I need help with a little problem I can’t quite figure out.
My goal is to change the size of a ParticleEmitter depending on its parent’s own size via script. (as the title suggests)
I made two yellow stars which can transform into blue stars. The only difference is one is smaller and the other is bigger.
Here’s the view on the problem:
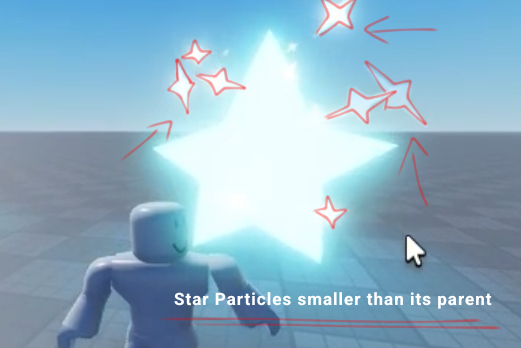
What I want the particles to look like:
ServerScript:
local ReplicatedStorage = game:GetService("ReplicatedStorage")
local RemoteEvent = ReplicatedStorage:WaitForChild("RemoteEvent")
local CollectionService = game:GetService("CollectionService")
local TS = game:GetService("TweenService")
local Debris = game:GetService("Debris")
RemoteEvent.OnServerEvent:Connect(function(player, MouseTarget)
local Character = player.Character
if Character then
if CollectionService:HasTag(MouseTarget, "Star") then
-- Tweens
local GlitterSound = MouseTarget:FindFirstChild("GlitterSound")
local PointLight = MouseTarget:FindFirstChildWhichIsA("PointLight")
if PointLight then
local LightInfo = TweenInfo.new(4, Enum.EasingStyle.Circular, Enum.EasingDirection.Out)
local Light = {Color = Color3.fromRGB(37, 208, 255)}
local LightTween = TS:Create(PointLight, LightInfo, Light)
LightTween:Play()
end
local HoverInfo = TweenInfo.new(3, Enum.EasingStyle.Sine, Enum.EasingDirection.InOut, 0, true)
local Hover = {CFrame = MouseTarget.CFrame * CFrame.new(0, 5, 0) * CFrame.fromEulerAnglesXYZ(0, 180, 0)}
local ColorInfo = TweenInfo.new(2, Enum.EasingStyle.Linear)
local ColorChange = {Color = Color3.fromRGB(119, 255, 250)}
local HoverTween = TS:Create(MouseTarget, HoverInfo, Hover)
local ColorTween = TS:Create(MouseTarget, ColorInfo, ColorChange)
HoverTween:Play()
ColorTween:Play()
GlitterSound:Play()
-- Particles
local ParticleEmitter = script.ParticleEmitter:Clone()
ParticleEmitter.Enabled = true
ParticleEmitter.Parent = MouseTarget
HoverTween.Completed:Connect(function()
ParticleEmitter.Enabled = false
Debris:AddItem(ParticleEmitter, 1)
end)
end
end
end)
Any ideas on how to possibly calculate this? If you have any suggestions, please share!