For some reason the head disappears after tweening. IDK why it is like this, also there are no errors. Here’s the script:
local CurrentCam = game.Workspace.CurrentCamera
local JumpscareEvent = game.ReplicatedStorage.GameEvents.Jumpscare
local JumpscareCam = game.Workspace.Jumpscare.Camera
local Monster = game.Workspace.Jumpscare.Freddy
local meshPart = Monster.Head
local TS = game:GetService("TweenService")
local Anim = Monster:WaitForChild("Humanoid").Animation
local AnimTrack = Monster:WaitForChild("Humanoid"):LoadAnimation(Anim)
local SFX = game.Workspace.SFX
local targetPosition = Vector3.new(-20.38, 10.039, -1.149)
local targetRotation = Vector3.new(-18, 0, 0)
local function moveAndRotate()
-- Create a new tween for position
local positionTween = TS:Create(
meshPart,
TweenInfo.new(0.4), -- Duration of the tween (2 seconds in this case)
{ Position = targetPosition }
)
-- Create a new tween for rotation
local rotationTween = TS:Create(
meshPart,
TweenInfo.new(0.2), -- Duration of the tween (2 seconds in this case)
{ Orientation = targetRotation }
)
-- Play the position tween first
positionTween:Play()
-- Connect a function to play the rotation tween after the position tween is complete
positionTween.Completed:Connect(function()
rotationTween:Play()
end)
end
JumpscareEvent.OnClientEvent:Connect(function()
CurrentCam.CameraType = Enum.CameraType.Scriptable
CurrentCam.CFrame = JumpscareCam.CFrame
SFX["FNaF 6 Salvage Jumpscare"]:Play()
moveAndRotate()
end)
The following files attached are either related to the heirarchy or the bug itself.
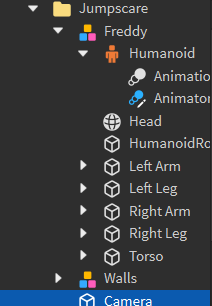