Script:
local Services = {
Players = game:GetService("Players");
ReplicatedStorage = game:GetService("ReplicatedStorage");
UserInputService = game:GetService("UserInputService");
TweenService = game:GetService("TweenService");
RunService = game:GetService("RunService")
}
local Player = Services.Players.LocalPlayer
local Character = Player.Character or Player.CharacterAdded:Wait()
local Humanoid = Character:WaitForChild("Humanoid")
local Root = Character:WaitForChild("HumanoidRootPart")
local DefaultAutoRotate = Humanoid.AutoRotate
print(DefaultAutoRotate)
local Camera = workspace.CurrentCamera
local CameraMinZoomDistance = Player.CameraMinZoomDistance
local CameraMaxZoomDistance = Player.CameraMaxZoomDistance
local DefaultCameraOffset = Humanoid.CameraOffset
local Mouse = Player:GetMouse()
local DefaultMouseIcon = Mouse.Icon
local AimDistance = 10
local UnaimDistance = 16
local Status = {
isEquipped = false;
isAiming = false;
canShoot = true;
isReloading = false;
startedLaser = false;
}
local Folders = {
RemoteEvents = Services.ReplicatedStorage.Remotes.Events;
RemoteFunctions = Services.ReplicatedStorage.Remotes.Functions;
}
local Remotes = {
DetectGun = Folders.RemoteFunctions.DetectGun;
FireGun = Folders.RemoteFunctions.FireGun;
GetGunSettings = Folders.RemoteFunctions.GetGunSettings;
ReloadGun = Folders.RemoteFunctions.ReloadGun;
GetAmmoCount = Folders.RemoteFunctions.GetAmmoCount;
GetMouseHitLocation = Folders.RemoteFunctions.GetMouseHitLocation;
StartCloseLaser = Folders.RemoteFunctions.StartCloseLaser;
}
local Modules = {
CameraShaker = require(Services.ReplicatedStorage.Libs.CameraShaker);
}
local GunSettings
GunSettings = Remotes.GetGunSettings:InvokeServer()
local PlayerAnims = {}
local Sounds = {
AimDownSound = script.AimDownSound;
AimUpSound = script.AimUpSound;
}
local Tweens = {
AimInTween = TweenInfo.new(0.5, Enum.EasingStyle.Quad, Enum.EasingDirection.Out, 0, false, 0);
AimOutTween = TweenInfo.new(0.5, Enum.EasingStyle.Quad, Enum.EasingDirection.Out, 0, false, 0);
RootTween = TweenInfo.new(0.01, Enum.EasingStyle.Sine, Enum.EasingDirection.Out, 0, false, 0);
CameraTweenAim = TweenInfo.new(0.4, Enum.EasingStyle.Sine, Enum.EasingDirection.Out, 0, false, 0);
CameraTweenUnAim = TweenInfo.new(0.4, Enum.EasingStyle.Sine, Enum.EasingDirection.Out, 0, false, 0);
}
local CameraTweenAim = Services.TweenService:Create(Player, Tweens.AimInTween, {CameraMinZoomDistance = AimDistance, CameraMaxZoomDistance = AimDistance})
local CameraTweenUnAim = Services.TweenService:Create(Player, Tweens.AimOutTween, {CameraMinZoomDistance = UnaimDistance, CameraMaxZoomDistance = UnaimDistance})
local tool = nil
local currentAmmo = 0
local currentAmmoLabel = Player.PlayerGui:WaitForChild("ScreenGui"):WaitForChild("CurrentAmmo")
local maxAmmoLabel = Player.PlayerGui:WaitForChild("ScreenGui"):WaitForChild("MaxAmmo")
local function updateAmmoDisplay()
if currentAmmoLabel and tool ~= nil then
currentAmmoLabel.Parent.Enabled = true
currentAmmoLabel.Text = currentAmmo
local t = currentAmmo / GunSettings[tool.Name].MaxAmmo
currentAmmoLabel.TextColor3 = Color3.new(1, 1, 1):Lerp(Color3.new(1, 0.3, 0.3), 1 - t)
if maxAmmoLabel then
maxAmmoLabel.Text = GunSettings[tool.Name].MaxAmmo
end
end
if tool == nil then
currentAmmoLabel.Parent.Enabled = false
end
end
local function shakeCam(shakeCf)
Camera.CFrame = Camera.CFrame * shakeCf
end
local function shakeCamera(mode)
if mode == "recoil" then
local renderPriority = Enum.RenderPriority.Camera.Value + 1
local camShake = Modules.CameraShaker.new(renderPriority, shakeCam)
camShake:Start()
camShake:Shake(Modules.CameraShaker.Presets.Recoil)
