The solution is really simple. You have to set the Y direction of the main (Base part of the switch) in the switch orientation.
Script:
-- Bool value
local isOn = true -- Bool
-- Light
local folder = script.Parent.Parent.Parent.Parent.Lights -- Lights folder
local light1 = folder.Light.SurfaceLight -- Lights
-- Switch Parts:
local trig = script.Parent.Parent.Parent.trig -- Trigger
local main = script.Parent.Parent.Parent.Main -- Main part
-- Direction
local dirS = nil -- Y direction
dirS = main.Orientation.Y -- Set the Y direction of the main
-- Functions
function on()
isOn = true
light1.Parent.Material = Enum.Material.Neon
light1.Enabled = true
script.Parent.Parent.Parent.trig.Orientation = Vector3.new(0, dirS, 10)
end
function off()
isOn = false
light1.Parent.Material = Enum.Material.Glass
light1.Enabled = false
script.Parent.Parent.Parent.trig.Orientation = Vector3.new(0, dirS, -10)
end
function onClicked()
-- Other things
if isOn == true then off() else on() end
end
script.Parent.Parent.ClickDetector.MouseClick:connect(onClicked)
off()
here is the switch in the explorer:
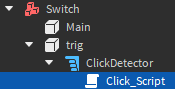
and the lights folder, with its parts:
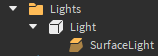
If you have multiple lights and the switch have to enable or disable all of them you need a for loop:
-- Bool value
local isOn = true -- Bool
-- Light
local folder = script.Parent.Parent.Parent.Parent.Lights -- Lights folder
local lights = folder:GetDescendants() -- Lights
-- Switch Parts:
local trig = script.Parent.Parent.Parent.trig -- Trigger
local main = script.Parent.Parent.Parent.Main -- Main part
-- Direction
local dirS = nil -- Y direction
dirS = main.Orientation.Y -- Set the Y direction of the main
-- Tween
local tween = game:GetService("TweenService") -- TweenService
-- Functions
function on()
isOn = true
for _,v in pairs(lights) do
--[[ If you have both Surface light and Spot light you can easily add:
if v:IsA("SurfaceLight") or v:IsA("SpotLight") then ]]--
if v:IsA("SurfaceLight") then -- Checks if the object is a "Surface Light", and if it is true then:
v.Parent.Material = Enum.Material.Neon -- Change material
v.Enabled = true -- Enable the light
end
end
script.Parent.Parent.Parent.trig.Orientation = Vector3.new(0, dirS, 10)
end
function off()
-- Same think that i did above:
isOn = false
for _,v in pairs(lights) do
if v:IsA("SurfaceLight") then
v.Parent.Material = Enum.Material.Glass
v.Enabled = false
end
end
script.Parent.Parent.Parent.trig.Orientation = Vector3.new(0, dirS, -10)
end
function onClicked()
-- Other things
if isOn == true then off() else on() end
end
-- Event
script.Parent.Parent.ClickDetector.MouseClick:connect(onClicked)
off()
I want to give you some advice on the aesthetics of switch. If you want to make more realistic the light switch, give it an animation:
-- Bool value
local isOn = true -- Bool
-- Light
local folder = script.Parent.Parent.Parent.Parent.Lights -- Lights folder
local lights = folder:GetDescendants() -- Lights
-- Switch Parts:
local trig = script.Parent.Parent.Parent.trig -- Trigger
local main = script.Parent.Parent.Parent.Main -- Main part
-- Direction
local dirS = nil -- Y direction
dirS = main.Orientation.Y -- Set the Y direction of the main
-- Tween
local tween = game:GetService("TweenService") -- TweenService
-- Functions
function on()
isOn = true
for _,v in pairs(lights) do
--[[ If you have both Surface light and Spot light you can easily add:
if v:IsA("SurfaceLight") or v:IsA("SpotLight") then ]]--
if v:IsA("SurfaceLight") then -- Checks if the object is a "Surface Light", and if it is true then:
v.Parent.Material = Enum.Material.Neon -- Change material
v.Enabled = true -- Enable the light
end
end
-- Create an animation for the trigger, you can customize it on what you like. I link to you the animation doc at the end of the reply.
tween:Create(trig, TweenInfo.new(0.2, Enum.EasingStyle.Quad, Enum.EasingDirection.InOut),{Orientation = Vector3.new(0, dirS, 10)}):Play()
end
function off()
-- Same think that i did above:
isOn = false
for _,v in pairs(lights) do
if v:IsA("SurfaceLight") then
v.Parent.Material = Enum.Material.Glass
v.Enabled = false
end
end
tween:Create(trig, TweenInfo.new(0.2, Enum.EasingStyle.Quad, Enum.EasingDirection.InOut),{Orientation = Vector3.new(0, dirS, -10)}):Play()
end
function onClicked()
-- Other things
if isOn == true then off() else on() end
end
-- Event
script.Parent.Parent.ClickDetector.MouseClick:connect(onClicked)
off()
And for the end you can add a sound when you click on the trigger:
I advise you to local only one sound, and not a sound per trigger, this because is more efficient and customizable.
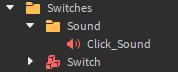
Here is the final script:
-- Bool value
local isOn = true -- Bool
-- Light
-- There i added a "Parent" to the local because we inserted the switch in a folder.
local folder = script.Parent.Parent.Parent.Parent.Parent.Lights -- Lights folder
local lights = folder:GetDescendants() -- Lights
-- Switch Parts:
local trig = script.Parent.Parent.Parent.trig -- Trigger
local main = script.Parent.Parent.Parent.Main -- Main part
-- Direction
local dirS = nil -- Y direction
dirS = main.Orientation.Y -- Set the Y direction of the main
-- Sound
local Click_Sound = main.Parent.Parent.Sound.Click_Sound -- The click sound
-- Tween
local tween = game:GetService("TweenService") -- TweenService
-- Functions
function on()
isOn = true
for _,v in pairs(lights) do
--[[ If you have both Surface light and Spot light you can easily add:
if v:IsA("SurfaceLight") or v:IsA("SpotLight") then ]]--
if v:IsA("SurfaceLight") then -- Checks if the object is a "Surface Light", and if it is true then:
v.Parent.Material = Enum.Material.Neon -- Change material
v.Enabled = true -- Enable the light
end
end
-- Create an animation for the trigger, you can customize it on what you like. I link to you the animation doc at the end of the reply.
tween:Create(trig, TweenInfo.new(0.2, Enum.EasingStyle.Quad, Enum.EasingDirection.InOut),{Orientation = Vector3.new(0, dirS, 10)}):Play()
end
function off()
-- Same think that i did above:
isOn = false
for _,v in pairs(lights) do
if v:IsA("SurfaceLight") then
v.Parent.Material = Enum.Material.Glass
v.Enabled = false
end
end
tween:Create(trig, TweenInfo.new(0.2, Enum.EasingStyle.Quad, Enum.EasingDirection.InOut),{Orientation = Vector3.new(0, dirS, -10)}):Play()
end
function onClicked()
-- Play click sound:
Click_Sound:Play()
if isOn == true then off() else on() end
end
-- Event
script.Parent.Parent.ClickDetector.MouseClick:connect(onClicked)
off()
Documentation:
I hope this helped!