Children of cloned bullet object are not recognized after the clone.
local b
local start
local tr
if config:FindFirstChild('trailBullets') then
if config.trailBullets.Value then
b = game:GetService('ReplicatedStorage').trailBullet:Clone()
b.outerTrail.Color = ColorSequence.new(rayColor.Value)
start = CFrame.new(bPos,targetCF.p)
tr = true
else
if not isLaser.Value then
b = game:GetService('ReplicatedStorage').realBullet:Clone()
start = CFrame.new(bPos,targetCF.p) * CFrame.new(0,0,-2)
else
b = game:GetService('ReplicatedStorage').bullet:Clone()
b:FindFirstChild("SurfaceLight").Color = rayColor.Value
b:FindFirstChild("ParticleEmitter").Color = ColorSequence.new(rayColor.Value)
b:FindFirstChild("ParticleEmitter"):Emit(40)
b:FindFirstChild("ParticleEmitter2").Color = ColorSequence.new(rayColor.Value)
b:FindFirstChild("ParticleEmitter2"):Emit(.5)
start = CFrame.new(bPos,targetCF.p) * CFrame.new(0,0,-2)
end
end
else
if not isLaser.Value then
b = game:GetService('ReplicatedStorage').realBullet:Clone()
start = CFrame.new(bPos,targetCF.p) * CFrame.new(0,0,-2)
else
b = game:GetService('ReplicatedStorage'):WaitForChild("bullet"):Clone()
--b.SurfaceLight.Color = rayColor.Value
b:WaitForChild("ParticleEmitter").Color = ColorSequence.new(rayColor.Value)
b:FindFirstChild("ParticleEmitter"):Emit(50)
b:FindFirstChild("ParticleEmitter2").Color = ColorSequence.new(rayColor.Value)
b:FindFirstChild("ParticleEmitter2"):Emit(.5)
start = CFrame.new(bPos,targetCF.p) * CFrame.new(0,0,-2)
end
end
This line -
b:WaitForChild(âParticleEmitterâ).Color = ColorSequence.new(rayColor.Value)
prints the following error message.
Line 213 is depicted here.
Image of bullet Model
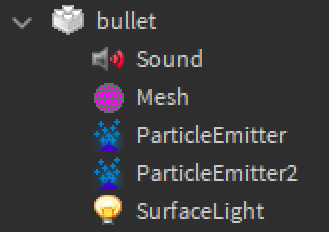
I have tried changing around the initial parent of the bullet, but the clone script itself does not error. I have also tried changing out the normal â.â that was there in between it and the object name, with âWaitForChildâ.
Edit 1: Full Function if needed.
local fire = function(mP,bPos)
shootAnim:Play()
for _,v in next,barrel:GetChildren() do
if v:IsA('ParticleEmitter') then
v.Enabled = true
delay(.02,function()
for _,v in next,barrel:GetChildren() do
if v:IsA('ParticleEmitter') then
v.Enabled = false
end
end
end)
end
end
local targetCF = mP + Vector3.new(rand:NextNumber(-config.spread.Value,config.spread.Value),rand:NextNumber(-config.spread.Value,config.spread.Value),0)
local r
local reflectFactor = math.rad(math.random(160,200))
local b
local start
local tr
if config:FindFirstChild('trailBullets') then
if config.trailBullets.Value then
b = game:GetService('ReplicatedStorage').trailBullet:Clone()
b.outerTrail.Color = ColorSequence.new(rayColor.Value)
start = CFrame.new(bPos,targetCF.p)
tr = true
else
if not isLaser.Value then
b = game:GetService('ReplicatedStorage').realBullet:Clone()
start = CFrame.new(bPos,targetCF.p) * CFrame.new(0,0,-2)
else
b = game:GetService('ReplicatedStorage').bullet:Clone()
b:FindFirstChild("SurfaceLight").Color = rayColor.Value
b:FindFirstChild("ParticleEmitter").Color = ColorSequence.new(rayColor.Value)
b:FindFirstChild("ParticleEmitter"):Emit(40)
b:FindFirstChild("ParticleEmitter2").Color = ColorSequence.new(rayColor.Value)
b:FindFirstChild("ParticleEmitter2"):Emit(.5)
