okay maybe what would help is giving u more information
local plr = game.Players.LocalPlayer
local UIS = game:GetService("UserInputService")
local TweenService = game:GetService("TweenService")
local StarterGui = game:GetService("StarterGui")
StarterGui:SetCoreGuiEnabled(Enum.CoreGuiType.PlayerList, false)
local GameGUI = plr.PlayerGui:WaitForChild("GameGUI")
local PickaxeGUI = plr.PlayerGui.PickaxeGUI
local Pickaxe = PickaxeGUI.Pickaxe
local MP = 0 --MinePoints
local TMP = 0 --Total MinePoints
local tmpLabel = GameGUI.TotalMP.TotalMP
local mpLabel = GameGUI.MP.MP
mpLabel.Text = MP .. " MP"
tmpLabel.Text = TMP .. " Total MP"
local mineCDtime = 0.1
local mineCD = 0
local Materials = GameGUI.Materials:GetChildren()
local Mineables = GameGUI.Mineables:GetChildren()
print(Mineables)
GameGUI.Mineables.Grass.Visible = true
local previousframe
local tabel = {}
UIS.InputBegan:Connect(function(input)
if input.UserInputType == Enum.UserInputType.MouseButton1 and mineCD <= tick() then
mineCD = tick() + mineCDtime
TMP = TMP+1
MP = MP+1
mpLabel.Text = MP .. " MP"
tmpLabel.Text = TMP .. " Total MP"
local tweenInfoGo = TweenInfo.new(
0.03,
Enum.EasingStyle.Linear,
Enum.EasingDirection.Out,
0,
false,
0
)
local tweenInfoBack = TweenInfo.new(
0.06,
Enum.EasingStyle.Linear,
Enum.EasingDirection.Out,
0,
false,
0
)
local tweenInfoSTARTPOS = TweenInfo.new(
0.03,
Enum.EasingStyle.Linear,
Enum.EasingDirection.Out,
0,
false,
0
)
local TweenGo = TweenService:Create(Pickaxe, tweenInfoGo, {Rotation = 5})
TweenGo:Play()
wait(0.03)
local TweenBack = TweenService:Create(Pickaxe, tweenInfoBack, {Rotation = -5})
TweenBack:Play()
wait(0.06)
local TweenSTART = TweenService:Create(Pickaxe, tweenInfoSTARTPOS, {Rotation = 0})
TweenSTART:Play()
print(tabel)
print(previousframe)
if previousframe then
previousframe.Visible = false
previousframe = nil
end
local n = math.random(1,3)
for i,v in Mineables do
if n == i then
Mineables[i].Visible = true
previousframe = Mineables[i]
end
end
end
end)
this is the localscript thats in StarterPlayer
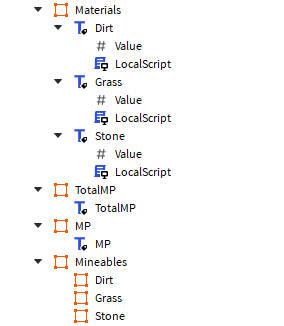
and this screenshot again
My issue here is that when i click, it shows me 1 of the 3 frames which are, dirt,grass, or stone, for example if it shows stone, and i click again, i want it to add 1 to my stones

but the problem im having is, i cant make it so that it will add +1 to the stone when i click on the stone frame that shows, just putting it in for i,v in Mineables do
will add 3, because it loops through 3 different frames
i hope this helps 