Hello,
I need help with one small problem.
I have custom character with one cube and if player press “W” then cube move in camera look direction.
All works great in Local Script but i want to make it in normal script
so that other players can see too.
I tried RemoteEvents
and Modules but i cant get that Camera.CFrame.LookVector
.
Can you tell me how to get Camera.CFrame.LookVector
in normal Script
?
Here is my main code for this function:
--// FUNCTIONS //--
UserInputService.InputBegan:Connect(function(Input)
if (Input.UserInputType == Enum.UserInputType.Keyboard) then
if (Input.KeyCode == Enum.KeyCode.W and Character.Config.Health.Value > 0 and Character:FindFirstChild("Base")) then
Character.Moving.Value = true;
Character.Base.Material = Material.Value;
Character.Base.BrickColor = Color.Value;
Character.Base.Move_Start:Play();
repeat
local Direction = game.Workspace.CurrentCamera.CFrame.lookVector;
Character.Base.Velocity = Direction * Max_Speed.Value;
wait(.1);
until Character.Moving.Value == false;
Character.Base.Velocity = Vector3.new(0,0,0);
end;
end;
end);
UserInputService.InputEnded:Connect(function(Input)
if (Input.UserInputType == Enum.UserInputType.Keyboard) then
if (Input.KeyCode == Enum.KeyCode.W and Character.Config.Health.Value > 0 and Character:FindFirstChild("Base")) then
wait(.05)
Character.Moving.Value = false
end;
end;
end);
1 Like
You cannot access from the server a client’s camera because… Well, it is a local camera. Each client has their own local camera which allows them to rotate, zoom and move how they want without being locked to a single camera equal to everyone (it’d be very messy if it wasn’t this way).
To achieve client/server communication you need to use Remotes. Modules are separate, and aren’t about communication.
You should set up a RemoteEvent and use that for communication. Have the client send the server it’s camera informations, and have server handle the event to move the character.
If you need help regarding Remotes and client/server communication, I recommend checking out the following articles:
1 Like
I tryed RemoteEvents and sent camera informations but if i test it cube go to look direction of camera from edit mode.
I can try it again and send here more informations about my script with RemoteEvents.
Don’t have the server handle the repeat.
Instead, have a loop (RunService.RenderStepped) in the client that sends the information to the server when the Moving value is true. Have the client handle the bool, and the server handle the position change.
There is my changed code to RemoteEvents:
--// FUNCTIONS //--
UserInputService.InputBegan:Connect(function(Input)
if (Input.UserInputType == Enum.UserInputType.Keyboard) then
if (Input.KeyCode == Enum.KeyCode.W and Character.Config.Health.Value > 0 and Character:FindFirstChild("Base")) then
local Camera = game:GetService("Workspace").CurrentCamera;
Character:FindFirstChild("Start_Movement_Event"):FireServer(Player, Camera);
end;
end;
end);
UserInputService.InputEnded:Connect(function(Input)
if (Input.UserInputType == Enum.UserInputType.Keyboard) then
if (Input.KeyCode == Enum.KeyCode.W and Character.Config.Health.Value > 0 and Character:FindFirstChild("Base")) then
wait(.05)
Character:FindFirstChild("Stop_Movement_Event"):FireServer();
end;
end;
end);
And here is photo of Character model:
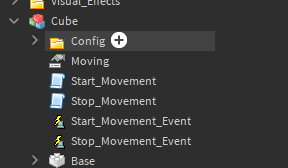
Start_Movement script:
--// VARIABLES //--
local Cube = script.Parent;
local Start_Movement_Event = Cube:WaitForChild("Start_Movement_Event");
local Moving = Cube:WaitForChild("Moving");
local Config = Cube:WaitForChild("Config");
local Material = Config.Material;
local Color = Config.Color;
local Max_Speed = Config.Max_Speed;
--// FUNCTIONS //--
Start_Movement_Event.OnServerEvent:Connect(function(Player, Camera)
Cube.Moving.Value = true;
Cube.Base.Material = Material.Value;
Cube.Base.BrickColor = Color.Value;
Cube.Base.Move_Start:Play();
repeat
local Direction = Camera.CFrame.lookVector;
Cube.Base.Velocity = Direction * Max_Speed.Value;
wait(.1);
until Cube.Moving.Value == false;
Cube.Base.Velocity = Vector3.new(0,0,0);
end);
And Stop_Movement script:
–// VARIABLES //–
l ocal Cube = script.Parent;
local Stop_Movement_Event = Cube:WaitForChild("Stop_Movement_Event");
local Moving = Cube:WaitForChild("Moving");
--// FUNCTIONS //--
Stop_Movement_Event.OnServerEvent:Connect(function()
Moving.Value = false;
end);
Here is error what i get in output:

Again, you shouldn’t be using a repeat loop inside remotes.
Have the client handle the position, and keep updating the server with the new position for the cube.
1 Like
Okey here:
--// FUNCTIONS //--
UserInputService.InputBegan:Connect(function(Input)
if (Input.UserInputType == Enum.UserInputType.Keyboard) then
if (Input.KeyCode == Enum.KeyCode.W and Character.Config.Health.Value > 0 and Character:FindFirstChild("Base")) then
Move = true;
end;
end;
end);
UserInputService.InputEnded:Connect(function(Input)
if (Input.UserInputType == Enum.UserInputType.Keyboard) then
if (Input.KeyCode == Enum.KeyCode.W and Character.Config.Health.Value > 0 and Character:FindFirstChild("Base")) then
Move = false
Character:FindFirstChild("Stop_Movement_Event"):FireServer();
end;
end;
end);
RunService.RenderStepped:Connect(function()
if (Move == true) then
local Camera = game:GetService("Workspace").CurrentCamera;
Character:FindFirstChild("Start_Movement_Event"):FireServer(Player, Camera);
end;
end);
But i still getting that same error in output.