We can actually use some trigonometry for this! Which if you’re not familiar with, it is ideal to learn it before reading this solution!
In trig, each trig function (sin, cos, tan) have a certain sign in the 4 quadrants of the cartesian plane.
So to put it simply, in the first quadrant (a quadrant is one of the squares that make the plane) or in your picture is deined as section A, any triagnle built there will have all the trig functions be positive, in N°2 only the sin, in N°3 only tan, in N°4 only cos.
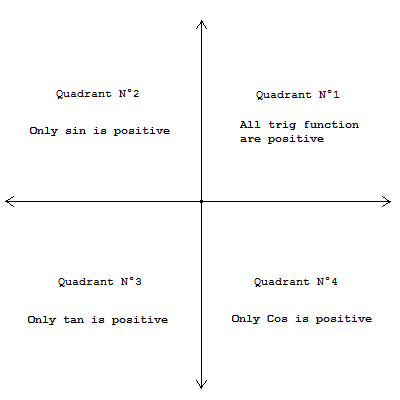
Things might’ve become complicated, but let me demonstrate: Let’s say the mousePos is in the first quadrant, we wanna prove that it’s there!
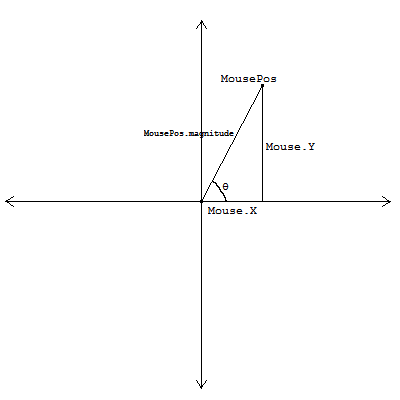
As you can see, the triangle formed by the mousePos (where the opposite side is Mouse.Y
the adjacent is Mouse.X
and the hypotunuse is the MousePos’s length or in other words MousePos.magnitude
, and we have the angle θ which we are going to use the trig function on) is found in the first quadrant, meaning that what we have to do is as follows: check if sin(θ) and cos(θ) and tan(θ)
are all positive.
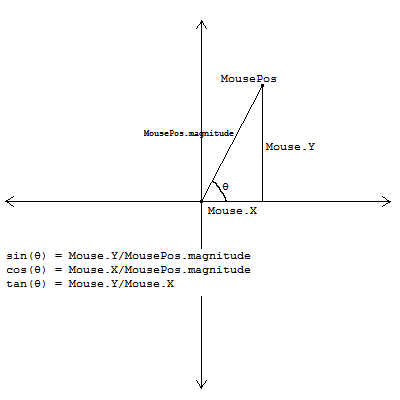
This example shows what happens if mousePos is in first quadrant
And that’s actually it! It works. We calculate the sin(θ) and cos(θ) and tan(θ)
and then we put all of our if statments, if all are positive than the mousePos is in first quadrant, if just sin is positive then mousePos is in second quadrant, if just tan is positive than it’s in third quadrant, if just cos is positive than it’s in fourth quadrant.
And we can use math.sign() to check if it’s negative or positive, this function returns 1 if it’s positive, and returns -1 if it’s negative (it does as well return 0 if the input was 0, but in this situation we shouldn’t meet a case where it’s 0)
local adjacent = mouse.X
local opposite = mouse.Y
local hypotenuse = Vector2(adjacent, hypotenuse).magnitude --there isn't a mouse.Position so we make a 2d vector with the mouse x and y and .magnitude is that vector's length
if math.sign(opposite/hypotenuse) == 1 and math.sing(adjacent/hypotenuse) == 1 and math.sign(opposite/adjacent) == 1 then
print("mouse is in section A")
elseif math.sign(opposite/hypotenuse) == 1 then
print("mouse is in section B")
elseif math.sign(opposite/adjcent) == 1then
print("mouse is in section C")
elseif math.sign(adjacent/hypotenuse) == 1 then
print("mouse is in section D")
end
There we go! Not sure if it works because I have no means of testing this, but hopefully it does!
And sorry if I didn’t go in depth in explaining this, the basic stuff, because most of it you gotta learn before reading this! So yeah, learn trigonometry, and if you got any question ask