I’m trying to get the player removed off the table after they step off the brick … Also sorry that my scripting currently lacks proper indentation… Got attempt to compare table on table.sort.
local function onTouch(hit)
if not debounce then
debounce = true
local player = game.Players:GetPlayerFromCharacter(hit.Parent)
if player then
local image = nil
table.insert(ingame,{player.Name})
table.sort(ingame)
whiteboard()
wait()
tabletoboard()
wait (2)
debounce = false
end
end
end
-- works
local function onTouchEnded(hit)
local player = game.Players:GetPlayerFromCharacter(hit.Parent)
if player then
removeplayer(player.Name)
table.sort(ingame) -- got attempt to compare table in output
whiteboard()
wait()
tabletoboard()
end
end
here is the remove player function
function removeplayer(name)
for i = 1,#ingame do
if ingame[i] == name then
table.remove(ingame, i)
end
end
end
I’ve tried to understand what Attempt to compare table too but I can’t figure out what comparison its trying to make.
UPDATE
I’ve rebinded the adding and removal of players to a value/value counter in my game that was already properly adding and removing players so I know that the table is being updated correctly.
The problem I’m still having is that I have a separate board that says the names of the players, the names are being added but when they step off the name is still there. The separate counter which counts the number of values in the index is decreasing though.
part.TouchEnded:connect(function(hit)
-- other stuff
table.remove(touching, index) -- works b/c number decreased
table.sort(touching) -- unsure if this is affecting it or not
tabletoboard() -- this works for the touch but not the touch ended
function tabletoboard() -- ment to update when anyonesteps on or off
for number,d in pairs(touching) do
-- stuff for sending names to the board/ list of player
Basically I’m trying to create an updated list and send it everytime. I don’t understand if the player was removed from the table as shown on the counter, how are they still on the updated board?
1 Like
The problem is that you’re inserting table elements instead of just the player names. That means that if ingame[i] == name then
is comparing a table to a string.
You should just do table.insert(ingame, player.Name)
Tried removing {}, got invalid argument #3 (string expected got nil) on another part of my script trying to pull information from the table.
On the remove player function, try this:
function removeplayer(name)
for i, v in pairs(ingame) do
if v == name then
table.remove(ingame, i)
end
end
end
I added that to the removal function but now I’m getting the attempt to compare table error on the first table.sort for adding players to the table.
Going off of @MayorGnarwhal’s response, an easy fix would probably be to just retrieve the element you have in your table within a table. Right now your ingame table looks like
{ {MayorGnarwhal}, {buildermandiamond}, {salmonbear242}}
so your removeplayer function should look like
function removeplayer(name)
for i = 1,#ingame do
if ingame[i][0] == name then -- the change being [0] after ingame[i]
table.remove(ingame, i)
end
end
end
but I highly recommend you go to the problematic part of your script with invalid argument #3 since your script only happened to be working in the past because you were looking inside of a list, but now you’re looking inside of a string (which doesn’t make sense, which is why you get nil).
Hope this helps!
I implemented your solution for the remove player function and still got attempt to compare table, when I combine it with @MayorGnarwhal solution its Argument #3
See my update, thanks for re - writing my remove function but I scraped that part.
1 Like
In your updated function, I think you meant to use ipairs() instead of pairs(). Also, can you explain why you have a separate table that you’re using to update the board? Why not directly update the board?
Originally I was using the ingame table but in my new draft, everything is held in the touching table. The touching tale is being added and taken away from as shown on my ingame counter but when the actual list is shown it’s still there after the tabletoboard function.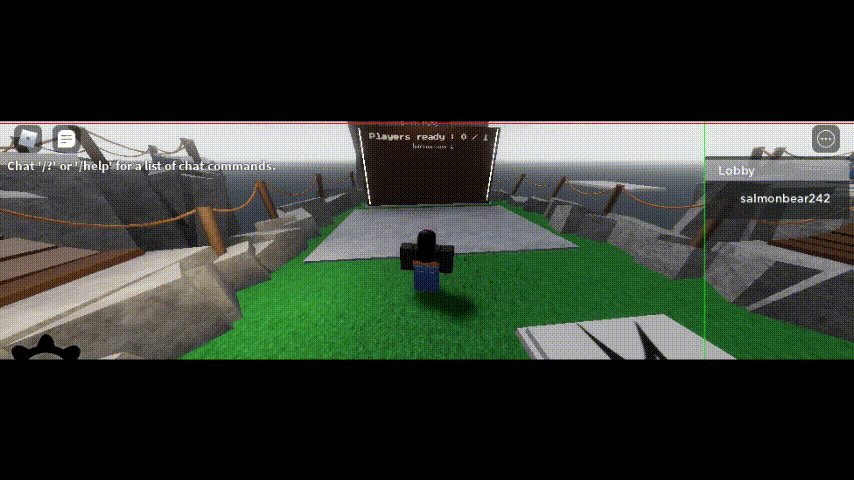
Have you verified that you’re actually being removed from the table and it’s not just an issue where you’re not properly removing the character icon?
