I’m actually gonna think that it’s a problem with the script instead. I think your MoveTo mechanism works perfectly, but the points are not ordered at all making them move to another point that they’re not intended to.
Here’s an example I just made using waypoints in a folder and just simply moving to each one.
Code Example
for _, part in pairs(workspace.Folder:GetChildren()) do
rigHumanoid:MoveTo(part.Position)
rigHumanoid.MoveToFinished:Wait()
end
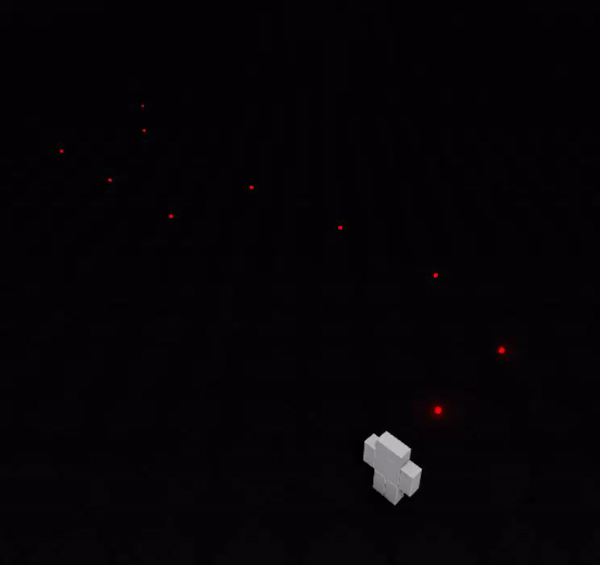
As you can see, the script works. But the logic is still not complete. Although it seems like its skipping, there’s no script determining which waypoint to move to FIRST, having the engine just order by itself.
There are two ways you can do this.
Order by distance
table.sort(waypoints, function(a, b)
local aDistance = (a.Position - rigHumanoid.RootPart.Position).magnitude
local bDistance = (b.Position - rigHumanoid.RootPart.Position).magnitude
return aDistance < bDistance
end)
for _, part in pairs(waypoints) do
rig.Humanoid:MoveTo(part.Position)
rig.Humanoid.MoveToFinished:Wait()
end
This works very well, but you can see flaws that it could still ‘skip’ because one waypoint is actually closer than the other.
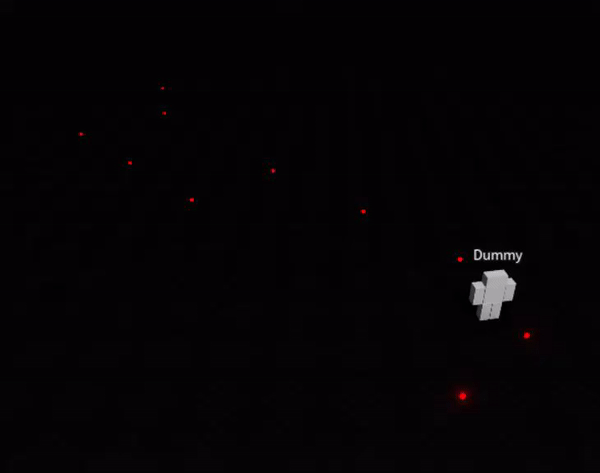
Order by number
table.sort(waypoints, function(a, b)
return tonumber(a.Name) < tonumber(b.Name)
end)
for _, part in pairs(waypoints) do
rig.Humanoid:MoveTo(part.Position)
rig.Humanoid.MoveToFinished:Wait()
end
This works just as well as the first one, but it is ideal if you want it to walk in a specific path, being the perfect method that can be utilized for the game.
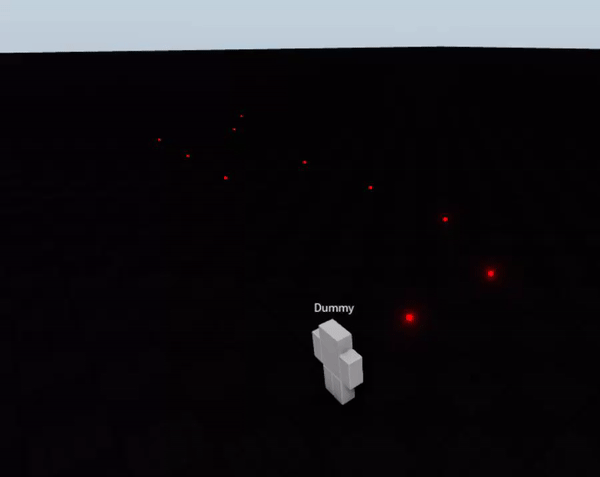
I will attach a place file of this game that shows how each method works, you can find the script inside of the Dummy in the workspace.
Movement Example.rbxl (32.6 KB)
Try numbering your waypoints from closest to furthest (1 to x) and use this in your script and see how it works;
function mob.Move(enemy, map)
local humanoid = enemy.Humanoid
local waypoints = map.WayPoints:GetChildren()
table.sort(waypoints, function(a, b)
return tonumber(a.Name) < tonumber(b.Name)
end)
for _, waypoint in pairs(waypoints) do
humanoid:MoveTo(waypoints[waypoint].Position)
humanoid.MoveToFinished:Wait()
end
end
Mark as solution if this helped you! A little off topic; but a very cool project you’re making. I wish the best of luck 