I was working on optimizing my hotbar system, but while doing so I came across a bug. The function removeItem()
has one param, an array. Most of the time it looks something like this:
{
{
"testItemOne", --item name
1 --amount of the item
},
{
"testItemTwo",
1
}
}
(extra details:)
If I were to put this in an i,v in [array]
loop and print v every iteration, I should see this:
{
"testItemOne"
1
}
{
"testItemTwo",
1
}
Instead, I would see this:
{
"testItemOne"
1
}
{
"testItemOne",
1
}
But, when I loop through it, it keeps giving me the first item of the array, for every iteration. Here is my code:
function removeItem(items: Array)
for h,m in pairs(items) do
local itemID = m[1]
local count = m[2]
print(itemID, count)
local returns = false
for i,v in game.Players.LocalPlayer.hotbar:GetChildren() do
if v:GetAttribute("id") == itemID and v.Value >= count then
game.ReplicatedStorage.events.setVal:FireServer(v, v.Value - count)
task.wait(0.1)
if v.Value <= 0 then
game.ReplicatedStorage.events.setName:FireServer(v, "")
--game.ReplicatedStorage.events.setIMG:FireServer(v.Parent.icon, "")
end
returns = true
break
end
end
return returns
end
end
The console output:
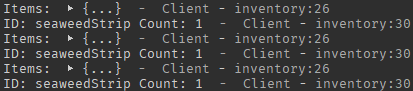
why not structure your dictionary like this:
{
id= “testItemOne”,
count = 1
}
Then just access like this:
local itemID = m[“id”]
local count = m[“count”]
i already coded everything around this format, so it just makes it easier to go along with it
Honestly just put a breakpoint and add some watches. Doing that would make it trivial to solve
In the long run, this is probably a better structure to replicate.
Nevertheless, to the OP, I modified your code a bit but can’t verify if it works at the moment.
function removeItem(items: Array)
for h,m in pairs(items) do
local itemID = m[1]
local count = m[2]
print(itemID, count)
local returns = false
for i,v in game.Players.LocalPlayer.hotbar:GetChildren() do
if v:GetAttribute("id") == itemID and v.Value >= count then
game.ReplicatedStorage.events.setVal:FireServer(v, v.Value - count)
task.wait(0.1)
if v.Value <= 0 then
game.ReplicatedStorage.events.setName:FireServer(v, "")
--game.ReplicatedStorage.events.setIMG:FireServer(v.Parent.icon, "")
end
returns = true
break
end
end
if not returns then
return false
end
end
return true
end
I’m suspecting the issue lies in the return returns
line of your original code, but I could be way off. Try inserting some print()
statements throughout the original code to help single out the problem.
2 Likes
That definitely helped, thank you! But, for some reason it fires three times, so I’m troubleshooting that right now.
1 Like