So A GUI with buttons, that when clicked, telelport them? Question also becomes if you want to hook this up to a datastore to save when the leave, and reload on rejoin.
I just made I checkpoint system myself so idc if I share my code with you
Here are my setup recommendations:
Make 2 folders, One for when the player touches this part, we will record them touching it, and the other folder, for the location the player will be teleported to. We will also add a Object, and a IntValue in the ‘player touches this part’ in the folder. Example:
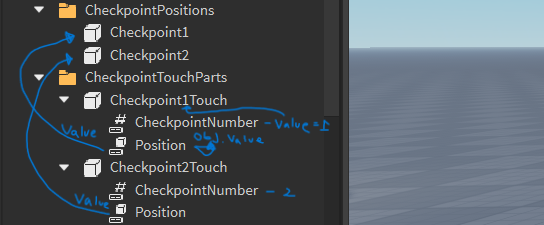
(PS. the parts can be invisible and cancollide off if you don’t want the player to see them.)
Also Have a remote Event in rep. stroage. We will use this to teleport the player on the server, as well as a server script in server script service with this code:
game.ReplicatedStorage.TeleportPlayer.OnServerEvent:Connect(function(player, part)
player.Character:PivotTo(part:GetPivot())
end)
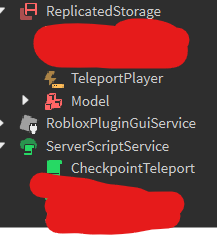
I had this GUI set up
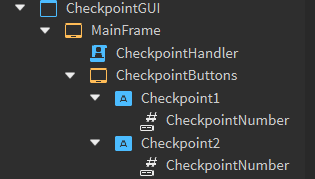
Then inside that local script I did this, and boom, it works:
local UnlockedCheckpoints = {}
local plr = game.Players.LocalPlayer
for i, v in game.Workspace.CheckpointTouchParts:GetChildren() do
if v:IsA("Part") then
v.Touched:Connect(function(hit)
if hit.Parent == plr.Character and table.find(UnlockedCheckpoints, v:FindFirstChildWhichIsA("IntValue").Value) == nil then
table.insert(UnlockedCheckpoints, v:FindFirstChildWhichIsA("IntValue").Value)
end
end)
end
end
for i, v in script.Parent.CheckpointButtons:GetChildren() do
if v:IsA("TextButton") then
v.MouseButton1Click:Connect(function()
if table.find(UnlockedCheckpoints, v:FindFirstChildWhichIsA("IntValue").Value) then
local PosPart = nil
for i, TouchParts in game.Workspace.CheckpointTouchParts:GetChildren() do
if TouchParts:IsA("Part") and v:FindFirstChildWhichIsA("IntValue").Value == TouchParts:FindFirstChildWhichIsA("IntValue").Value then
PosPart = TouchParts:FindFirstChildWhichIsA("ObjectValue").Value
end
end
if PosPart == nil then
error("No Position Part Found")
else
game.ReplicatedStorage.TeleportPlayer:FireServer(PosPart)
end
end
end)
end
end
Hope this helps and lmk if you have questions!