local function lerp(p0,p1,t)
return p0*(1-t) + p1*t
end
local function Quad(p0,p1,p2, t)
local L1 = lerp(p0,p1,t)
local L2 = lerp(p1,p2,t)
local Quad = lerp(L1,L2,t)
return Quad
end
local Middle = (End + Start) /2 + Type
coroutine.resume(coroutine.create(function()
for i = 1,Loops do
local t = i/Loops
local updated = Quad(Start,Middle,End,t)
TweenService:Create(Part,TweenInfo.new(t / 100,Enum.EasingStyle.Linear,Enum.EasingDirection.Out),{Position = updated}):Play()
RunService.Stepped:Wait()
end
Can someone please explain this Bezier Formulae? Im very confused with whats happening but i know what you can do with this code.
Thanks!
It isn’t all there, so some is a bit hard to decipher.
Generally, the lerp takes two points and a “time” variable (that ranges from 0 to 1). Think of this as returning a position value along a line from p0 to p1 at time t. In other words, when t=0 the position value returned is the first point. When t=1 it is the second point, and when t is between 0 and 1 the position is somewhere on the line between p0 and p1.
The Quad function is presumably short for “Quadratic Bezier”. It accepts 3 points and a “time” var that was discussed above. To visualize what that looks like, think of two sticks tied together at one end (can be bent into ‘L’ shapes with various angles). If you were to tie strings along one stick and attach them to the opposite end of the other stick, you’d end up with something like this (from Wikipedia page on Bezier curves):
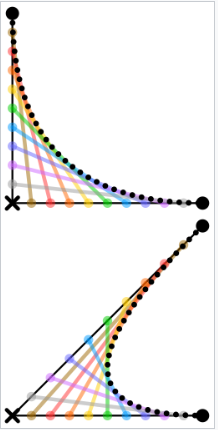
The 3 points passed to the fn would represent the big dots on the ends and the ‘x’ in the middle. The first lerp in the Quad fn finds a location (at time t) along one stick and the second finds a corresponding location along the other stick in the opposite direction (L1 and L2). The two positions are then passed to a third lerp that returns the position of the dot along the curve (finds loc along string rather than along one of the sticks; Rather than finding the spot where strings cross it just uses the ‘t’ value). So the Quad fn is returning a dot location along the curve for a given value of ‘t’ and 3 end points (start, mid, end).
Not sure what “Type” is supplying, but I assume it’s being used to calculate the middle point that’s labeled with the ‘x’ in the graphic.
It then creates a coroutine (code that runs in it’s own thread) and “resumes” it (the coroutine returned by ‘create’ doesn’t start running automatically, so this just starts the thing running). The “Loops” in this case give the resolution of the curve (more loop iterations means more “strings”, so more dots along the curve).
The TweenService bit is moving the position of “Part” from where it is currently to each new dot as they are calculated (over amount of time specified as ‘t / 100’, which I guess would make it go slower as it reaches the end of the curve).
The RunService…Wait() is just a fancy pause between each dot calculation.
Anyway, that’s how it looks to me.
1 Like
Thanks alot! This really helped!
1 Like