Confused with operators working with table
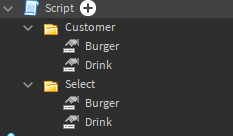
Table1 and Table2 are different tables, so they compare false.
You would need to procedurally check every value to each other to make sure the contents are the same.
As said before, they are different tables: each instance has different information, even if they all have the same properties, to compare 2 tables you can encode them.
local T1, T2 = {}, {}
for _, v in pairs(Customer:GetChildren()) do
table.insert(T1, v.Name)
end
for _, v in pairs(Select:GetChildren()) do
table.insert(T2, v.Name)
end
function Encode(Table)
local Service = game:GetService("HttpService")
local Success, Text = pcall(Service.JSONEncode, Service, Table)
if not Success then warn(Text) return end
return Text
end
print(Encode(T1) == Encode(T2))
JSONEncode
does not accept instances.
table.concat will produce a string that could be compared to another table, but this could be tricked by the contents of the table.
While converting tables to strings for comparisons works, I’d personally recommend against doing that and writing a general case table comparison function, or a custom one tailored to your data if you intend on using it a lot.
String creation is a pretty heavy task that involves one, if not several memory allocations to make room to store the string under the hood. Allocations are incredibly expensive compared to other tasks, so you could easily see slow downs in code that requires using it a lot.
If you just need it every once in a while you’re fine using the other methods, but I’d still recommend keeping in mind that it is slow if you ever change your use cases for it in the future.
If you’re interested in the details about why:
The algorithm also has to search through the whole table and manually convert the data to string data in a sensible way for every entry in the table. Then when you do comparisons with the strings that comparison could at best be nearly instant where the comparison detects a difference early, and at worst you need to compare every character in the string to confirm they are in fact the same string.
Memory allocations, in short, are where the program asks for more memory to store data. You could compare it to making a new file on disk to write data. Not quite the same thing, but pretty similar concept.
The slow part is that the part of the program doing the allocation has to make sure it owns enough memory to give some to you to use. If it doesn’t, it needs to ask the operating system for some more. Once it knows it has enough it has to search through the memory it owns to find a good spot to lend out to you for use.
When you make a new string you need somewhere to store all the characters, so you need to allocate enough space to store the whole thing. If the string creation guesses the wrong amount to allocate the first time then it needs to allocate a larger amount and copy all of the current work into the new string memory to continue.
That’s quite a lot of extra work required just to compare two tables.