I have this script where when you take damage or gain health it say + or - however much you got but there is a problem, the health stacks for example when i eat a fruit that has 3 clicks it says +5 +10 +15 and when i take damage its the same
local humanoid = character:WaitForChild("Humanoid")
local indicatorFrame = script.Parent -- Reference to the Frame for indicators
local previousHealth = humanoid.Health
local maxIndicators = 4 -- Maximum number of indicators on screen
local indicatorList = {} -- Store indicator instances
local cooldown = false
local cooldownTime = 2 -- Cooldown time in seconds
local function createIndicator(amount, isDamage)
local indicator = Instance.new("TextLabel")
indicator.Size = UDim2.new(0, 100, 0, 20)
indicator.Position = UDim2.new(0, math.random(0, indicatorFrame.AbsoluteSize.X - 100), 0, math.random(0, indicatorFrame.AbsoluteSize.Y - 20))
indicator.BackgroundTransparency = 1
indicator.TextScaled = true
indicator.Text = (isDamage and "-" or "+") .. tostring(amount)
indicator.TextColor3 = isDamage and Color3.fromRGB(255, 0, 0) or Color3.fromRGB(0, 255, 0) -- Red or Green color
indicator.Font = Enum.Font.LuckiestGuy
indicator.TextStrokeTransparency = 0 -- Set text stroke transparency to 0
indicator.Parent = indicatorFrame
table.insert(indicatorList, indicator)
wait(2) -- Display the indicator for 2 seconds
indicator:Destroy()
table.remove(indicatorList, 1) -- Remove the indicator from the list
end
local function onHealthChanged()
local healthDifference = humanoid.Health - previousHealth
if healthDifference < 0 then
print("Creating damage indicator:", math.abs(healthDifference))
local numIndicators = math.ceil(math.abs(healthDifference) / 5) -- Calculate the number of damage indicators to display
for i = 1, numIndicators do
createIndicator(5, true) -- Display damage indicators
end
elseif healthDifference > 0 then
if not cooldown then
local numIndicators = math.ceil(healthDifference / 5) -- Calculate the number of healing indicators to display
print("Creating", numIndicators, "healing indicator(s)")
for i = 1, numIndicators do
createIndicator(5, false) -- Display healing indicators
end
cooldown = true
wait(cooldownTime)
cooldown = false
else
wait(cooldownTime) -- Wait for the existing cooldown
end
end
previousHealth = humanoid.Health
end
humanoid.HealthChanged:Connect(onHealthChanged)
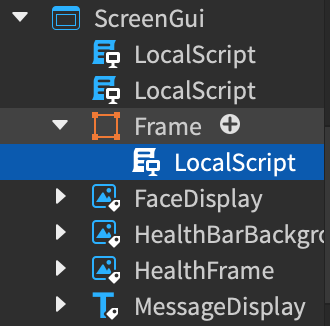
Hello @DemonicPandazYT! 
I tried to fix your local script, and here it is!
local humanoid = character:WaitForChild("Humanoid")
local indicatorFrame = script.Parent
local previousHealth = humanoid.Health
local maxIndicators = 4
local indicatorList = {}
local cooldown = false
local cooldownTime = 2
local function createIndicator(amount, isDamage)
local indicator = Instance.new("TextLabel")
indicator.Size = UDim2.new(0, 100, 0, 20)
indicator.Position = UDim2.new(0, math.random(0, indicatorFrame.AbsoluteSize.X - 100), 0, math.random(0, indicatorFrame.AbsoluteSize.Y - 20))
indicator.BackgroundTransparency = 1
indicator.TextScaled = true
indicator.Text = (isDamage and "-" or "+") .. tostring(amount)
indicator.TextColor3 = isDamage and Color3.fromRGB(255, 0, 0) or Color3.fromRGB(0, 255, 0)
indicator.Font = Enum.Font.LuckiestGuy
indicator.TextStrokeTransparency = 0
indicator.Parent = indicatorFrame
table.insert(indicatorList, indicator)
wait(2)
indicator:Destroy()
table.remove(indicatorList, 1)
end
local function onHealthChanged()
local healthDifference = humanoid.Health - previousHealth
if healthDifference < 0 then
print("Creating damage indicator:", math.abs(healthDifference))
createIndicator(math.abs(healthDifference), true)
elseif healthDifference > 0 then
if not cooldown then
print("Creating healing indicator:", healthDifference)
createIndicator(healthDifference, false)
cooldown = true
wait(cooldownTime)
cooldown = false
else
wait(cooldownTime)
end
end
previousHealth = humanoid.Health
end
humanoid.HealthChanged:Connect(onHealthChanged)
If you found this answer useful, do not forget to mark it as solved
.
@Katastro5 
1 Like
its problems are worse than the old one i want it to look like this when taking damage
try test ur script out in game, the damage value stacks.
1 Like
I am really sorry, buddy. I am doing my best right now to help you. Just to let you know, I am not a script expert, I am kind of a newbie on scripting. I rewrote your local script, and I think that’s what you want. I also added as many debug prints as possible to make it easier for you to fix it. Also, I can’t open Roblox Studio right now because on my current laptop I don’t have Roblox Studio installed, but I have it on my desktop (I am not at home).
local humanoid = character:WaitForChild("Humanoid")
local indicatorFrame = script.Parent
local previousHealth = humanoid.Health
local maxIndicators = 4
local indicatorList = {}
local cooldown = false
local cooldownTime = 2
local yOffset = 0
local function createIndicator(amount, isDamage)
local indicator = Instance.new("TextLabel")
indicator.Size = UDim2.new(0, 100, 0, 20)
indicator.Position = UDim2.new(0, 0, 0, yOffset)
indicator.BackgroundTransparency = 1
indicator.TextScaled = true
indicator.Text = (isDamage and "-" or "+") .. tostring(amount)
indicator.TextColor3 = isDamage and Color3.fromRGB(255, 0, 0) or Color3.fromRGB(0, 255, 0)
indicator.Font = Enum.Font.LuckiestGuy
indicator.TextStrokeTransparency = 0
indicator.Parent = indicatorFrame
table.insert(indicatorList, indicator)
print("Indicator created:", indicator.Text, "at yOffset:", yOffset)
wait(2)
indicator:Destroy()
table.remove(indicatorList, 1)
end
local function onHealthChanged()
for _, indicator in ipairs(indicatorList) do
indicator:Destroy()
end
indicatorList = {}
local healthDifference = humanoid.Health - previousHealth
print("Health difference:", healthDifference)
if healthDifference < 0 then
print("Creating damage indicator:", math.abs(healthDifference))
createIndicator(math.abs(healthDifference), true)
elseif healthDifference > 0 then
if not cooldown then
print("Creating healing indicator:", healthDifference)
createIndicator(healthDifference, false)
cooldown = true
print("Cooldown started")
wait(cooldownTime)
cooldown = false
print("Cooldown ended")
else
wait(cooldownTime)
print("Cooldown still active")
end
end
previousHealth = humanoid.Health
end
humanoid.HealthChanged:Connect(onHealthChanged)
1 Like
thanks dude i appreciate the support! ill look into the bugs.
You’re welcome, buddy. If you found my answer useful, please mark it as solved
.