Recently, me and my friend have been trying to make a script that creates a textlabel on a players screen when they are holding a tool. We are trying to make the text appear once the player holds the item, and only disappear once the player dies/leaves the game. We thought we could do this by enabling and disabling a screengui, but it doesn’t seem to work. Does anyone have a possible solution to this?
Here is our current script:
local StarterGui = game:GetService("StarterGui")
local item = script.Parent
local player = game.Players.LocalPlayer
local screenGui = StarterGui:FindFirstChild("UsedNoclip")
local textLabel = screenGui:FindFirstChild("NoclipText")
screenGui.Enabled = false -- Hide the TextLabel initially
local hasBeenHeld = false -- Flag to track if the item has been held
-- Function to check if the item is being held
local function checkHold()
local humanoid = item.Parent:FindFirstChildOfClass("Humanoid")
-- Check if the item is being held by a character and hasn't been held before
if humanoid and humanoid:IsA("Humanoid") and humanoid:GetState() == Enum.HumanoidStateType.Seated and not hasBeenHeld then
-- The item is held for the first time, show the text label
screenGui.Enabled = true
hasBeenHeld = true
end
end
-- Connect the checkHold function to the Heartbeat event
item.Parent:WaitForChild("Humanoid").Heartbeat:Connect(checkHold)
-- Function to check if the player dies and reset the flag
local function onDeath()
hasBeenHeld = false
screenGui.Enabled = false
end
-- Connect the onDeath function to the Died event
item.Parent:WaitForChild("Humanoid").Died:Connect(onDeath)
11 Likes
The ScreenGUI you’re likely referring to is not from the PlayerGUI, but instead in the StarterGUI. Maybe you can change it to
local screenGui = player.PlayerGui:FindFirstChild("UsedNoclip")
1 Like
I just tried this, and it doesn’t seem to be doing anything 
Is a LocalScript a child of ScreenGui, Or can you screenshot where is LocalScript/Script located?
It’s possible you are trying to change the label inside of the “StarterGui” Note, when a player is in-game, their GUI is not inside of StarterGui. Rather, it is inside of their player object in game.Players.
If this is in a local script, you can start by replacing StarterGui with the PlayerGui, where the player’s gui is located.
you can do this by finding the localplayer then finding the playergui inside of the localplayer.
local localplayer = game.Players.LocalPlayer
local playergui = localplayer.PlayerGui
You should be able to treat the “player gui” the same as you treated the starter gui in your script.
1 Like
For reference, whenever you are in-game, disabling anything in the StarterGui will have no difference, however disabling things in the PlayerGui does make a difference.
2 Likes
The LocalScript is a child of the tool inside of the StarterPack.
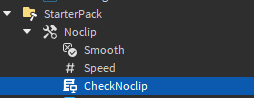
1 Like
Ok, that makes a lot more sense. Now we have the playergui working, but we think something on lines 15 - 24 (the function) is preventing it from appearing.
Also instead of:
local humanoid = item.Parent:FindFirstChildOfClass("Humanoid")
would it be better to change it as:
local humanoid = player:FindFirstChild("Humanoid")
?
The item.Parent would be the StarterPack, so that wouldn’t work.
It depends on what “player” means. If it’s the character, then yes, that could work. However the other option works as well, it might just be less efficient.
If the “player” is the actual player object in the player service, try player.Character:FindFirstChild(“Humanoid”) instead.
When doing that I get an error saying “Incomplete statement: expected assignment or a function call.” 
Just give me a minute, Wait please
I have a question, is your

screenGui is an actual ScreenGui object? Or is it a TextLabel? If it is a TextLabel, you should do
TextLabel.Visible = false
rather than setting .Enabled = false
because that property doesn’t exist in a TextLabel
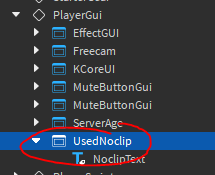
yes, screenGui is a PlayerGui
So you just use
local screenGui = StarterGui:FindFirstChild("UsedNoclip")
with StarterGui instead of PlayerGui
StarterGui wouldn’t work because it’s a gui for the player, and not players as SinkyProot mentioned earlier
Does this work? Difficult to test without the actual tools themselves, I am trying to solve this without them.
local item = script.Parent
local player = game.Players.LocalPlayer
local screenGui = player:WaitForChild("PlayerGui"):FindFirstChild("UsedNoclip")
local textLabel = screenGui:FindFirstChild("NoclipText")
screenGui.Enabled = false -- Hide the ScreenGui initially
local hasBeenHeld = false -- Flag to track if the item has been held
-- Function to check if the item is being held
local function checkHold()
local humanoid = item.Parent:FindFirstChildOfClass("Humanoid")
-- Check if the item is being held by a character and hasn't been held before
if humanoid and humanoid:GetState() == Enum.HumanoidStateType.Seated and hasBeenHeld == false then
-- The item is held for the first time, show the text label
screenGui.Enabled = true
hasBeenHeld = true
end
end
-- Connect the checkHold function to the Heartbeat event
item.Parent:WaitForChild("Humanoid").Heartbeat:Connect(checkHold)
-- Function to check if the player dies and reset the flag
local function onDeath()
hasBeenHeld = false
screenGui.Enabled = false
end
-- Connect the onDeath function to the Died event
item.Parent:WaitForChild("Humanoid").Died:Connect(onDeath)
Also, which line has this error?
So that’s mean you have 2 kind of ScreenGui named “UsedNoclip”?
This doesn’t seem to work either. I’m also not getting any errors which is kinda weird. Just making sure, the ScreenGui is supposed to be in StarterGui where it gets cloned into PlayerGui, correct?