Although the title says that my function isn’t killing the player, a bunch of things in it aren’t working. First I’ll show you the function:
local Object = game.Workspace.Orca
local debounce = false
local function killPlayer(otherPart)
if debounce == false then
debounce = true
touching = true
wait(0.5)
local ObjectPosition = Object.PrimaryPart.Position
local partParent = otherPart.Parent
local humanoid = partParent:FindFirstChild("Humanoid")
Object.PrimaryPart.Position += Vector3.new(0, 10, 0)
if humanoid then
humanoid.Health = 0
end
wait(1)
Object.PrimaryPart.Position = ObjectPosition
wait()
touching = false
debounce = false
end
end
script.Parent.Touched:Connect(killPlayer)
touching
doesn’t really matter in this. Orca is a model and this is what it looks like:
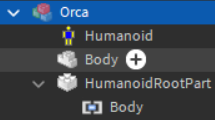
What I’m trying to do is make the orca go down underwater and back up. The PrimaryPart of the Orca is HumanoidRootPart and it moves down and up but the Body only moves down but not back up. Also, Humanoid.health doesn’t kill you unless you move around a bit.
Sorry if that was confusing but any help would be greatly appreciated!
make sure the code is on server
local killed = false;
script.Parent.Touched:Connect(function(hit)
if killed then
return;
end;
local parent = hit.Parent;
local humanoid = parent:FindFirstChild'Humanoid';
if humanoid then killed = true;
local oldCF = Object:GetPrimaryPartCFrame();
Object:PivotTo(oldCF * CFrame.new(0,10,0));
humanoid:TakeDamage(humanoid.Health);
task.delay(1, function()
Object:PivotTo(oldCF);
killed = false;
end);
end;
end);
Yeah, it’s a server script, it looks like your code is working though sometimes the humanoid doesn’t take damage, do you know the cause of this?
Also, I wondered if you could do a debounce of 5 seconds for each player since they can still activate it when they’re lying in pieces on top of the part.
It still sometimes doesn’t take damage, do you know what I need to do to fix this?
I believe that other parts can activate your function, so make sure you use the debounce after you detected the humanoid and not before.
I have a near-exact copy of @WhitexHat’s code and there’s no debounce in his code so that’s not it. 
Also, (off-topic) is your font on the devforum “normal”; as in the opposite of the bold font it usually is?
How many times does it do damage? Also yes, my font changed.
If you touch it there’s about a 75% chance you’ll take damage, if not then if you move around a bit then you’ll die.
I’m not really sure why that happens… It sounds weird!
script.Parent.Touched:Connect(function(hit)
if killed then
print('Debounce - cancel')
return;
end;
Try to see if this prints while you’re unable to take damage. If you’re saying there’s a chance that you won’t take damage sometimes, then to me it sounds like either the problem inlays with collision to the part itself or you’re trying to take damage while the debounce is still stalling.
According to the code written a few posts above, there’s a 1 second task delay in which the ‘killed’ variable acts as a debounce.
Maybe you can do this :
local Object = game.Workspace.Orca
local debounce = false
local function killPlayer(otherPart)
if debounce == false then
debounce = true
touching = true
wait(0.5)
local ObjectPosition = Object.PrimaryPart.Position
local partParent = otherPart.Parent
local humanoid = partParent:FindFirstChild("Humanoid")
Object.PrimaryPart.Position += Vector3.new(0, 10, 0)
if humanoid then
humanoid:TakeDamage(humanoid.MaxHealth)
end
wait(1)
Object.PrimaryPart.Position = ObjectPosition
wait()
touching = false
debounce = false
end
end
script.Parent.Touched:Connect(killPlayer)
check your parts and see if they have CanTouch property enabled
Wait…
Possible problem 1: The orca could be activating it because CanTouch is set to true in all its parts. And it has a Humanoid.
Possible problem 2: It might be that if killed is equal to true, then it stops other people from dying for 1 second. Now that I think about it, it only stopped working when multiple people touched it at the same time. - I think this is actually the problem
Sorry for not realising these things before.
I can’t tell what the problem is, it should be working
add me on discord so I can help you out endriu#0001