end
end
Character.ChildAdded:Connect(function(Obj)
local IsGun = Remotes.DetectGun:InvokeServer(Obj)
print(IsGun)
if IsGun then
delay(0, function()
if not PlayerAnims[Player] then
PlayerAnims[Player] = {}
end
if not PlayerAnims[Player][Obj.Name] then
PlayerAnims[Player][Obj.Name] = {}
end
for i, v in pairs(script.Guns:GetChildren()) do
if v.Name == Obj.Name then
for index, value in pairs(v.Animations:GetChildren()) do
if not PlayerAnims[Player][Obj.Name][value.Name] then
--local animTrack = Humanoid:LoadAnimation(value)
-- PlayerAnims[Player][Obj.Name][value.Name] = animTrack
end
end
end
end
end)
Services.UserInputService.MouseBehavior = Enum.MouseBehavior.LockCenter
Status.isEquipped = true
tool = Obj
Mouse.Icon = ""
print("Equipped "..Obj.Name)
currentAmmo = Remotes.GetAmmoCount:InvokeServer(Obj.Name)
updateAmmoDisplay()
end
end)
Character.ChildRemoved:Connect(function(Obj)
local IsGun = Remotes.DetectGun:InvokeServer(Obj)
print(IsGun)
if IsGun then
if Obj:WaitForChild("Crosshair") then
local Crosshair = Obj:WaitForChild("Crosshair")
local Front = Crosshair:FindFirstChild("Front")
local Back = Crosshair:FindFirstChild("Back")
delay(0, function()
for i, v in pairs(Front:GetChildren()) do
v.BackgroundTransparency = 1
end
for i, v in pairs(Back:GetChildren()) do
v.BackgroundTransparency = 1
end
end)
end
--PlayerAnims[Player][Obj.Name]["Fire"]:Stop()
--PlayerAnims[Player][Obj.Name]["Aim"]:Stop()
Services.TweenService:Create(Humanoid, Tweens.CameraTweenAim, {["CameraOffset"] = DefaultCameraOffset}):Play()
Services.UserInputService.MouseBehavior = Enum.MouseBehavior.Default
Status.isEquipped = false
Humanoid.AutoRotate = DefaultAutoRotate
tool = nil
Mouse.Icon = DefaultMouseIcon
CameraTweenUnAim:Play()
Sounds.AimUpSound:Play()
print("Unequipped "..Obj.Name)
currentAmmo = 0
updateAmmoDisplay()
end
end)
Services.UserInputService.InputBegan:Connect(function(input)
if input.UserInputType == Enum.UserInputType.MouseButton1 and Status.isEquipped and Status.canShoot and Status.isAiming then
Status.canShoot = false
local FiredGun = Remotes.FireGun:InvokeServer(tool)
if FiredGun then
currentAmmo = currentAmmo - GunSettings[tool.Name]["BulletAmount"]
--PlayerAnims[Player][tool.Name]["Fire"]:Play()
shakeCamera("recoil")
updateAmmoDisplay()
end
task.wait(GunSettings[tool.Name]["FireRate"])
Status.canShoot = true
end
if input.UserInputType == Enum.UserInputType.MouseButton2 and Status.isEquipped then
Status.isAiming = true
if tool:FindFirstChild("Crosshair") then
local Crosshair = tool:WaitForChild("Crosshair")
local Front = Crosshair:FindFirstChild("Front")
local Back = Crosshair:FindFirstChild("Back")
delay(0, function()
for i, v in pairs(Front:GetChildren()) do
Services.TweenService:Create(v, TweenInfo.new(0.65, Enum.EasingStyle.Sine, Enum.EasingDirection.Out, 0, false, 0), {["BackgroundTransparency"] = 0.3}):Play()
end
for i, v in pairs(Back:GetChildren()) do
Services.TweenService:Create(v, TweenInfo.new(0.65, Enum.EasingStyle.Sine, Enum.EasingDirection.Out, 0, false, 0), {["BackgroundTransparency"] = 0.3}):Play()
end
end)
end
Services.TweenService:Create(Humanoid, Tweens.CameraTweenAim, {["CameraOffset"] = Vector3.new(2.5, 0, 0)}):Play()
--PlayerAnims[Player][tool.Name]["Aim"]:Play()
CameraTweenAim:Play()
Humanoid.AutoRotate = false
Sounds.AimDownSound:Play()
end
if input.KeyCode == Enum.KeyCode.R and Status.isEquipped and not Status.isReloading and Status.canShoot then
Status.isReloading = true
Status.canShoot = false
local ReloadedGun = Remotes.ReloadGun:InvokeServer(tool.Name)
task.wait(GunSettings[tool.Name]["ReloadTime"])
