Yeah, that’s a pretty confusing error message IMO 
Check this out:
local Array_InstanceToString: ({Instance}) -> {string} = function()
end
… which gives this error:

Makes sense, the variable type declaration says it should take {Instance} and return {string}, but it takes nothing and returns nothing, so we get that error.
Now check out this:
local Array_InstanceToString: ({Instance}) -> {string}
function Array_InstanceToString(Array)
for i = 1, #Array do
Array[i] = Array[i].Name
end
return Array
end
basically, just declaring first then defining. That gives two errors:

This actually also makes sense. You declared that Array
should be of type {Instance}, but you’re basically doing Array[i] = "string"
, i.e. assigning a string to something that you declared should be an instance. You’re trying to put a string into an instance array. Luau sees this and tries to implicitly convert the string to an Instance, but it can’t so you get that error meassage.
… and
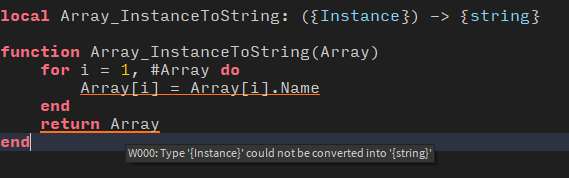
You declared that Array
is of type {Instance} and that the function must return a {string}. When you do return Array
, Array
still has type {Instance} so there’s a mismatch between what you return and what you declared earlier that you would return. Once again, Luau tries to convert your Instance array into a string array, which it can’t so it gives up and gives you the second error message.
The combined error message is still confusing as all heck tho 


Check this out:
Instead of .Name it uses .Parent because I wanted to see if that’s what the error message was referring to. The beginning of the first part is clear, it’s saying that it’s trying to convert from one kind of function to another. The first type is a function of type
({t1}) -> <CYCLE> where t1 = {-Parent: t1 -}
which it tries to convert to the second type:
({Instance}) -> {string}
That whole LoC is one type. It’s a type of function that takes an Instance array and returns a string array.
As for that crazy cycle stuff, my best guess is that Luau figures out that the definition of the type the function takes is cyclical, or self-referential, because it can see that you’re turning objects of type t1
into objects of type t1.Name
(or Parent because I changed it).
The reason that’s an error is that putting string values into an Instance array…
a) You can’t do that
b) It wouldn’t make the Instance array into a string array
The fundamental problem with your function is that you’re trying to put strings into an Instance array. I can see that you’re trying to avoid making a new table and instead do the conversion in place. AFAIK Luau doesn’t have an “as” keyword or any other way of treating an array of one type as if it were an array of a different type.
To fix your function, do something like
local Array_InstanceToString: ({Instance}) -> {string} = function(Array)
local Result = {}
for i = 1, #Array do
Result[i] = Array[i].Name
end
return Result
end
or even
local Array_InstanceToString: ({Instance}) -> {string} = function(Array)
local Result: {string} = {}
for i = 1, #Array do
Result[i] = Array[i].Name
end
return Result
end
which gives no errors but does allocate a new table for the return value.