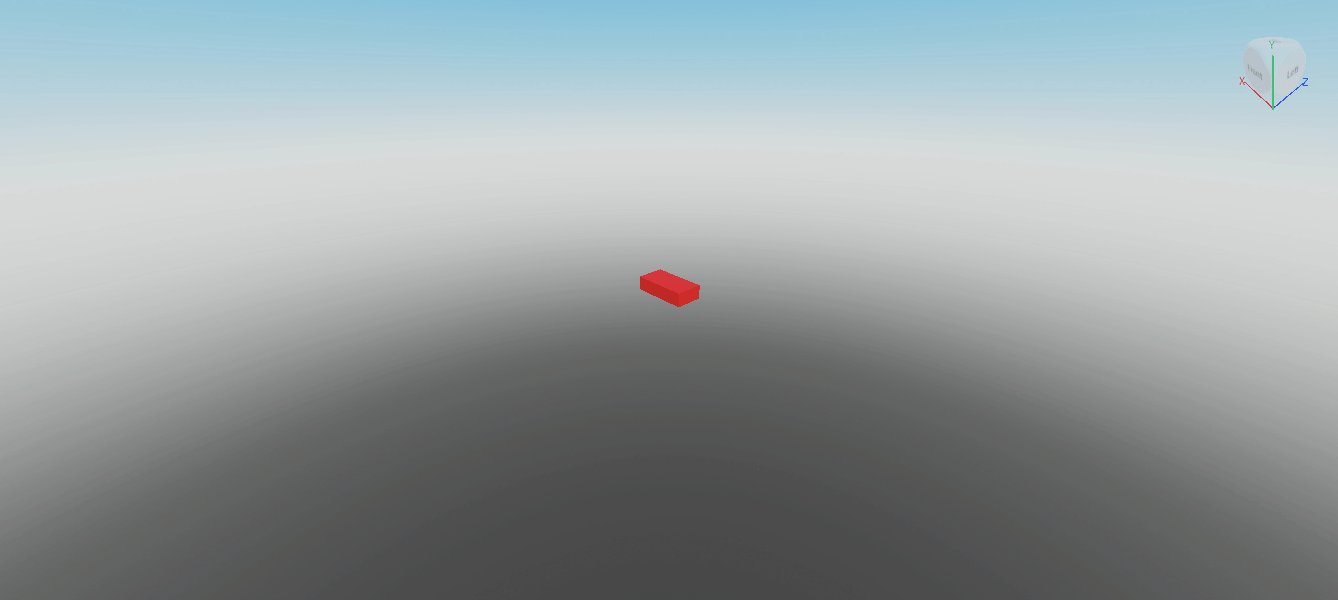