Hello!
I’m a beginner and not very knowledgeable in Roblox scripting, so of course I’ve run into another problem with a script without any errors in the output or pointers for what I need to fix. I’ve looked all over, but couldn’t find any solutions. So here I am.
Basically, for some context:
I’m working on a singleplayer game where you play as a self-aware NPC that found themselves in a post-death purgatory for NPCs. The game starts with your character being very surprised that they’re suddenly conscious and able to think for themselves, and they realize they need to find a way out of the purgatory. After their shock is over, an invisible wall preventing the player from proceeding will lose collision, allowing them to walk through and start the game. Once this happens, a RemoteEvent located in the ReplicatedStorage called “IntroStart” is fired for the client, and then a localscript inside a credits GUI will activate once it recognizes this, and then it’ll tween the transparency of the text and UIstroke inside of it multiple times, as well as changing the text. The code looks like this:
local rs = game.ReplicatedStorage
local ts = game.TweenService
local text = script.Parent.TextLabel
local stroke = text.UIStroke
text.Transparency = 1
stroke.Transparency = 1
rs:WaitForChild("IntroStart").OnClientEvent:Connect(function()
wait(10)
--Developed/Written
text.Text = [[Developed and Written
by Murky]]
local tweentext = ts:Create(text,TweenInfo.new(3,Enum.EasingStyle.Quad,Enum.EasingDirection.InOut,0,false,0),{Transparency = 0})
tweentext:Play()
local tweenstroke = ts:Create(stroke,TweenInfo.new(3,Enum.EasingStyle.Quad,Enum.EasingDirection.InOut,0,false,0),{Transparency = 0.65})
tweenstroke:Play()
wait(5)
tweentext = ts:Create(text,TweenInfo.new(3,Enum.EasingStyle.Quad,Enum.EasingDirection.InOut,0,false,0),{Transparency = 1})
tweentext:Play()
tweenstroke = ts:Create(stroke,TweenInfo.new(3,Enum.EasingStyle.Quad,Enum.EasingDirection.InOut,0,false,0),{Transparency = 1})
tweenstroke:Play()
wait(5)
--Music Sourced
text.Text = [[Music sourced from
the Roblox Library]]
tweentext = ts:Create(text,TweenInfo.new(3,Enum.EasingStyle.Quad,Enum.EasingDirection.InOut,0,false,0),{Transparency = 0})
tweentext:Play()
tweenstroke = ts:Create(stroke,TweenInfo.new(3,Enum.EasingStyle.Quad,Enum.EasingDirection.InOut,0,false,0),{Transparency = 0.65})
tweenstroke:Play()
wait(5)
tweentext = ts:Create(text,TweenInfo.new(3,Enum.EasingStyle.Quad,Enum.EasingDirection.InOut,0,false,0),{Transparency = 1})
tweentext:Play()
tweenstroke = ts:Create(stroke,TweenInfo.new(3,Enum.EasingStyle.Quad,Enum.EasingDirection.InOut,0,false,0),{Transparency = 1})
tweenstroke:Play()
wait(5)
--An Experience
text.Text = [[23
An experience]]
tweentext = ts:Create(text,TweenInfo.new(3,Enum.EasingStyle.Quad,Enum.EasingDirection.InOut,0,false,0),{Transparency = 0})
tweentext:Play()
tweenstroke = ts:Create(stroke,TweenInfo.new(3,Enum.EasingStyle.Quad,Enum.EasingDirection.InOut,0,false,0),{Transparency = 0.65})
tweenstroke:Play()
wait(5)
tweentext = ts:Create(text,TweenInfo.new(3,Enum.EasingStyle.Quad,Enum.EasingDirection.InOut,0,false,0),{Transparency = 1})
tweentext:Play()
tweenstroke = ts:Create(stroke,TweenInfo.new(3,Enum.EasingStyle.Quad,Enum.EasingDirection.InOut,0,false,0),{Transparency = 1})
tweenstroke:Play()
wait(5)
end)
Now I know it’s messy, a bit hard to read, and probably an inefficient solution, but I’m just trying to create something that works for now. As far as I can tell, there’s no reason for this code not to work.
For some more context, I’ll show some explorer screenshots and what the serverscript that executes the remote event looks like - I’m sure you can tell which is which.
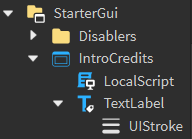
Any and all help is seriously appreciated, cause I’ve been stumped on this since yesterday. Thank you!