Hey there! This isn’t too hard to make, I think the best way to approach this by making an intvalue setting that intvalue equal to 3, and then every time the player died, negate one of that value, with it also checking if the value is equal to 0, change the player’s team.
You need to make a script into serverscriptservice, all the code will be written in a script in serverscriptservice.
First of all, let’s make the value, I’m not sure when you want the player to have this, but let’s assume you want the system whenever a player joins, so let’s create a simple player added function like this:
game.Players.PlayerAdded:Connect(function(player)
end)
This function will fire every time a player has joined the game, let’s now create the value.
game.Players.PlayerAdded:Connect(function(player)
local lives = Instance.new("IntValue") -- Creating the value
lives.Name = "Lives" -- Setting a name for the value
lives.Parent = player -- Parenting the intvalue to the player
end)
Great, we now made the value, let’s now set it to 3 using this line of code, I think it’s pretty obvious how I do it.
lives.Value = 3
Alright, now we made our value, let’s now check when a player has died, we can do this using the humanoid.Died function, a simple build-in function that checks when a player has died. We do have to make sure the player’s character has already spawned in though, we can do this using the character added function:
game.Players.PlayerAdded:Connect(function(player)
local lives = Instance.new("IntValue") -- Creating the value
lives.Name = "Lives" -- Setting a name for the value
lives.Value = 3
lives.Parent = player -- Parenting the intvalue to the player
player.CharacterAdded:Connect(function(character) -- Whenever the player's character has loaded in, this event will fire
end)
end)
Now we can actually use the humanoid.Died event, we will also make use of the function WaitForChild, this function will wait for the humanoid, so it doesn’t get loaded too quickly, and to prevent any errors.
local humanoid = character:WaitForChild("Humanoid") -- Using the waitforchild methode
humanoid.Died:Connect(function() -- When the player has died, this function will run
end)
Alright, now we can just remove 1 live from the player by saying live.Value -= 1
local humanoid = character:WaitForChild("Humanoid") -- Using the waitforchild methode
humanoid.Died:Connect(function() -- When the player has died, this function will run
lives.Value -= 1 -- Decrements one life of the player
end)
Almost there! We just have to make sure the player actually switches team, we can do it by using an if statement, we’ll say: if the player’s deaths are equal to 0, switch the player’s team.
local humanoid = character:WaitForChild("Humanoid")
humanoid.Died:Connect(function()
lives.Value -= 1
-- Make sure to put this AFTER we have removed the one live from the player, so it doesn't check it too early
if lives.Value == 0 then -- When using an if statement to check something, you use == instead of =, == is being used to check something and = is used to set something to something.
end
end)
Alright, now we would have to change the player’s team, we would have to do this by using teamcolors, teams use color’s for this, as you can see, every team should have a different color, so let’s assume we want to switch to the police team, just set the team color to white. You can do that like this:
humanoid.Died:Connect(function()
lives.Value -= 1
-- Make sure to put this AFTER we have removed the one live from the player, so it doesn't check it too early
if lives.Value == 0 then -- When using an if statement to check something, you use == instead of =, == is being used to check something and = is used to set something to something.
player.TeamColor = BrickColor.new("White") -- We have to use brickcolor, since that's what teams use.
end
end)
My team for instance
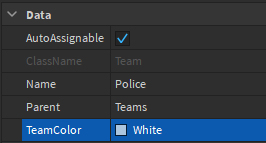
Make sure to change the brick color to your team color, also make sure you spelled your team color correctly, if you didn’t it won’t work.
Alright, this should actually be it, now, I am not sure if the player just get’s their life reset, if so, make sure to set the lives to 3 again.
lives.Value -= 1
-- Make sure to put this AFTER we have removed the one live from the player, so it doesn't check it too early
if lives.Value == 0 then -- When using an if statement to check something, you use == instead of =, == is being used to check something and = is used to set something to something.
player.TeamColor = BrickColor.new("White") -- We have to use brickcolor, since that's what teams use.
lives.Value = 3
end
If that’s not the case, please let me know, so I can help you with it.
Now, your script should look something like this:
game.Players.PlayerAdded:Connect(function(player)
local lives = Instance.new("IntValue")
lives.Name = "Lives"
lives.Value = 3
lives.Parent = player
player.CharacterAdded:Connect(function(character)
local humanoid = character:WaitForChild("Humanoid")
humanoid.Died:Connect(function()
lives.Value -= 1
if lives.Value == 0 then
player.TeamColor = BrickColor.new("White")
end
end)
end)
end)
I didn’t really have time to test this yet or you want anything changed, so if it doesn’t work, please let me know.
I hope this helped you and also helped you gain more understanding of lua.