Hi! I have a question about finding everything in an arbitrary layer of a matrix.
Let’s say I have an array that serves as a 2D matrix. It’s in the pattern of matrix[y][x]. For my first function, I want to write a for loop that gathers all the items at x = 1, which would be (1,any). For my second function I want the opposite; a for loop that gathers all items at y = 1, which would be (any,1). Only problem is that I don’t know how to do this. Here’s the general pattern I feel it should follow:
if (something) then
for _,part in pairs(Array[any][1]) do
(something)
end
else
for _,part in pairs(Array[1][any]) do
(something)
end
end
I’ve checked around for things like the %d pattern and have tried things like […] but I can’t seem to figure out what I should be using. It seems so intuitive yet I can’t figure out what I should be doing. My friend says the only way would be to create another array somehow, but that would be impractical since the array I’m going to be using is a 4D matrix. I’m hopeful that there’s an easy solution that I’m yet to discover but I also know that Lua can be frustratingly unintuitive at times so really hoping it isn’t gonna be some long esoteric process.
Any help would be greatly appreciated!
You are not using a numerical array like this? The answer is probably how you setup the array, I am confused.
for i=1, #Array[any] do
print(Array[i][1])
end
Wow, completely forgot that iterator feature of for loops even existed. Apologies, I’m a bit new to this still. However, I can’t seem to find a way to apply this to a 4D matrix. Here’s what I tried:
for i,j,k in #Array do
print(Array[i][1][j][k])
end
Any idea what I’m doing wrong here?
Oh and, for reference, the error I’m getting at line 1 of that would be “attempt to iterate over a number value”
This feels like it’s almost there, although I’m still getting strange errors. When I remove the k, I get a nil output, but when I add the k again, I get the error “attempt to index nil with nil.” What’s missing?
Can you send your array or how did you setup it?
An example:
array = {
x = {1,2,3},
y = {
y1 = 4,
y2 = 5,
y3 = 6
}
}
local Array = {}
for w = 1, value do
local ArraySub1 = {}
for z = 1, value do
local ArraySub2 = {}
for y = 1, value do
local ArraySub3 = {}
for x = 1, value do
local thing = Instance.New("thingy")
table.insert(ArraySub3,thing)
end
table.insert(ArraySub2,ArraySub3)
end
table.insert(ArraySub1,ArraySub2)
end
table.insert(Array,ArraySub1)
end
I’m aware that this is probably a horrible way to do something like this but like I said, sorta new to this.
i don’t think this is a 2d matrix
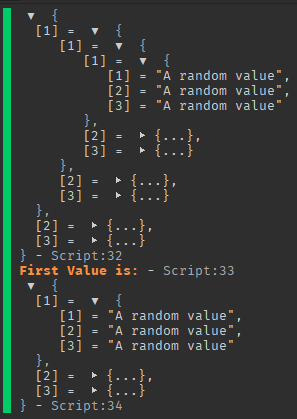
anyway i think you would need a recursive search function( maybe a 4D one? i don’t really know)
local mainMatrix = {}
local size = 2
function CreateTheMatrix()
for w = 1, size do
local ArraySub1 = {}
for z = 1, size do
local ArraySub2 = {}
for y = 1, size do
local ArraySub3 = {}
for x = 1, size do
local value = math.random(1,2)
table.insert(ArraySub3,value)
end
table.insert(ArraySub2,ArraySub3)
end
table.insert(ArraySub1,ArraySub2)
end
table.insert(mainMatrix,ArraySub1)
end
end
function CountXInMatrix(searchIn, valueToCount)
local count = 0
local matrix = table.clone(searchIn)
local function SearchInArray(array)
for index,value in array do
if typeof(value) == "table" then
SearchInArray(value)
elseif value == valueToCount then
count += 1
end
end
end
-- assumes the first value is always an array
for index,array in matrix do
SearchInArray(array)
end
return count
end
CreateTheMatrix()
print(mainMatrix)
print(CountXInMatrix(mainMatrix,1))
print(CountXInMatrix(mainMatrix,2))
print(CountXInMatrix(mainMatrix,3))
Thanks, I’ll give this a try next time I have a chance.
1 Like