Here is a simple script I have for client sided knockback:
local Att = Instance.new("Attachment", VictimRP)
local Force
local Upper = nil
if UpV == nil then
Upper = 1
else
Upper = UpV
end
EnemyHum.PlatformStand = true
EnemyHum.AutoRotate = false
Force = Instance.new("LinearVelocity")
Force.Attachment0 = Att
Force.VectorVelocity = (Dir + Vector3.new(0,UpV,0)).Unit * (KBPower / 100)
Force.RelativeTo = Enum.ActuatorRelativeTo.World
Force.MaxForce = math.huge
Force.Parent = VictimRP
local Rot = Instance.new("AngularVelocity")
Rot.Attachment0 = Att
Rot.AngularVelocity = Vector3.new(1, 1, 1) * Rotation
Rot.MaxTorque = math.huge
Rot.RelativeTo = Enum.ActuatorRelativeTo.Attachment0
Rot.Parent = VictimRP
game.Debris:AddItem(Force, 0.2)
game.Debris:AddItem(Rot, 0.2)
game.Debris:AddItem(Att, 0.2)
task.wait(0.7)
EnemyHum.PlatformStand = false
EnemyHum.AutoRotate = true
RP.Orientation = Vector3.new(0,RP.Orientation.Y,0)
The issue lies with the last line. I need to keep it in order to prevent the player from tripping if he is on the ground (before suggesting to just replace this line, please know that this is the only working solution I found to prevent tripping, trust me when I say I’ve tried everything).
It works exactly as intended, except the hit player is permanently twisted in a random direction, as shown in the photo. This ONLY appears for the hit player’s client; the hit player looks normal on the server. Does anybody know how I can fix this?
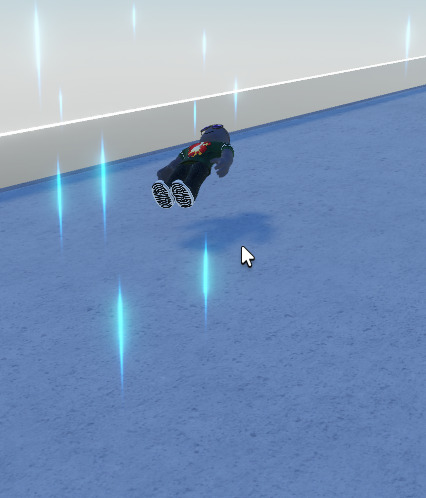
1 Like
assigning a physics constraint to a player on the client will only have an effect for the player whos localscript applies the force. you could apply it to another player and you will see the effect but if the effect isnt replicated for the other player, that target player wont see a thing.
either replicate it using remote events or send an event to the server and have the server apply the physics objects so everyone sees it.
As for the twisting, you are using angular velocity constraints and setting them relative to one of the attachments. you just need to tween the properties of the constraint and the orientation of the attachments.
you set the maxForce to math.huge meaning nothing can over power it. so yeah it will have a permanent effect. which isnt wrong to do, it just explains the locking in place and twisting
Thank you for responding!
Replicating it for all players makes the entire KB super choppy, which defeats the point of it being client sided. About what you said afterwards, does that mean that even though the forces are destroyed, they are still applied, which causes the “stuck” effect?
no, if the constraints are destroyed then no force should be applied. If there is then something else is happening. either there are duplicate constraints or you have something else on the plyr character.
You dont need it to be on the server, you can replicate it to the other clients using remote events. thats how most shooters work. they copy the bullet’s info, send the info to the server and the server sends the info to all other clients besides the sender, and the clients do the hard work of spawning the bullet based on the recieved info
That is my issue. I am certain there is nothing else influencing the player. Their orientation just gets messed up on their client, and I just need to know how to reset it.
why are you so certain? what have you checked? just asking for clarification
have you checked the character in explorer for both client and server?
I have. No other scripts create velocities or forces, and there are no other parts welded to the player. I just have no idea why the orientation is only messed up on the client.
its messed up only on the client because you create the objects on a local script. local scripts only run on the client. every client has their own version of that local script.
I’m aware of that. Do you know how I can reset the player to a neutral position once they’re messed up?
i dont have enough information for that answer.
For one, i dont see any code destroying the objects.
Two, i dont have access to the explorer so i cant be 100% sure you checked everything
I see. They are destroyed with debris service. Thank you for trying to help. I’ll keep trying to find a solution on my own 
why dont you just use Instance:Destroy() ??
I tried that already. Nothing changes.
can you show the version of the code where you try that?
because if that doesnt work then you either anchor the player in place or you do have duplicate objects somewhere
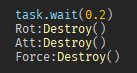
Everything is the same, just replace the debris part with that
now can you run the script with that new code and if the issue happens take a screenshot of whatever part you set VictimRP to?
I wanna see it in the client and server views while the game is running.
Don’t worry, I finally found a workaround for trip-cancelling that doesn’t involve that single tediously buggy line of code. Sorry for wasting your time
, but have a great day!