This is what I am trying to accomplish:
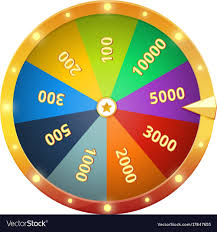
This is what I got so far:
I have the circle part, which has a SurfaceGui on it, with an Image (the image being the white circle you see)
What I am trying to figure out is how to go make frames like the reference image above. I don’t want a preset image, as I’d like to be able to add more sections to the wheel in the future, or be able to change the prizes on it easily. Is there a way to create the ‘pizza slice’ shapes using the Slicing? Or would I be best of trying to find a pizza slice image, and just using that? Which would bring up more questions as to how to actually position the parts around the wheel properly (which I’m guessing will require some crazy math formula 
Any advice or tips is much appreciated 
2 Likes
Based on what your question asked (after reading it more carefully), what you’d need to do is create a variety of different sized slices (different size in the arc length, preferably in an even number of degrees to keep things easy).
The “slice” images need to be entirely white and uploaded as if they were still in the circle, i.e.
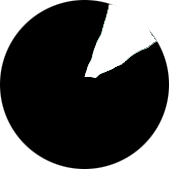
For readability the circle was made black, though you should also crop out the black part as well.
Then, if you know the length of each slice, you simple do something like:
--the arc length of each slice picture you upload
local sliceLengths = {10, 15, 20, 25, 30, 45, 60}
--{angle the slice begins at, angle the slice ends at, type of slice}
local sliceAngles = {}
local atAngle = 0
while atAngle < 360 do
local chosenSliceLength = sliceLengths[math.random(1, #sliceLengths)]
----create/clone imagelabel of the correct slice, the variable can be called "img"
img.Rotation = atAngle
atAngle = atAngle + chosenSliceLength
table.insert(sliceAngles, {atAngle, ((chosenSliceLength <= 360 and chosenSliceLength) or 360), "Common/Rare/Etc"})
end
Layer the images correctly to where the first image’s ZIndex is 1 greater than the last and you won’t have to make the slices fit into 360 degrees.
2 Likes
The only way to “Slice” GUI objects is using ClipsDescendants
, which doesn’t work with rotated objects for whatever reason. Furthermore, objects always rotate at their center, not their AnchorPoint, so you’d either have to do some complicated math, or upload images that have the rotational point as their center.
The easiest way to do this is to upload all slices you’re going to use, or always full circles you’re going to use. It seems inefficient, but is much faster than trying to come up with a good solution using only scripts.
1 Like
You can upload one slice. That slice would then be cloned and positioned/rotated inside the script.
You can try something like this:
local ANGLE = 15 --> Totally dependant on your_slice_object!
local RADIUS = 40 --> Radius in pixels, should be radius of your circle object.
local slice = your_slice_object
local n = 2*math.pi*RADIUS / ANGLE --> Number of slices.
for i=1, n do
local angle = i * ANGLE
local x = math.cos(angle) * RADIUS
local y = math.sin(angle) * RADIUS
local position = UDim2.new(0, x, 0, y)
local newSlice = slice:Clone()
newSlice.Position = Position
newSlice.Rotation = Rotation
...
end
5 Likes