Also, it looks like your Players ready is working properly, how does your code for the number of players compare to that of the actual players in the touching table?
I’m going to go ahead and post most of the script
local touching = {} -- this is the table I'm using
function getUserIdFromUsername(name)
-stuff
end
local part = game.Workspace.part
function foundInList(player) -- checking if they are already in the list
for _,target in ipairs(touching) do
if target == player then
return true;
end
end
return false;
end
part.Touched:connect(function(hit)
local player = game.Players:playerFromCharacter(hit.Parent)
if not player then return end
if not foundInList(player) then
table.insert(touching, player)
table.sort(touching)
for number,d in ipairs(touching) do -- puts players CURRENTLY on the list from table to board
game.Workspace.ListOfPlayers.SurfaceGui:findFirstChild(number).Text = d.Name -- works fine
game.Workspace.ListOfPlayers.SurfaceGui:findFirstChild(number).ImageLabel.Image = game:GetService("Players"):GetUserThumbnailAsync(getUserIdFromUsername(d.Name), Enum.ThumbnailType.HeadShot, Enum.ThumbnailSize.Size150x150)
end
end
end)
part.TouchEnded:connect(function(hit)
local player = game.Players:playerFromCharacter(hit.Parent)
if not player then return end
for index, touch in pairs(touching) do
if player == touch then
end
table.remove(touching, index) -- see ** down below to see why I know this part wors
table.sort(touching)
wait()
for number,d in ipairs(touching) do
game.Workspace.ListOfPlayers.SurfaceGui:findFirstChild(number).Text = d.Name -- not updating the list... player does not get removed
game.Workspace.ListOfPlayers.SurfaceGui:findFirstChild(number).ImageLabel.Image = game:GetService("Players"):GetUserThumbnailAsync(getUserIdFromUsername(d.Name), Enum.ThumbnailType.HeadShot, Enum.ThumbnailSize.Size150x150)
end
end
end)
while true do
script.Parent.Text = "Players ready : ".. #touching.." / "..#game.Players:GetPlayers() --** player is removed b/c number went down
wait(0.1)
end
I just want to say thanks for your continuous help so far! @buildermandiamond
1 Like
I just want to say thanks for your continuous help so far!
No problem!
I’m not sure if when you copy and paste, the formatting gets all messed up or if your code is unindented like this. If the latter, please take some time to properly indent so you can have a clear picture of what’s going on.
part.TouchEnded:connect(function(hit)
local player = game.Players:playerFromCharacter(hit.Parent)
if not player then return end
for index, touch in pairs(touching) do
if player == touch then end
table.remove(touching, index) -- see ** down below to see why I know this part wors
table.sort(touching)
wait()
for number,d in ipairs(touching) do
game.Workspace.ListOfPlayers.SurfaceGui:findFirstChild(number).Text = d.Name -- not updating the list... player does not get removed
game.Workspace.ListOfPlayers.SurfaceGui:findFirstChild(number).ImageLabel.Image = game:GetService("Players"):GetUserThumbnailAsync(getUserIdFromUsername(d.Name), Enum.ThumbnailType.HeadShot, Enum.ThumbnailSize.Size150x150)
end
end
end)
Two things
-
Why do you use wait()?
-
When you remove from the table, you are basically going through everything else that exists in the table and remaking the name and image on the board without ever removing the name and imageLabel of the ‘removed’ element. Instead of running a loop, I would set the Text to an empty string and the ImageLabel to nil (haven’t used surfacegui before so not entirely sure this will work) for the player’s index that is no longer touching.
1 Like
Sorry about my formatting, I’ll try to fix that in future post.
- I use wait() because I think I read somewhere it could help with performance or something?? Guess not.
- Because you reminded me I wasn’t removing the player, I realized that my system for adding players to the list is fundamentally flawed. Because I already have the children of the GUI (1, 2, 3 and so on) already layed out, when I remove it it will leave a blank space where that player used to be and the my UIGridTemplate won’t slide down. I’ll have to convert it to a template based system which will avoid many of the problems I’m having with this script.
Thanks!
1 Like
when I remove it it will leave a blank space where that player used to be and the my UIGridTemplate won’t slide down.
Yes that’s one issue I saw that could arise in the future. I would recommend just maintaining the order of the players in the list as they come (instead of sorting each time someone touches or leaves the plate). I feel like if you position the UIGridTemplates in the script beforehand (based on the max number of players that can join) then you could optimize by just setting or resetting some of the templates rather than each and everyone everytime someone begins or ends a touch.
For example,
- If a new player touches, add them to the table and add their name and image to the next available UIGridTemplate
- If a player leaves, remove them from the list and then shift all the players to the right of them in the list to the left on the templates.
1 Like