Hello everyone, I’m back. As of my last post, I’ve finally managed to make the folder work by the use of module scripts, and so far it’s been working fine.
Now, I’ve come across an issue while porting the Pathfinding code. Let me lay out what happens:
- the enemy spawns in, and creates a path to its goal. It then follows it normally.
- the enemy is coded to drop the path and attempt to kill a player if it spots one within its vision range, so when I place a test dummy near it, it drops the path and proceeds forward.
- once the dummy is out of sight or dead, it then creates a new path towards its goal, like it should.
- however, instead of following its new path, it always goes back to the last waypoint it itself off in from the oldest path and continues following the old path.
Here’s a visual representation of what I mean:
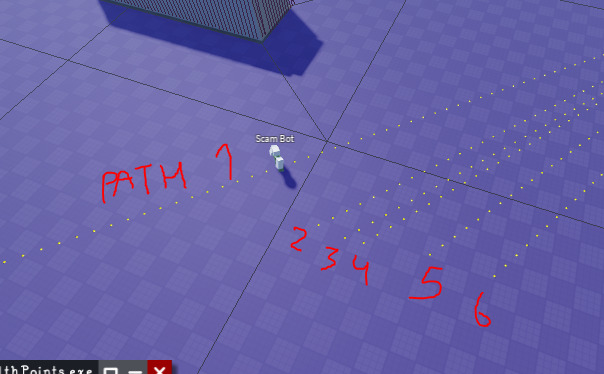
Instead of following paths 2-6, it instead always follows Path 1. There’s also an interesting issue, in which whenever I try to print the path’s waypoints, it returns not one, but all previously calculated paths:
19:35:43.021 Current waypoint: 14 Total waypoints: 54 - Server - Pathfinding:67
19:35:43.021 Current waypoint: 34 Total waypoints: 78 - Server - Pathfinding:67
…and since it checks the oldest path’s waypoints last, I assume it then follows the oldest path due to such. The path itself is always created when the code is told to compute it, and it’s supposed to run itself based on the newly computed path. I don’t know why any of this is happening, since it worked fine when it was just a normal Server Script…
Any ideas? I’d love to find a solution to this. Any help appreciated!
Can you show the scripts because it might be the way you made the pathfinding bit
The code was taken from an example script I found somewhere in the DevForum. I intend on rewriting it in my own way once I get all the porting done, but for now, I’ve got this:
This does the path computing.
local function ComputePath(Character, startGoal:Vector3, endGoal:Vector3)
local HumRP = Character.HumanoidRootPart
local Humanoid = Character.Humanoid
local RetriesLeft = 30 -- 30 retries
local RetryInterval = .1 -- delay .1 seconds each time it fails
for i = RetriesLeft, 1, -1 do
local Path = PathfindingService:CreatePath({
AgentCanJump = true,
AgentCanClimb = true,
WaypointSpacing = 2.75,
Costs = {
Dangerous = math.huge
}
})
Path:ComputeAsync(startGoal, endGoal)
if Path.Status == Enum.PathStatus.Success then
return Path
else
task.wait(RetryInterval)
end
end
warn("Path failed to compute.") -- this will be ran when the for loop is finished, or when all the retries failed.
while true do -- if pathfinder fails to compute, force the enemy to go to the terminal
local Magnitude = (HumRP.CFrame.p - EndPoint.CFrame.p).Magnitude
if Magnitude <= 25 then -- if the enemy manages to get near the terminal, forcefully end the loop
Humanoid:MoveTo(HumRP.CFrame.p)
end
Humanoid:MoveTo(EndPoint.CFrame.p)
task.wait(.1)
end
end
This part does the actual pathfinding movement.
local function WalkHumanoid(endGoal:Vector3)
local humanoid = Character.Humanoid
local HumRP = Character.HumanoidRootPart
local Path = ComputePath(Character, HumRP.Position, endGoal)
print(Path)
-- setting up path properties
local Waypoints = Path:GetWaypoints()
local CurrentWaypointIndex = 2 -- the point to go after the first point
-- setup connections
local MovedToFinishConnection:RBXScriptConnection
local PathBlockedConnection:RBXScriptConnection
MovedToFinishConnection = humanoid.MoveToFinished:Connect(function(reached)
if reached then -- if the humanoid reached the waypoint within 8 seconds
if CurrentWaypointIndex < #Waypoints then -- if we have not cycle through the last waypoint
CurrentWaypointIndex += 1 -- we increase the index by 1 so that the humanoid moves to the next waypoint
humanoid:MoveTo(Waypoints[CurrentWaypointIndex].Position) -- and the same thing
if Waypoints[CurrentWaypointIndex].Action == Enum.PathWaypointAction.Jump then
humanoid.Jump = true
end
else -- we cycled the whole path series!
print("Path reached!")
MovedToFinishConnection:Disconnect() -- disconnect the unnecessary event to avoid memory leaks
end
else -- failed to reach waypoint within 8 seconds, the dummy probably is stuck, so let's recompute it!
MovedToFinishConnection:Disconnect() -- disconnect the unnecessary event to avoid memory leaks
WalkHumanoid(humanoid, HumRP.Position, endGoal) -- we are changing our start goal and replacing it to the current position of our dummy instead of reusing the old one
end
end)
PathBlockedConnection = Path.Blocked:Connect(function(blockedWaypointIndex)
warn(blockedWaypointIndex, CurrentWaypointIndex)
if blockedWaypointIndex >= CurrentWaypointIndex then -- a waypoint ahead of us is blocked! recompute the path
-- disconnect the events
MovedToFinishConnection:Disconnect()
PathBlockedConnection:Disconnect()
humanoid.WalkToPoint = HumRP.Position -- make our humanoid stop walking at its current position and cancel it's movement to the next waypoint
WalkHumanoid(humanoid, HumRP.Position, endGoal)
end
end)
-- make the humanoid walk
humanoid:MoveTo(Waypoints[CurrentWaypointIndex].Position)
if Waypoints[CurrentWaypointIndex].Action == Enum.PathWaypointAction.Jump then
humanoid.Jump = true
end
end
Again, this code is from a DevForum post, and I have yet to rewrite it on my own. It worked fine until I had to port it to a ModuleScript, then it all kinda just fell apart there for some reason.