if ReloadedGun then
currentAmmo = GunSettings[tool.Name].MaxAmmo
updateAmmoDisplay()
end
Status.canShoot = true
Status.isReloading = false
end
if input.KeyCode == Enum.KeyCode.H and Status.isEquipped then
if tool ~= nil then
if tool:WaitForChild("Handle"):WaitForChild("LaserPoint") then
Status.startedLaser = not Status.startedLaser
local StartLaser = Remotes.StartCloseLaser:InvokeServer(tool, Status.startedLaser)
end
end
end
end)
Services.UserInputService.InputEnded:Connect(function(input)
if input.UserInputType == Enum.UserInputType.MouseButton2 and Status.isEquipped then
Status.isAiming = false
if tool:WaitForChild("Crosshair") then
local Crosshair = tool:WaitForChild("Crosshair")
local Front = Crosshair:FindFirstChild("Front")
local Back = Crosshair:FindFirstChild("Back")
delay(0, function()
for i, v in pairs(Front:GetChildren()) do
Services.TweenService:Create(v, TweenInfo.new(0.4, Enum.EasingStyle.Sine, Enum.EasingDirection.Out, 0, false, 0), {["BackgroundTransparency"] = 1}):Play()
end
for i, v in pairs(Back:GetChildren()) do
Services.TweenService:Create(v, TweenInfo.new(0.4, Enum.EasingStyle.Sine, Enum.EasingDirection.Out, 0, false, 0), {["BackgroundTransparency"] = 1}):Play()
end
end)
end
Services.TweenService:Create(Humanoid, Tweens.CameraTweenUnAim, {["CameraOffset"] = DefaultCameraOffset}):Play()
--PlayerAnims[Player][tool.Name]["Fire"]:Stop()
CameraTweenUnAim:Play()
Humanoid.AutoRotate = DefaultAutoRotate
Sounds.AimUpSound:Play()
end
end)
Services.RunService.RenderStepped:Connect(function()
if Status.isAiming then
local camLookVector = Camera.CFrame.lookVector
local camRotation = math.atan2(-camLookVector.X, -camLookVector.Z)
local Tween = Services.TweenService:Create(Root, Tweens.RootTween, {CFrame = CFrame.new(Root.Position) * CFrame.Angles(0, camRotation, 0)})
Tween:Play()
end
if tool ~= nil then
local muzzle = tool:WaitForChild("Handle"):WaitForChild("Muzzle")
local ray = Ray.new(muzzle.WorldPosition, muzzle.CFrame.LookVector * math.huge)
local hit, position = workspace:FindPartOnRayWithIgnoreList(ray, {Character})
if hit and hit.Parent:FindFirstChildOfClass("Humanoid") then
print("found")
if tool:WaitForChild("Crosshair") then
local Crosshair = tool:WaitForChild("Crosshair")
local Front = Crosshair:FindFirstChild("Front")
local Back = Crosshair:FindFirstChild("Back")
delay(0, function()
for i, v in pairs(Front:GetChildren()) do
Services.TweenService:Create(v, TweenInfo.new(0.4, Enum.EasingStyle.Sine, Enum.EasingDirection.Out, 0, false, 0), {["BackgroundColor3"] = Color3.fromRGB(255, 98, 101)}):Play()
end
for i, v in pairs(Back:GetChildren()) do
Services.TweenService:Create(v, TweenInfo.new(0.4, Enum.EasingStyle.Sine, Enum.EasingDirection.Out, 0, false, 0), {["BackgroundColor3"] = Color3.fromRGB(255, 98, 101)}):Play()
print(v.BackgroundColor3)
end
end)
end
else
local Crosshair = tool:WaitForChild("Crosshair")
local Front = Crosshair:FindFirstChild("Front")
local Back = Crosshair:FindFirstChild("Back")
delay(0, function()
for i, v in pairs(Front:GetChildren()) do
Services.TweenService:Create(v, TweenInfo.new(0.4, Enum.EasingStyle.Sine, Enum.EasingDirection.Out, 0, false, 0), {["BackgroundColor3"] = Color3.fromRGB(255, 255, 255)}):Play()
end
for i, v in pairs(Back:GetChildren()) do
Services.TweenService:Create(v, TweenInfo.new(0.4, Enum.EasingStyle.Sine, Enum.EasingDirection.Out, 0, false, 0), {["BackgroundColor3"] = Color3.fromRGB(255, 255, 255)}):Play()
end
end)
end
end
end)
Remotes.GetMouseHitLocation.OnClientInvoke = function(player)
return Mouse.Hit.Position
end
When I put the print you asked:
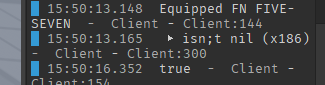