start = CFrame.new(bPos,targetCF.p) * CFrame.new(0,0,-2)
end
end
else
if not isLaser.Value then
b = game:GetService('ReplicatedStorage').realBullet:Clone()
start = CFrame.new(bPos,targetCF.p) * CFrame.new(0,0,-2)
else
b = game:GetService('ReplicatedStorage'):WaitForChild("bullet"):Clone()
b.Parent = workspace.rayStorage
b.SurfaceLight.Color = rayColor.Value
b:WaitForChild("ParticleEmitter").Color = ColorSequence.new(rayColor.Value)
b:FindFirstChild("ParticleEmitter"):Emit(50)
b:FindFirstChild("ParticleEmitter2").Color = ColorSequence.new(rayColor.Value)
b:FindFirstChild("ParticleEmitter2"):Emit(.5)
start = CFrame.new(bPos,targetCF.p) * CFrame.new(0,0,-2)
end
end
b.CFrame = start
b.Parent = workspace.rayStorage
flash(barrel.flash)
fireSound:Play()
if #plrs:GetPlayers() > 1 then
render:FireServer(bPos,targetCF,rayColor.Value,speed.Value,ignores,isLaser.Value,reflectFactor,barrel,fireSound,tr)
end
ammo.Value = ammo.Value - 1
updateGUI()
local latency = 0
local lastStep = bPos
spawn(function()
while (b.CFrame.p-start.p).magnitude < 3000 do
latency = run.RenderStepped:Wait()
if not isLaser.Value then
b.CFrame = b.CFrame*CFrame.new(0,0,-latency*speed.Value)*CFrame.Angles(math.rad(-.1),0,0)
else
b.CFrame = b.CFrame*CFrame.new(0,0,-latency*speed.Value)
end
local part,position,norm = workspace:FindPartOnRayWithIgnoreList(Ray.new(lastStep,(b.Position-lastStep).unit*(lastStep-b.Position).magnitude),ignores)
if part and part.Name == 'reflect' and not r then
b.CFrame = b.CFrame*CFrame.Angles(0,reflectFactor,0)
r = true
end
--[[ if part and part:IsDescendantOf(char) and r then
if part.Parent:FindFirstChild('Humanoid') then
damageEvent:FireServer(dmg.Value,part.Parent.Humanoid)
elseif part.Parent.Parent:FindFirstChild('Humanoid') then
damageEvent:FireServer(dmg.Value,part.Parent.Parent.Humanoid)
end
end]]
if part and not part:IsDescendantOf(char) then
local h = game:GetService('ReplicatedStorage').hit:Clone()
h.Parent = workspace.rayStorage
h.CFrame = CFrame.new(position,position+norm)
h.Smoke1:Emit(15)
h.Smoke2:Emit(12)
h.Sparks:Emit(30)
h.Explosion:Emit(15)
h.Sound:Play()
game:GetService('Debris'):AddItem(h,2)
for _,v in next,h:GetChildren()do
if v:IsA('ParticleEmitter') or v:IsA('PointLight') then
v.Enabled = true
end
end
delay(.1,function()
for _,v in next,h:GetChildren()do
if v:IsA('ParticleEmitter') or v:IsA('PointLight') then
v.Enabled = false
end
end
end)
if part.Name ~= 'reflect' then
if exp.Value then
explodeEvent:FireServer(position)
end
local hum = part.Parent:FindFirstChildOfClass('Humanoid') or part.Parent.Parent:FindFirstChildOfClass('Humanoid') or (part.Parent.Parent.Parent and part.Parent.Parent.Parent:FindFirstChildOfClass('Humanoid'))
if hum then
if tk.Value then
damageEvent:FireServer(dmg.Value,hum)
spawn(flashHitmarker)
hitmark:Play()
else
local tplr = plrs:GetPlayerFromCharacter(hum.Parent)
if tplr then
if tplr.Team ~= plr.Team then
damageEvent:FireServer(dmg.Value,hum)
spawn(flashHitmarker)
hitmark:Play()
end
else
damageEvent:FireServer(dmg.Value,hum)
spawn(flashHitmarker)
hitmark:Play()
end
end
end
end
break
end
lastStep = b.Position
end
if config:FindFirstChild('trailBullets') then
if config.trailBullets.Value == true then
wait(3)
b:Destroy()
else
b:Destroy()
end
else
b:Destroy()
end
end)
end