So I am making a tower defense game and I came across this problem.
The zombie I am using will not go to the correct location for some reason? It goes through a for loop that goes through the waypoints. It uses the number to name it and that works just fine, but the problem is that it instantly moves towards the second waypoint, why does it do this?
Script inside the zombie:
local human = script.Parent.Humanoid
local waypoints = script.Parent.Parent.Parent.Waypoints
for point = 1, #waypoints:GetChildren() do
human:MoveTo(waypoints[point].Position)
human.MoveToFinished:Wait()
end
script.Parent:Destroy()
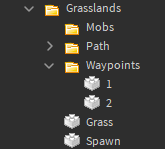
The waypoints are where the zombie moves. And the mobs folder is where the zombie is located.
This is the prototype map I made. The red parts are waypoints. THEY ARE NAMED CORRECTLY.
The zombie is moving to the second waypoint.
https://i.gyazo.com/e4707c1fff853335d6af39a91517fb0c
Tried naming them by maybe “A” or “B”?
Always could try using ipairs
too since it sorts through the array returned in order.
Still does the same thing the number names do. It just ignores the first one.
Something like this would return the array in numeric order,
for i, v in ipairs(game.Workspace.Folder:GetChildren()) do
print(v.Name)
end
Output:

Like, I said you could probably make ipairs
work.
Completely randomized names, and it still is returned in the order it is in the folder.
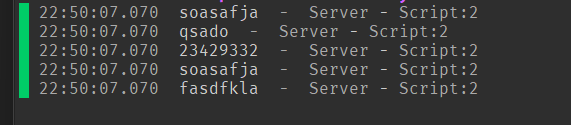
Try this,
local human = script.Parent.Humanoid
local waypoints = script.Parent.Parent.Parent.Waypoints:GetChildren()
for i, v in ipairs (waypoints) do
human:MoveTo(waypoints[v].Position)
human.MoveToFinished:Wait()
end
script.Parent:Destroy()
I also used ipairs
, it just skips 1 for some reason.
Also that script wouldn’t work, “v” is the part, indexing it to the parent makes no sense.
No, v
is the part found in the folder, but it doesn’t matter anyways if ipairs
didn’t work for you.
I even made it so it destroys the part when traveled to, and it instantly destroyed 1 and 2 completely skipping 1.
That is what I said, please listen.
I’m pretty sure it is actually doing it in the correct order just always skipping the first part? Which is odd, tried renaming them to like, “2”, and “3”, or is it always the first one.
Always the first one. For some reason.
Have you tried three or more parts?
Yes. There is currently 8 parts.
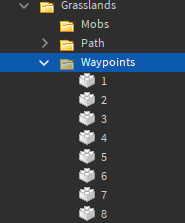
It routes to each one in order skipping 1?
Okay, so I figured out something weird. It destroys the 1st part no problem, but does not move to it, but the other parts are moved to just fine.
Change point to = 0,
local human = script.Parent.Humanoid
local waypoints = script.Parent.Parent.Parent.Waypoints
for point = 0, #waypoints:GetChildren() do
human:MoveTo(waypoints[point].Position)
human.MoveToFinished:Wait()
end
script.Parent:Destroy()
Try that.
I’m not sure if you can start point at 0, so. but worth a shot, what you said is really funny and that shouldn’t just be skipping 1 at all.
It does not work, unfortunately.
The zombie does everything but walk to the 1st part, it does acknowledge it but doesn’t walk to it.