Okay, so I’m making an SCP Containment breach type game, that includes a round system. The round system should pick from a table of 3 teams, and put a player on one of those 3 teams. The problem is, I don’t know how to do that with a normal script. Now, I could do it using a combination of local scripts + remote events, but I’m trying to keep it as simple as possible.
This is what I have so far:
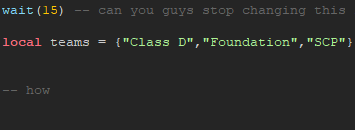
I have no idea what to do next
I’ve tried using free model scripts and fixing them so it works for my game but they don’t work.
If anybody can help me with this, I would really appreciate it
are the teams random or just like a team system?
Not really sure what you mean by this, but incase I understand you right the teams are already made
are the teams suppose to be randomized like after the round the player has a chance to go the other team
Yes, the teams are supposed to be randomized after each round
To choose a random team you would want to do it like this:
local random = Random.new()
local teams = {"Class D", "Foundation", "SCP"}
local teamSelected = random:NextInteger(1, #teams)
--// Team selected will then return one of those 3 teams so you can do what you need to after
in order to make them random you would have to make a random value for example
teams[“Class D”] = math.random
if you dont know what random does here is a article about it. Random | Documentation - Roblox Creator Hub
and then you would have to make a table for it
if you also dont know what a table does here is a article about it. Tables | Documentation - Roblox Creator Hub
i hope this helps!
im still learning random and tables and can only help a little
Thanks guys! I got it to work, its a little buggy right now but it shouldn’t be too had to fix.
You can do this in 3 steps:
1. Select a number of players for each team
You get the number of players in the server then generate a number of players for each team:
local PlayersToSpawn = game:GetService("Players"):GetPlayers() -- Get a table of players to spawn in game
local AmountOfPlayers = #PlayersToSpawn
local ClassDAmount = math.floor(0.4 * AmountOfPlayers) -- math.floor() will remove the decimal place from the number, ex. 1.6 --> 1
local FoundationAmount = ClassDAmount -- Spawn foundation as much as class D
local SCPAmount = AmountOfPlayers - ClassDAmount - FoundationAmount -- Set the SCP amount to the remaining players
2. Randomize players on each team
Do a for loop for each team, and pick a random player to spawn on a certain team.
local ClassD = {} -- Make a table of players to spawn as class D
for i = 1, ClassDAmount do
local SelectedPlayer = math.random(1, #PlayersToSpawn) -- Pick a random player
table.insert(ClassD, PlayersToSpawn[SelectedPlayer]) -- Insert the player in the table
table.remove(PlayersToSpawn, SelectedPlayer) -- Remove the player from the players table so you don't select him again for another team
end
Repeat the above for each team
3. Spawn the players
Go in each team table and spawn your players
for i,v in pairs(ClassD) do
-- spawn your player as a class-D
end
for i,v in pairs(Foundation) do
-- spawn your player as foundation
end
for i,v in pairs(SCPs) do
-- spawn your player as an SCP
end