Im making a gun. I wanna know how I can transfer the raycast results from client to server so I can find the parent of the part and make sure its a players character so I can then find humanoid. can someone help me?
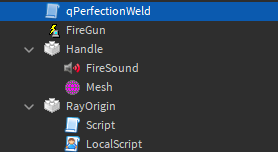
Im making a gun. I wanna know how I can transfer the raycast results from client to server so I can find the parent of the part and make sure its a players character so I can then find humanoid. can someone help me?
I reccomend you never allow the the client to send final results of a raycast as client scripts are heavily exploitable and will always tell the server that they hit no matter what, so you should allow the server to be raycasting.
If you want to send information then send the mouse.Hit.Position into the :FireServer()
as so:
script.Parent.Parent.FireGun:FireServer(mouse.Hit.Position)
because if the client sends a position over a wall it will still miss since the raycast hit a wall on the server
Is there a way I can send over Unitray instead of mouse hit position?
Mouse.Hit.Position keeps all the unitray/etc so the serverscript can access it through there. The reason why im sending just the Hit.Position is because i only want the most basic information from the client that is hard to mirror
Also try using
local MousePosition = --Your mouse position from remote event
local origin = -- your origin
local Direction = (MousePosition - origin).Unit
local ray = workspace:RayCast(origin,Direction, --your raycastparams)
origin is supposed to be script.Parent.Position
as your subtracting a vector3(mouse.hit.Position) - another vector3(script.Parent.Position) and then getting a normalized copy of it
I did .Position but Im still getting the same error
also in your raycastparams, you can have it only filter player.Character
raycastparams.FilterDescendantsInstances = {player.Character}
since the tool is also a descendant of the player character you can leave it like this
can i see your new code in a code box?
I try copy and pasting my code but only half of it goes into a code box how do I fix do that?
Select the entire script then put it inside a code box, is your ctrl c and ctrl v not working?
If not then try to send a screenshot of the code
this is whats happening
— local script
local Players = game:GetService(“Players”)
local player = Players.LocalPlayer
local mouse = player:GetMouse()
script.Parent.Parent.Activated:Connect(function()
local raycastParams = RaycastParams.new()
raycastParams.FilterDescendantsInstances = {player.Character}
raycastParams.FilterType = Enum.RaycastFilterType.Blacklist
local rayOrigin = script.Parent.Position
local raycastResult = workspace:Raycast(rayOrigin, mouse.UnitRay.Direction* 250 , raycastParams)
print(raycastResult)
script.Parent.Parent.Handle.FireSound:Play()
script.Parent.Parent.FireGun:FireServer(player, mouse.Hit.Position)
end)
—normal script
script.Parent.Parent.FireGun.OnServerEvent:Connect(function(player,mouse)
local MousePosition = mouse --Your mouse position from remote event
local origin = script.Parent.Position-- your origin
local Direction = (MousePosition - origin).Unit
local raycastParams = RaycastParams.new()
raycastParams.FilterDescendantsInstances = {script.Parent.Parent, player.Character}
raycastParams.FilterType = Enum.RaycastFilterType.Blacklist
local ray = workspace:RayCast(origin,Direction, raycastParams)
print("IS THIS")
end)
script.Parent.Parent.FireGun.OnServerEvent:Connect(function(player,mouse)
local MousePosition = mouse --Your mouse position from remote event
local origin = script.Parent.Position-- your origin
local Direction = (MousePosition - origin).Unit
local raycastParams = RaycastParams.new()
raycastParams.FilterDescendantsInstances = {script.Parent.Parent, player.Character}
raycastParams.FilterType = Enum.RaycastFilterType.Blacklist
local ray = workspace:RayCast(origin,Direction, raycastParams)
if ray then
print("IS THIS")
end
end)
Does it show any error? is it printing when the ray hits?
Print origin and print MousePosition, both of them need to be vector3 values
you need to print origin and MousePosition above the local Direction line
so do you want me to make it the first line in the function?